要用Python编写俄罗斯方块,可以使用Pygame库、定义基本游戏逻辑、创建图形界面、实现方块的移动和旋转。 下面我将详细介绍如何用Python编写一个简单的俄罗斯方块游戏。
一、安装Pygame库
为了开发俄罗斯方块游戏,我们首先需要安装Pygame库。Pygame是一个开源的Python库,用于编写视频游戏。安装Pygame的方法如下:
pip install pygame
二、游戏窗口初始化
首先,我们需要创建一个游戏窗口,并设置游戏的基本参数,例如窗口大小、标题等。
import pygame
import random
初始化Pygame
pygame.init()
设置窗口大小
screen_width = 300
screen_height = 600
screen = pygame.display.set_mode((screen_width, screen_height))
设置游戏标题
pygame.display.set_caption("俄罗斯方块")
定义颜色
colors = [
(0, 0, 0), # 背景颜色
(255, 0, 0), # 红色
(0, 255, 0), # 绿色
(0, 0, 255), # 蓝色
(255, 255, 0), # 黄色
(255, 0, 255), # 紫色
(0, 255, 255), # 青色
(255, 165, 0) # 橙色
]
三、定义方块形状
俄罗斯方块由不同形状的方块组成,这些形状可以用矩阵表示。我们需要定义这些形状。
# 定义方块形状
shapes = [
[[1, 1, 1, 1]],
[[1, 1], [1, 1]],
[[0, 1, 0], [1, 1, 1]],
[[1, 0, 0], [1, 1, 1]],
[[0, 0, 1], [1, 1, 1]],
[[1, 1, 0], [0, 1, 1]],
[[0, 1, 1], [1, 1, 0]]
]
四、定义方块类
为了管理方块的移动和旋转,我们可以定义一个方块类。
class Block:
def __init__(self, x, y, shape):
self.x = x
self.y = y
self.shape = shape
self.color = random.randint(1, len(colors) - 1)
self.rotation = 0
def rotate(self):
self.rotation = (self.rotation + 1) % len(self.shape)
五、游戏逻辑
我们需要定义游戏的主要逻辑,包括方块的移动、旋转、碰撞检测、消除完整行等。
def create_grid(locked_positions={}):
grid = [[(0, 0, 0) for _ in range(10)] for _ in range(20)]
for y in range(len(grid)):
for x in range(len(grid[y])):
if (x, y) in locked_positions:
grid[y][x] = locked_positions[(x, y)]
return grid
def draw_grid(surface, grid):
for y in range(len(grid)):
for x in range(len(grid[y])):
pygame.draw.rect(surface, grid[y][x], (x * 30, y * 30, 30, 30), 0)
def valid_space(shape, grid):
accepted_positions = [[(x, y) for x in range(10) if grid[y][x] == (0, 0, 0)] for y in range(20)]
accepted_positions = [x for sub in accepted_positions for x in sub]
formatted = convert_shape_format(shape)
for pos in formatted:
if pos not in accepted_positions:
if pos[1] > -1:
return False
return True
def convert_shape_format(shape):
positions = []
format = shape.shape[shape.rotation % len(shape.shape)]
for i, line in enumerate(format):
row = list(line)
for j, column in enumerate(row):
if column == '0':
positions.append((shape.x + j, shape.y + i))
for i, pos in enumerate(positions):
positions[i] = (pos[0] - 2, pos[1] - 4)
return positions
def check_lost(positions):
for pos in positions:
x, y = pos
if y < 1:
return True
