使用Python在谷歌上爬数据有几种方法:使用Selenium自动化浏览器、使用BeautifulSoup解析HTML、使用Google Custom Search API、通过Scrapy框架进行爬取。 其中,使用Selenium自动化浏览器是最常见的方法,因为它能够模拟用户行为,绕过一些反爬虫机制。例如,Selenium可以打开浏览器,输入关键词,翻页,并抓取搜索结果。接下来我们将详细介绍如何使用Selenium来在谷歌上爬数据。
一、安装和配置环境
在开始之前,我们需要安装Selenium和WebDriver。Selenium是一个功能强大的工具,它可以控制浏览器进行各种操作,而WebDriver是Selenium用来与浏览器进行交互的驱动程序。为了使用Selenium,我们还需要一个支持的浏览器,例如Google Chrome,以及相应的ChromeDriver。
pip install selenium
安装好Selenium后,我们需要下载适合自己Chrome版本的ChromeDriver,并将其路径配置到系统环境变量中。你可以从ChromeDriver官方网站下载适合你版本的ChromeDriver。
二、使用Selenium打开谷歌并搜索关键词
首先,我们需要导入Selenium库,并设置ChromeDriver路径。然后,我们可以使用Selenium打开谷歌主页,并输入搜索关键词。
from selenium import webdriver
from selenium.webdriver.common.keys import Keys
import time
设置ChromeDriver路径
driver_path = '/path/to/chromedriver'
driver = webdriver.Chrome(driver_path)
打开谷歌主页
driver.get("http://www.google.com")
找到搜索输入框
search_box = driver.find_element_by_name("q")
输入搜索关键词并回车
search_box.send_keys("Python web scraping")
search_box.send_keys(Keys.RETURN)
等待页面加载
time.sleep(3)
三、解析搜索结果
在搜索结果页面加载完成后,我们需要解析页面内容,提取我们需要的数据。我们可以使用Selenium的find_elements_by_xpath
方法来定位搜索结果。
# 获取所有搜索结果
results = driver.find_elements_by_xpath('//div[@class="g"]')
遍历搜索结果
for result in results:
# 获取结果标题
title = result.find_element_by_xpath('.//h3').text
# 获取结果链接
link = result.find_element_by_xpath('.//a').get_attribute('href')
# 获取结果描述
description = result.find_element_by_xpath('.//span[@class="st"]').text
print(f'Title: {title}')
print(f'Link: {link}')
print(f'Description: {description}')
print('-' * 80)
四、处理分页
有时候,我们需要获取多个页面的搜索结果。在这种情况下,我们需要处理分页。我们可以使用Selenium点击“下一页”按钮,然后重复上述步骤抓取数据。
# 获取下一页按钮
next_button = driver.find_element_by_xpath('//a[@id="pnnext"]')
点击下一页按钮
next_button.click()
等待页面加载
time.sleep(3)
重新获取搜索结果
results = driver.find_elements_by_xpath('//div[@class="g"]')
遍历搜索结果
for result in results:
# 获取结果标题
title = result.find_element_by_xpath('.//h3').text
# 获取结果链接
link = result.find_element_by_xpath('.//a').get_attribute('href')
# 获取结果描述
description = result.find_element_by_xpath('.//span[@class="st"]').text
print(f'Title: {title}')
print(f'Link: {link}')
print(f'Description: {description}')
print('-' * 80)
五、使用BeautifulSoup解析HTML
有时候,单独使用Selenium可能不够高效。我们可以结合BeautifulSoup来解析HTML内容,这样可以更方便地提取数据。
from bs4 import BeautifulSoup
获取页面HTML内容
html = driver.page_source
解析HTML内容
soup = BeautifulSoup(html, 'html.parser')
获取所有搜索结果
results = soup.find_all('div', class_='g')
遍历搜索结果
for result in results:
# 获取结果标题
title = result.find('h3').text
# 获取结果链接
link = result.find('a')['href']
# 获取结果描述
description = result.find('span', class_='st').text
print(f'Title: {title}')
print(f'Link: {link}')
print(f'Description: {description}')
print('-' * 80)
六、使用Google Custom Search API
如果你不想使用Selenium模拟浏览器行为,可以考虑使用Google Custom Search API。你需要先创建一个自定义搜索引擎,并获取API密钥。
import requests
设置API密钥和搜索引擎ID
api_key = 'YOUR_API_KEY'
search_engine_id = 'YOUR_SEARCH_ENGINE_ID'
设置搜索关键词
query = 'Python web scraping'
构建API请求URL
url = f'https://www.googleapis.com/customsearch/v1?q={query}&key={api_key}&cx={search_engine_id}'
发送API请求
response = requests.get(url)
解析API响应
data = response.json()
遍历搜索结果
for item in data['items']:
# 获取结果标题
title = item['title']
# 获取结果链接
link = item['link']
# 获取结果描述
description = item['snippet']
print(f'Title: {title}')
print(f'Link: {link}')
print(f'Description: {description}')
print('-' * 80)
七、使用Scrapy框架
