在Python的类中使用print方法有很多方法,例如,通过在类方法中使用print、在__str__或__repr__方法中定义print、使用__init__方法以及使用@property方法。 其中,最常用的方法是在类的方法中直接使用print语句,来输出对象的属性或其他信息。这种方法简单直接,适合大多数场景。
在Python中,类是一种面向对象编程的基本概念,使用类可以创建自定义数据类型。通过在类中定义方法,可以对对象进行操作。下面将详细介绍如何在Python的类中使用print方法。
一、在类方法中使用print
在类的方法中使用print是最常见的方式。通过这种方式,我们可以输出对象的属性或其他信息。
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def display_info(self):
print(f"Name: {self.name}, Age: {self.age}")
创建对象
person = Person("John", 25)
person.display_info() # 输出:Name: John, Age: 25
在上面的例子中,我们在display_info
方法中使用了print函数,来输出对象的name
和age
属性。
二、在__str__和__repr__方法中使用print
__str__
和__repr__
方法是Python中用于定义对象的字符串表示的方法。__str__
方法用于为用户提供友好的对象描述,而__repr__
方法则用于开发者调试时提供的对象描述。
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def __str__(self):
return f"Name: {self.name}, Age: {self.age}"
def __repr__(self):
return f"Person(name={self.name}, age={self.age})"
创建对象
person = Person("John", 25)
print(person) # 输出:Name: John, Age: 25
print(repr(person)) # 输出:Person(name=John, age=25)
在上面的例子中,我们定义了__str__
和__repr__
方法,并在其中使用了print函数。这样,当我们打印对象时,会输出我们定义的字符串表示。
三、在__init__方法中使用print
__init__
方法是Python类的构造方法,用于初始化对象的属性。在__init__
方法中使用print,可以输出对象的初始化信息。
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
print(f"Creating Person: Name: {self.name}, Age: {self.age}")
创建对象
person = Person("John", 25) # 输出:Creating Person: Name: John, Age: 25
在上面的例子中,我们在__init__
方法中使用了print函数,来输出对象的初始化信息。
四、使用@property方法
@property方法用于定义属性的getter方法。我们可以在getter方法中使用print函数,来输出属性的值。
class Person:
def __init__(self, name, age):
self._name = name
self._age = age
@property
def name(self):
print(f"Getting name: {self._name}")
return self._name
@property
def age(self):
print(f"Getting age: {self._age}")
return self._age
创建对象
person = Person("John", 25)
print(person.name) # 输出:Getting name: John
print(person.age) # 输出:Getting age: 25
在上面的例子中,我们使用@property方法定义了name
和age
属性的getter方法,并在getter方法中使用了print函数。
五、在类的静态方法和类方法中使用print
除了实例方法外,我们还可以在类的静态方法和类方法中使用print。静态方法使用@staticmethod装饰器,类方法使用@classmethod装饰器。
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
@staticmethod
def static_method():
print("This is a static method")
@classmethod
def class_method(cls):
print("This is a class method")
调用静态方法和类方法
Person.static_method() # 输出:This is a static method
Person.class_method() # 输出:This is a class method
在上面的例子中,我们定义了静态方法static_method
和类方法class_method
,并在其中使用了print函数。
六、在异常处理块中使用print
在类的方法中,我们可以使用异常处理块来捕获异常,并在异常处理块中使用print函数来输出异常信息。
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def display_info(self):
try:
print(f"Name: {self.name}, Age: {self.age}")
except Exception as e:
print(f"An error occurred: {e}")
创建对象
person = Person("John", 25)
person.display_info() # 输出:Name: John, Age: 25
在上面的例子中,我们在display_info
方法中使用了异常处理块,并在except
块中使用了print函数来输出异常信息。
七、使用logging模块替代print
虽然print函数在调试时非常方便,但在实际开发中,我们通常使用logging模块来记录日志。logging模块提供了更强大的日志记录功能,可以灵活地控制日志的输出格式和输出位置。
import logging
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
logging.basicConfig(level=logging.INFO)
logging.info(f"Creating Person: Name: {self.name}, Age: {self.age}")
def display_info(self):
logging.info(f"Name: {self.name}, Age: {self.age}")
创建对象
person = Person("John", 25)
person.display_info() # 输出日志信息
在上面的例子中,我们使用logging模块记录日志信息。logging模块提供了比print函数更强大的功能,可以更方便地进行日志管理。
总结
通过以上介绍,我们了解了在Python的类中使用print方法的多种方式,包括在类方法中使用print、在__str__和__repr__方法中使用print、在__init__方法中使用print、使用@property方法、在静态方法和类方法中使用print、在异常处理块中使用print,以及使用logging模块替代print。每种方法都有其适用的场景,选择合适的方法可以使我们的代码更清晰、更易读。
在实际开发中,尽量减少直接使用print函数,建议使用logging模块进行日志记录,以便更好地管理和控制日志输出。
相关问答FAQs:
如何在Python类中重写print功能?
在Python中,可以通过重写__str__
和__repr__
方法来自定义类的打印输出。__str__
方法用于定义用户友好的字符串表示,而__repr__
方法则用于开发者的可读性。在类中实现这些方法后,可以直接使用print()
函数打印对象,输出将会根据这些方法定义的格式进行显示。
在Python类中使用print时,如何格式化输出?
可以通过定义类的属性以及在__str__
或__repr__
方法中使用格式化字符串来实现输出格式的自定义。例如,使用f-string或format()
方法,可以灵活地控制输出内容和样式,这样在调用print()
时,输出将会更加直观和易于理解。
如果我的类中有多个属性,怎样确保它们都被打印出来?
为了确保所有属性都被打印,可以在__str__
或__repr__
方法中显式列出每个属性。例如,可以使用一个循环遍历类的__dict__
属性来动态生成输出内容,或者手动将每个属性添加到返回的字符串中,这样在使用print()
时,所有相关信息都将被一并显示。
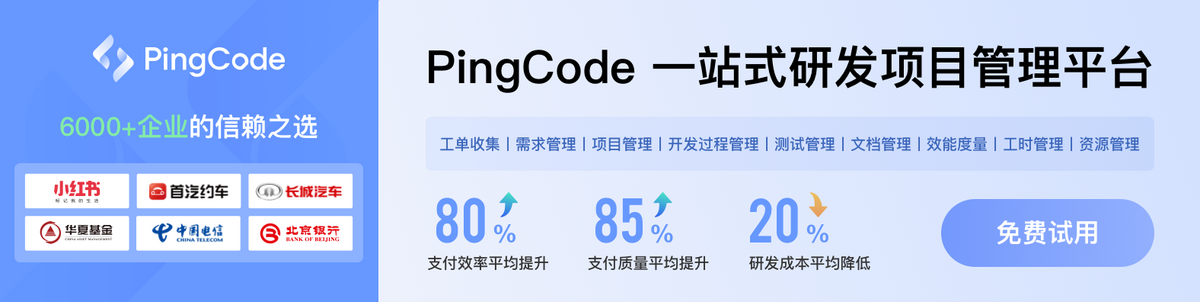