return False
def get_shape():
return Block(5, 0, random.choice(shapes))
def draw_window(surface):
surface.fill((0, 0, 0))
draw_grid(surface, grid)
pygame.display.update()
六、主游戏循环
主游戏循环控制游戏的运行,包括处理用户输入、更新游戏状态、绘制游戏画面等。
def main():
locked_positions = {}
grid = create_grid(locked_positions)
change_piece = False
run = True
current_piece = get_shape()
next_piece = get_shape()
clock = pygame.time.Clock()
fall_time = 0
while run:
grid = create_grid(locked_positions)
fall_speed = 0.27
for event in pygame.event.get():
if event.type == pygame.QUIT:
run = False
pygame.display.quit()
quit()
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT:
current_piece.x -= 1
if not valid_space(current_piece, grid):
current_piece.x += 1
if event.key == pygame.K_RIGHT:
current_piece.x += 1
if not valid_space(current_piece, grid):
current_piece.x -= 1
if event.key == pygame.K_DOWN:
current_piece.y += 1
if not valid_space(current_piece, grid):
current_piece.y -= 1
if event.key == pygame.K_UP:
current_piece.rotate()
if not valid_space(current_piece, grid):
current_piece.rotate()
shape_pos = convert_shape_format(current_piece)
for i in range(len(shape_pos)):
x, y = shape_pos[i]
if y > -1:
grid[y][x] = colors[current_piece.color]
if change_piece:
for pos in shape_pos:
p = (pos[0], pos[1])
locked_positions[p] = colors[current_piece.color]
current_piece = next_piece
next_piece = get_shape()
change_piece = False
if check_lost(locked_positions):
run = False
draw_window(screen)
pygame.display.update()
if fall_time / 1000 >= fall_speed:
fall_time = 0
current_piece.y += 1
if not valid_space(current_piece, grid) and current_piece.y > 0:
current_piece.y -= 1
change_piece = True
clock.tick(60)
main()
七、总结
在这篇文章中,我们详细介绍了如何使用Python和Pygame库编写俄罗斯方块游戏。主要步骤包括安装Pygame库、初始化游戏窗口、定义方块形状和类、实现游戏逻辑、编写主游戏循环。 希望这篇文章能帮助你更好地理解Python游戏开发的基本原理,并激发你进一步探索和学习的兴趣。
相关问答FAQs:
如何用Python实现俄罗斯方块游戏的基本功能?
要实现俄罗斯方块的基本功能,您需要构建游戏的主要组件,包括游戏板、方块的生成和移动、碰撞检测以及消除行的逻辑。可以使用Pygame库,它提供了简单的图形和输入处理功能。您需要创建一个主循环来处理游戏逻辑和渲染方块,还要定义方块的形状和颜色。
使用Python编写俄罗斯方块时,有哪些常见的错误需要避免?
常见错误包括坐标计算错误、碰撞检测不准确、方块旋转时的逻辑问题、行消除算法的实现不当等。确保您的碰撞检测算法能够正确识别方块与边界和其他方块的碰撞,并且在消除行时更新游戏状态,避免出现游戏卡顿或逻辑错误。
如何提高用Python编写的俄罗斯方块游戏的可玩性?
可以通过添加不同的难度级别、增加方块的多样性、设置计时器、增加音效和背景音乐等方式来提高游戏的可玩性。此外,您还可以考虑增加分数系统、排行榜和多人对战模式等功能,以增强用户体验和互动性。
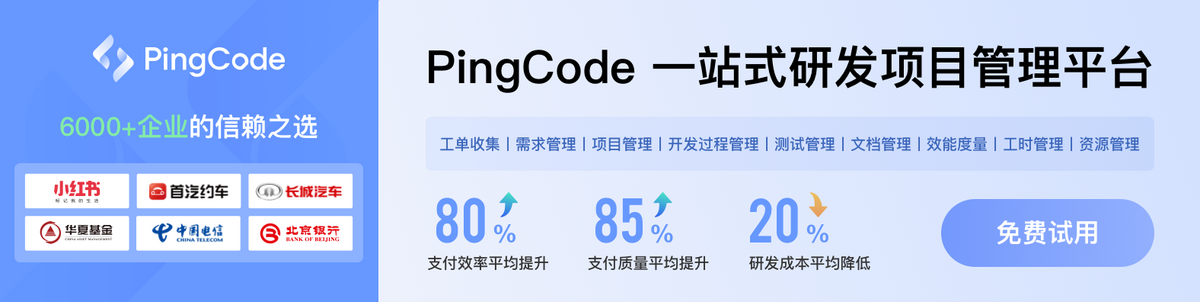