在Python中定义和调用函数的核心要点包括:使用def关键字定义函数、函数内可以调用其他函数、函数可以返回值、参数可以传递给函数。下面我们将详细解释这些要点,并展示一些示例代码。
一、定义函数和调用函数
在Python中,定义函数使用def
关键字。一个函数可以接受参数,并可以返回值。函数定义后,可以通过其名称来调用它。
def greet(name):
return f"Hello, {name}!"
在上面的例子中,我们定义了一个名为greet
的函数,它接受一个参数name
,并返回一个问候字符串。我们可以通过以下方式调用它:
message = greet("Alice")
print(message) # 输出: Hello, Alice!
二、函数内部调用其他函数
一个函数内部可以调用另一个函数。这使得代码更模块化和可重用。
def add(a, b):
return a + b
def multiply(a, b):
return a * b
def combined_operations(x, y):
sum_result = add(x, y)
product_result = multiply(x, y)
return sum_result, product_result
在这个例子中,combined_operations
函数内部调用了add
和multiply
函数。
sum_result, product_result = combined_operations(3, 4)
print(f"Sum: {sum_result}, Product: {product_result}")
三、带有默认参数值的函数
在Python中,函数参数可以有默认值。如果调用函数时未提供参数,则使用默认值。
def greet(name="World"):
return f"Hello, {name}!"
message = greet()
print(message) # 输出: Hello, World!
四、使用可变数量的参数
Python允许在函数定义中使用*args
和kwargs
来处理可变数量的参数。
def add(*args):
return sum(args)
result = add(1, 2, 3, 4)
print(result) # 输出: 10
在上面的例子中,*args
允许我们传递任意数量的参数给add
函数。
def display_info(kwargs):
for key, value in kwargs.items():
print(f"{key}: {value}")
display_info(name="Alice", age=30, city="New York")
kwargs
允许我们传递任意数量的关键字参数给display_info
函数。
五、嵌套函数
在Python中,函数可以嵌套在另一个函数内部。这种嵌套函数仅在其外部函数内有效。
def outer_function(text):
def inner_function():
print(text)
inner_function()
outer_function("Hello from the inner function!")
在这个例子中,inner_function
仅在outer_function
内有效,并打印了传递给outer_function
的text
参数。
六、返回函数
Python中的函数可以返回另一个函数。这种技术称为闭包。
def outer_function(text):
def inner_function():
return f"Message: {text}"
return inner_function
my_function = outer_function("Hello from the closure!")
print(my_function()) # 输出: Message: Hello from the closure!
在这个例子中,outer_function
返回了inner_function
,而inner_function
可以访问outer_function
的text
参数。
七、匿名函数
Python还支持匿名函数,使用lambda
关键字。匿名函数没有名称,通常用于简短的函数定义。
add = lambda a, b: a + b
result = add(2, 3)
print(result) # 输出: 5
在这个例子中,我们使用lambda
定义了一个匿名函数,并将其赋值给变量add
。
八、装饰器
装饰器是Python中的一种特殊函数,用于在不修改原函数代码的情况下,增加函数的功能。
def my_decorator(func):
def wrapper():
print("Something is happening before the function is called.")
func()
print("Something is happening after the function is called.")
return wrapper
@my_decorator
def say_hello():
print("Hello!")
say_hello()
在这个例子中,my_decorator
是一个装饰器,它在say_hello
函数前后打印消息。我们使用@my_decorator
语法将装饰器应用于say_hello
函数。
九、递归函数
递归函数是指在其定义中调用自身的函数。递归函数必须有一个基准条件,以避免无限递归。
def factorial(n):
if n == 1:
return 1
else:
return n * factorial(n - 1)
result = factorial(5)
print(result) # 输出: 120
在这个例子中,factorial
函数使用递归来计算一个数的阶乘。
十、生成器函数
生成器函数使用yield
关键字返回一个值,并记住其状态,以便在下次调用时继续执行。
def countdown(n):
while n > 0:
yield n
n -= 1
for number in countdown(5):
print(number)
在这个例子中,countdown
函数是一个生成器,每次调用时返回一个新的值,直到计数到零。
总结
在Python中定义和调用函数是编程的基本技能。通过使用函数,可以使代码更加模块化、可重用和易于维护。我们介绍了如何定义基本函数、嵌套函数、使用默认参数值、处理可变数量的参数、返回函数、使用匿名函数、装饰器、递归函数和生成器函数。这些技巧和概念将帮助你在Python编程中更加高效地使用函数。
相关问答FAQs:
在Python中,如何定义一个函数并在其中调用另一个函数?
在Python中,可以通过在一个函数的定义中直接调用另一个已定义的函数来实现函数的嵌套调用。首先,定义一个简单的函数,然后在另一个函数内部调用它。例如,您可以定义一个计算平方的函数,并在另一个函数中调用它来计算某个数的平方。以下是一个示例代码:
def square(x):
return x * x
def calculate_square_of_sum(a, b):
return square(a + b)
result = calculate_square_of_sum(3, 4)
print(result) # 输出: 49
在这个例子中,square
函数被calculate_square_of_sum
函数调用。
在函数内部调用自身是否可行?
是的,函数内部可以调用自身,这种技术称为递归。递归允许函数以简洁的方式解决问题。确保在递归过程中有一个明确的终止条件,以避免无限循环。例如,计算阶乘的函数可以使用递归方式:
def factorial(n):
if n == 0:
return 1
else:
return n * factorial(n - 1)
result = factorial(5)
print(result) # 输出: 120
如何处理函数参数并在调用时传递值?
在定义函数时,可以指定参数以接收外部传入的值。在函数调用时,您可以将具体的值传递给这些参数。例如:
def greet(name):
return f"Hello, {name}!"
def welcome_user(user_name):
return greet(user_name)
message = welcome_user("Alice")
print(message) # 输出: Hello, Alice!
在这个例子中,greet
函数接受一个参数name
,而welcome_user
函数则将用户名称传递给greet
函数。
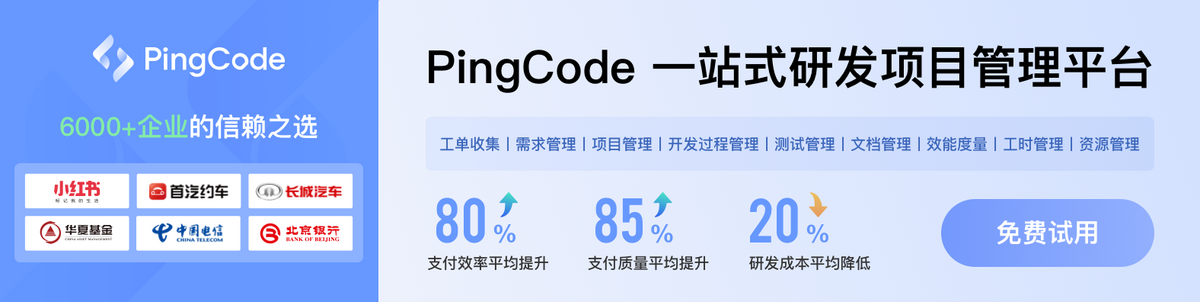