Python程序进行货币转换的方法有多种,可以通过调用API获取实时汇率、使用固定汇率、利用Python库等方式实现。调用API获取实时汇率、使用固定汇率、利用Python库。其中,调用API获取实时汇率是最常用的一种方式,因为它可以确保汇率的实时性和准确性。
调用API获取实时汇率可以确保汇率的实时性和准确性。例如,可以使用ExchangeRate-API、CurrencyLayer等在线服务来获取最新的汇率数据。通过发送HTTP请求到API端点,并解析返回的JSON数据,就可以获得不同货币之间的汇率,并用于转换计算。
一、调用API获取实时汇率
- 选择API服务
要进行货币转换,首先需要选择一个提供实时汇率数据的API服务。常见的API服务包括ExchangeRate-API、CurrencyLayer、Open Exchange Rates等。每个API服务都有自己的特点和使用方法,可以根据需求选择适合的服务。
ExchangeRate-API是一个流行的汇率数据提供商,它提供免费的和付费的API服务。免费版的API可以满足基本的汇率转换需求,而付费版则提供更多的功能和更高的请求频率。
- 获取API密钥
注册API服务后,需要获取API密钥(API Key),这是访问API的凭证。API密钥通常是一个长字符串,需要在发送请求时包含在请求参数中。
- 发送HTTP请求
使用Python的requests库可以方便地发送HTTP请求。以下是一个示例代码,展示如何使用ExchangeRate-API获取最新的汇率数据:
import requests
api_key = "your_api_key"
base_currency = "USD"
target_currency = "EUR"
url = f"https://v6.exchangerate-api.com/v6/{api_key}/latest/{base_currency}"
response = requests.get(url)
data = response.json()
if data["result"] == "success":
exchange_rate = data["conversion_rates"][target_currency]
print(f"Exchange rate from {base_currency} to {target_currency}: {exchange_rate}")
else:
print("Error fetching exchange rate data")
在这个示例中,api_key
是你的API密钥,base_currency
是基准货币,target_currency
是目标货币。请求URL包含了API密钥和基准货币。请求成功后,返回的数据是一个JSON对象,其中包含了所有目标货币的汇率。我们可以从中提取所需的汇率。
- 进行货币转换
有了汇率数据后,就可以进行货币转换了。假设我们要将100美元转换为欧元,可以使用以下代码:
amount = 100
converted_amount = amount * exchange_rate
print(f"{amount} {base_currency} is equal to {converted_amount:.2f} {target_currency}")
在这个示例中,amount
是要转换的金额,exchange_rate
是从API获取的汇率。转换后的金额通过乘以汇率计算得到,并格式化输出。
二、使用固定汇率
虽然使用API获取实时汇率是最准确的方法,但有时我们可能不需要实时汇率,而是使用固定的汇率进行转换。例如,在某些离线应用中,或者需要转换的货币对汇率波动不大时,可以使用预先设定的固定汇率。
- 定义固定汇率
首先,需要定义货币对的固定汇率。可以使用一个字典来存储这些汇率。例如,将美元转换为欧元的汇率设为0.85,将美元转换为日元的汇率设为110,可以使用以下代码:
exchange_rates = {
"USD_EUR": 0.85,
"USD_JPY": 110.0,
# 添加其他货币对和相应汇率
}
- 进行货币转换
有了固定汇率后,可以进行货币转换。例如,将100美元转换为欧元,可以使用以下代码:
amount = 100
base_currency = "USD"
target_currency = "EUR"
exchange_rate = exchange_rates[f"{base_currency}_{target_currency}"]
converted_amount = amount * exchange_rate
print(f"{amount} {base_currency} is equal to {converted_amount:.2f} {target_currency}")
在这个示例中,amount
是要转换的金额,base_currency
是基准货币,target_currency
是目标货币。汇率从定义的字典中获取,并用于计算转换后的金额。
三、利用Python库
除了直接调用API或使用固定汇率外,还可以利用一些专门用于货币转换的Python库。例如,forex-python是一个流行的库,它提供了简单的接口来获取汇率和进行货币转换。
- 安装forex-python库
首先,需要安装forex-python库。可以使用以下命令通过pip进行安装:
pip install forex-python
- 使用forex-python进行货币转换
安装完成后,可以使用forex-python库进行货币转换。以下是一个示例代码:
from forex_python.converter import CurrencyRates
amount = 100
base_currency = "USD"
target_currency = "EUR"
currency_rates = CurrencyRates()
exchange_rate = currency_rates.get_rate(base_currency, target_currency)
converted_amount = currency_rates.convert(base_currency, target_currency, amount)
print(f"Exchange rate from {base_currency} to {target_currency}: {exchange_rate}")
print(f"{amount} {base_currency} is equal to {converted_amount:.2f} {target_currency}")
在这个示例中,CurrencyRates
类用于获取汇率和进行货币转换。get_rate
方法返回指定货币对的汇率,convert
方法根据汇率进行金额转换。
