Python3集合操作
Python3中的集合是一种无序且不重复的元素集,它主要用于去重和集合运算。创建集合、添加元素、删除元素、集合运算是Python3集合操作的核心。下面将详细介绍如何在Python3中操作集合,并提供一些具体的示例和技巧。
一、创建集合
在Python3中,可以使用大括号 {}
或者 set()
函数来创建集合。需要注意的是,空集合必须使用 set()
函数来创建,因为 {}
创建的是空字典。
# 使用大括号创建集合
set1 = {1, 2, 3, 4, 5}
print(set1) # 输出: {1, 2, 3, 4, 5}
使用 set() 函数创建集合
set2 = set([1, 2, 3, 4, 5])
print(set2) # 输出: {1, 2, 3, 4, 5}
创建一个空集合
empty_set = set()
print(empty_set) # 输出: set()
二、添加元素
可以使用 add()
方法向集合中添加元素,集合会自动忽略重复的元素。
set1 = {1, 2, 3}
set1.add(4)
print(set1) # 输出: {1, 2, 3, 4}
尝试添加重复元素
set1.add(3)
print(set1) # 输出: {1, 2, 3, 4}
三、删除元素
可以使用 remove()
或 discard()
方法来删除集合中的元素。remove()
方法在元素不存在时会抛出 KeyError
异常,而 discard()
方法则不会。
set1 = {1, 2, 3, 4, 5}
set1.remove(3)
print(set1) # 输出: {1, 2, 4, 5}
尝试删除不存在的元素
set1.remove(10) # 抛出 KeyError
set1.discard(10) # 不会抛出异常
print(set1) # 输出: {1, 2, 4, 5}
四、集合运算
Python3集合支持多种集合运算,如并集、交集、差集和对称差集。
- 并集
并集操作可以使用 union()
方法或 |
运算符。
set1 = {1, 2, 3}
set2 = {3, 4, 5}
union_set = set1.union(set2)
print(union_set) # 输出: {1, 2, 3, 4, 5}
使用 | 运算符
union_set = set1 | set2
print(union_set) # 输出: {1, 2, 3, 4, 5}
- 交集
交集操作可以使用 intersection()
方法或 &
运算符。
set1 = {1, 2, 3}
set2 = {3, 4, 5}
intersection_set = set1.intersection(set2)
print(intersection_set) # 输出: {3}
使用 & 运算符
intersection_set = set1 & set2
print(intersection_set) # 输出: {3}
- 差集
差集操作可以使用 difference()
方法或 -
运算符。
set1 = {1, 2, 3}
set2 = {3, 4, 5}
difference_set = set1.difference(set2)
print(difference_set) # 输出: {1, 2}
使用 - 运算符
difference_set = set1 - set2
print(difference_set) # 输出: {1, 2}
- 对称差集
对称差集操作可以使用 symmetric_difference()
方法或 ^
运算符。
set1 = {1, 2, 3}
set2 = {3, 4, 5}
symmetric_difference_set = set1.symmetric_difference(set2)
print(symmetric_difference_set) # 输出: {1, 2, 4, 5}
使用 ^ 运算符
symmetric_difference_set = set1 ^ set2
print(symmetric_difference_set) # 输出: {1, 2, 4, 5}
五、集合的其他操作
除了基本的集合运算之外,Python3的集合还提供了一些其他常用的方法,如 isdisjoint()
、issubset()
、issuperset()
等。
- 判断两个集合是否不相交
使用 isdisjoint()
方法可以判断两个集合是否不相交,如果两个集合没有相同的元素则返回 True
,否则返回 False
。
set1 = {1, 2, 3}
set2 = {4, 5, 6}
print(set1.isdisjoint(set2)) # 输出: True
set3 = {3, 4, 5}