Scrapy是一个强大的爬虫框架,它可以高效地抓取和解析网页数据。使用Scrapy,你可以更灵活地处理大规模爬取需求。
import scrapy
class GoogleSpider(scrapy.Spider):
name = "google_spider"
allowed_domains = ["google.com"]
start_urls = ['https://www.google.com/search?q=Python+web+scraping']
def parse(self, response):
# 获取所有搜索结果
results = response.xpath('//div[@class="g"]')
for result in results:
# 获取结果标题
title = result.xpath('.//h3/text()').get()
# 获取结果链接
link = result.xpath('.//a/@href').get()
# 获取结果描述
description = result.xpath('.//span[@class="st"]/text()').get()
yield {
'title': title,
'link': link,
'description': description
}
# 获取下一页链接
next_page = response.xpath('//a[@id="pnnext"]/@href').get()
if next_page:
yield scrapy.Request(response.urljoin(next_page), callback=self.parse)
八、处理反爬虫机制
在爬取谷歌数据时,你可能会遇到一些反爬虫机制,例如CAPTCHA验证。为了绕过这些机制,你可以考虑以下几种方法:
- 增加请求间隔:在每次请求之间增加随机的等待时间,以模拟人类行为。
- 使用代理:通过代理服务器发送请求,以避免IP被封禁。
- 使用用户代理:在请求头中设置不同的用户代理,以模拟不同的浏览器和设备。
from selenium.webdriver.common.by import By
from selenium.webdriver.chrome.options import Options
import random
设置Chrome选项
chrome_options = Options()
chrome_options.add_argument("--headless")
chrome_options.add_argument("--disable-gpu")
chrome_options.add_argument("user-agent=Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/91.0.4472.124 Safari/537.36")
设置代理服务器
chrome_options.add_argument('--proxy-server=http://your-proxy-server:port')
设置ChromeDriver路径
driver = webdriver.Chrome(driver_path, options=chrome_options)
打开谷歌主页
driver.get("http://www.google.com")
找到搜索输入框
search_box = driver.find_element(By.NAME, "q")
输入搜索关键词并回车
search_box.send_keys("Python web scraping")
search_box.send_keys(Keys.RETURN)
等待页面加载
time.sleep(random.randint(2, 5))
获取所有搜索结果
results = driver.find_elements(By.XPATH, '//div[@class="g"]')
遍历搜索结果
for result in results:
# 获取结果标题
title = result.find_element(By.XPATH, './/h3').text
# 获取结果链接
link = result.find_element(By.XPATH, './/a').get_attribute('href')
# 获取结果描述
description = result.find_element(By.XPATH, './/span[@class="st"]').text
print(f'Title: {title}')
print(f'Link: {link}')
print(f'Description: {description}')
print('-' * 80)
九、数据存储和处理
在爬取数据后,我们通常需要将数据存储到文件或数据库中,以便后续处理。我们可以使用Python的pandas库将数据存储到CSV文件中,或者使用SQLite数据库进行存储。
使用pandas存储数据到CSV文件
import pandas as pd
创建一个空的DataFrame
df = pd.DataFrame(columns=['Title', 'Link', 'Description'])
遍历搜索结果
for result in results:
# 获取结果标题
title = result.find_element(By.XPATH, './/h3').text
# 获取结果链接
link = result.find_element(By.XPATH, './/a').get_attribute('href')
# 获取结果描述
description = result.find_element(By.XPATH, './/span[@class="st"]').text
# 添加数据到DataFrame
df = df.append({'Title': title, 'Link': link, 'Description': description}, ignore_index=True)
将DataFrame存储到CSV文件
df.to_csv('google_search_results.csv', index=False)
使用SQLite数据库存储数据
import sqlite3
连接到SQLite数据库(如果数据库不存在,则会创建一个新的数据库)
conn = sqlite3.connect('google_search_results.db')
创建一个Cursor对象
cur = conn.cursor()
创建一个表
cur.execute('''
CREATE TABLE IF NOT EXISTS search_results (
id INTEGER PRIMARY KEY,
title TEXT,
link TEXT,
description TEXT
)
''')
遍历搜索结果
for result in results:
# 获取结果标题
title = result.find_element(By.XPATH, './/h3').text
# 获取结果链接
link = result.find_element(By.XPATH, './/a').get_attribute('href')
# 获取结果描述
description = result.find_element(By.XPATH, './/span[@class="st"]').text
# 插入数据到表中
cur.execute('''
INSERT INTO search_results (title, link, description)
VALUES (?, ?, ?)