四、处理异常情况
在进行货币转换的过程中,可能会遇到一些异常情况,例如API请求失败、汇率数据缺失等。为了提高程序的健壮性,需要处理这些异常情况。
- 处理API请求失败
在调用API获取汇率数据时,可能会遇到网络问题、API服务不可用等情况。可以使用try-except语句捕获这些异常,并进行相应的处理。例如:
import requests
try:
response = requests.get(url)
response.raise_for_status()
data = response.json()
if data["result"] == "success":
exchange_rate = data["conversion_rates"][target_currency]
print(f"Exchange rate from {base_currency} to {target_currency}: {exchange_rate}")
else:
print("Error fetching exchange rate data")
except requests.exceptions.RequestException as e:
print(f"Error: {e}")
在这个示例中,requests.get
方法可能会抛出请求异常,response.raise_for_status
方法会在请求失败时抛出HTTP错误。通过捕获这些异常,可以避免程序崩溃,并输出错误信息。
- 处理汇率数据缺失
在使用固定汇率或API获取的汇率数据时,可能会遇到某些货币对的汇率数据缺失的情况。可以使用if语句检查汇率数据是否存在,并进行相应的处理。例如:
if f"{base_currency}_{target_currency}" in exchange_rates:
exchange_rate = exchange_rates[f"{base_currency}_{target_currency}"]
converted_amount = amount * exchange_rate
print(f"{amount} {base_currency} is equal to {converted_amount:.2f} {target_currency}")
else:
print(f"Error: Exchange rate for {base_currency} to {target_currency} not found")
在这个示例中,if
语句检查字典中是否存在指定货币对的汇率数据,如果不存在,则输出错误信息。
五、扩展功能
在基本的货币转换功能之外,还可以添加一些扩展功能,使程序更加实用和全面。
- 支持多种货币对
可以扩展程序,使其支持多种货币对的转换。可以使用一个循环来遍历多个货币对,并进行相应的转换。例如:
amount = 100
base_currency = "USD"
target_currencies = ["EUR", "JPY", "GBP"]
for target_currency in target_currencies:
if f"{base_currency}_{target_currency}" in exchange_rates:
exchange_rate = exchange_rates[f"{base_currency}_{target_currency}"]
converted_amount = amount * exchange_rate
print(f"{amount} {base_currency} is equal to {converted_amount:.2f} {target_currency}")
else:
print(f"Error: Exchange rate for {base_currency} to {target_currency} not found")
在这个示例中,target_currencies
是一个包含多个目标货币的列表,通过循环遍历每个目标货币,并进行相应的转换。
- 支持用户输入
可以扩展程序,使其支持用户输入基准货币、目标货币和金额。可以使用input函数获取用户输入,并进行相应的处理。例如:
base_currency = input("Enter base currency: ").upper()
target_currency = input("Enter target currency: ").upper()
amount = float(input("Enter amount: "))
if f"{base_currency}_{target_currency}" in exchange_rates:
exchange_rate = exchange_rates[f"{base_currency}_{target_currency}"]
converted_amount = amount * exchange_rate
print(f"{amount} {base_currency} is equal to {converted_amount:.2f} {target_currency}")
else:
print(f"Error: Exchange rate for {base_currency} to {target_currency} not found")
在这个示例中,用户输入的基准货币、目标货币和金额会被转换为大写,并进行相应的货币转换。
通过以上步骤,我们可以实现一个功能全面的货币转换程序。无论是通过调用API获取实时汇率、使用固定汇率,还是利用Python库,都可以满足不同场景的需求。在实际应用中,可以根据具体需求选择合适的方法,并处理异常情况,确保程序的健壮性和可靠性。
相关问答FAQs:
如何使用Python进行货币转换的基本步骤是什么?
要进行货币转换,您需要获取实时汇率数据。可以通过API(如ExchangeRate API、Open Exchange Rates等)获取汇率信息。接下来,编写一个简单的Python程序,输入金额和货币类型,利用获取的汇率进行计算,最后输出转换后的金额。
有没有推荐的Python库可以帮助进行货币转换?
是的,Python中有多个库可以简化货币转换的过程。例如,forex-python
库提供了方便的接口来获取汇率并进行货币转换。使用这个库,您只需安装它并调用相应的函数即可轻松完成转换。
在进行货币转换时,如何处理汇率波动对结果的影响?
汇率常常会发生波动,因此建议在进行货币转换时定期更新汇率信息。可以设置一个定时任务,每隔一定时间(例如每小时或每天)从API获取最新的汇率数据,以确保您的转换结果更为准确。同时,考虑到汇率的波动,您也可以使用平均汇率进行长期交易的估算。
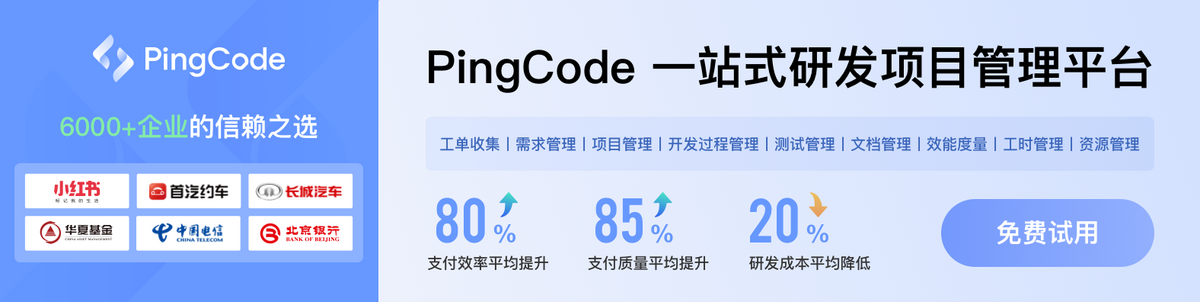