print(set1.isdisjoint(set3)) # 输出: False
- 判断子集
使用 issubset()
方法可以判断一个集合是否是另一个集合的子集。
set1 = {1, 2, 3}
set2 = {1, 2, 3, 4, 5}
print(set1.issubset(set2)) # 输出: True
set3 = {1, 2, 6}
print(set1.issubset(set3)) # 输出: False
- 判断超集
使用 issuperset()
方法可以判断一个集合是否包含另一个集合,即是否为其超集。
set1 = {1, 2, 3, 4, 5}
set2 = {1, 2, 3}
print(set1.issuperset(set2)) # 输出: True
set3 = {1, 2, 6}
print(set1.issuperset(set3)) # 输出: False
六、集合的遍历
集合是无序的,因此无法通过索引来访问集合中的元素,但可以使用 for
循环来遍历集合中的每一个元素。
set1 = {1, 2, 3, 4, 5}
for element in set1:
print(element)
七、集合的常见应用
- 数据去重
集合最常见的应用之一就是数据去重。由于集合中的元素是唯一的,可以利用集合的这一特性来去除列表中的重复元素。
list1 = [1, 2, 2, 3, 4, 4, 5]
unique_list = list(set(list1))
print(unique_list) # 输出: [1, 2, 3, 4, 5]
- 集合运算
集合运算在许多实际问题中都有应用,例如统计两个数据集的交集、并集等。
students_A = {"Alice", "Bob", "Charlie"}
students_B = {"Bob", "David", "Edward"}
并集
all_students = students_A.union(students_B)
print(all_students) # 输出: {'Alice', 'Bob', 'Charlie', 'David', 'Edward'}
交集
common_students = students_A.intersection(students_B)
print(common_students) # 输出: {'Bob'}
差集
only_A_students = students_A.difference(students_B)
print(only_A_students) # 输出: {'Alice', 'Charlie'}
对称差集
distinct_students = students_A.symmetric_difference(students_B)
print(distinct_students) # 输出: {'Alice', 'Charlie', 'David', 'Edward'}
总结
Python3集合是一种非常有用的数据结构,特别适用于需要处理无序且不重复元素的场景。通过掌握集合的基本操作、集合运算及其应用,可以在实际编程中更加高效地解决问题。希望通过本篇博客的介绍,能够帮助你更好地理解和使用Python3中的集合。
相关问答FAQs:
如何在Python3中创建集合?
在Python3中,可以使用大括号 {}
或者 set()
函数来创建集合。举个例子,使用大括号可以这样创建:my_set = {1, 2, 3}
,而使用 set()
函数则可以这样创建:my_set = set([1, 2, 3])
。请注意,集合中的元素是唯一的,因此如果在创建集合时包含重复元素,重复的部分将会被自动去除。
集合支持哪些常见的操作?
Python3中的集合支持多种操作,如并集、交集、差集和对称差集。可以使用 union()
方法或 |
运算符来执行并集操作,使用 intersection()
方法或 &
运算符来执行交集操作,使用 difference()
方法或 -
运算符来执行差集操作,使用 symmetric_difference()
方法或 ^
运算符来执行对称差集操作。这些操作使得集合在处理数据时非常灵活和方便。
如何在集合中添加或删除元素?
要在集合中添加元素,可以使用 add()
方法,例如 my_set.add(4)
。如果想要添加多个元素,可以使用 update()
方法,它可以接受一个列表、元组或其他可迭代对象。要删除元素,可以使用 remove()
方法,如果元素不存在则会引发错误;也可以使用 discard()
方法,该方法在元素不存在时不会引发错误。还有 pop()
方法可以随机删除并返回一个元素,但请注意,集合是无序的,因此无法确定删除的是哪个元素。
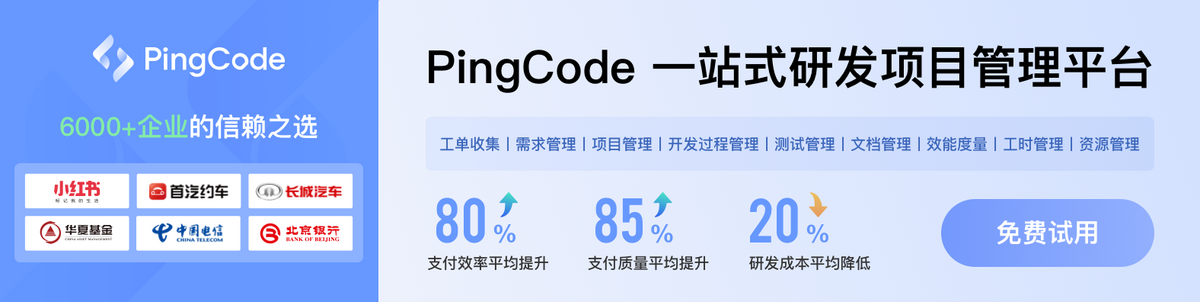