''', (title, link, description))
提交事务
conn.commit()
关闭连接
conn.close()
十、总结
使用Python在谷歌上爬数据的方法多种多样,每种方法都有其优缺点。Selenium是一个功能强大的工具,它可以模拟用户行为,绕过一些反爬虫机制;BeautifulSoup可以高效地解析HTML内容;Google Custom Search API可以直接获取搜索结果,而不需要模拟浏览器行为;Scrapy是一个高效的爬虫框架,适合大规模爬取需求。在实际应用中,你可以根据具体需求选择合适的方法,并结合多种技术手段,处理反爬虫机制,确保数据爬取的稳定性和高效性。最后,将爬取的数据存储到文件或数据库中,便于后续处理和分析。
相关问答FAQs:
如何使用Python进行网页爬取的基本步骤是什么?
在使用Python进行网页爬取时,首先需要安装一些常用的库,比如Requests和BeautifulSoup。Requests库可以帮助你发送HTTP请求,获取网页内容,而BeautifulSoup则用于解析HTML文档。接着,编写代码来请求特定的URL,获取页面的HTML源代码,并利用BeautifulSoup提取你需要的数据,比如标题、链接或其他信息。最后,处理和存储抓取到的数据,可能会选择将其保存到CSV文件或数据库中。
在爬取谷歌搜索结果时,有哪些需要注意的事项?
当爬取谷歌搜索结果时,需要遵循谷歌的使用条款,避免过于频繁地发送请求,以免被视为恶意行为而被封禁IP。使用代理IP和适当的请求间隔可以帮助减少被封的风险。此外,谷歌的搜索结果页面是动态生成的,可能需要使用Selenium等工具模拟浏览器操作来获取数据。确保遵循robots.txt文件中的规则,了解哪些页面允许爬取。
如何处理抓取到的数据以便进行分析?
抓取到的数据通常是以文本形式存在,首先需要对其进行清洗,去除多余的空格、特殊字符及HTML标签等。可以使用Pandas库将数据加载到DataFrame中,便于进一步分析。接下来,可以使用Python的数据分析库,如NumPy和Matplotlib,对数据进行统计和可视化分析,帮助你从抓取的数据中提取有价值的信息。
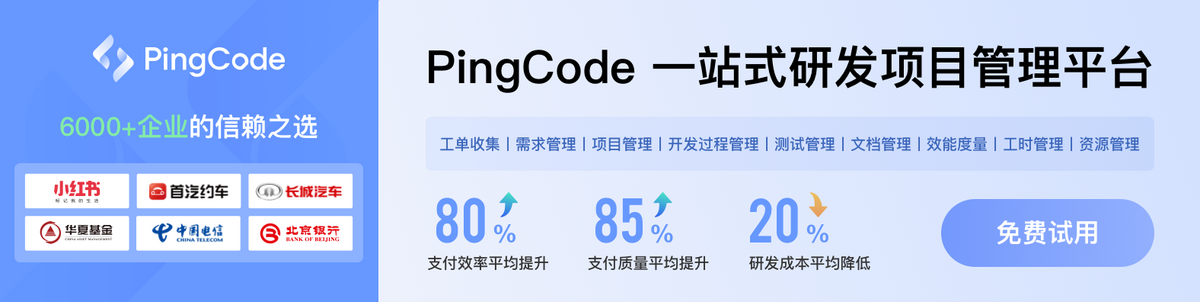