使用Python写工具破解密码的方法有很多,主要包括:字典攻击、暴力破解、哈希破解等。在本文中,我们将详细介绍这些方法,并提供一些示例代码来展示如何实现这些破解技术。通过这些方法,你可以更加深入地理解密码破解的原理和技术。
一、字典攻击
字典攻击是通过预先准备好的常用密码列表(即字典)来进行尝试匹配的破解方法。这种方法效率较高,但仅限于字典中包含的密码。
1.1、准备字典文件
首先,我们需要准备一个包含常用密码的字典文件。这个文件可以自己编写,或者从网上下载现成的字典文件。
password123
123456
qwerty
letmein
password1
...
1.2、实现字典攻击工具
以下是一个简单的字典攻击示例代码:
import hashlib
def hash_password(password):
return hashlib.md5(password.encode()).hexdigest()
def dictionary_attack(hash_to_crack, dictionary_file):
with open(dictionary_file, 'r') as file:
for line in file:
password = line.strip()
hashed_password = hash_password(password)
if hashed_password == hash_to_crack:
print(f"Password found: {password}")
return password
print("Password not found in dictionary.")
return None
if __name__ == "__main__":
hash_to_crack = "5f4dcc3b5aa765d61d8327deb882cf99" # MD5 hash for 'password'
dictionary_file = "password_dictionary.txt"
dictionary_attack(hash_to_crack, dictionary_file)
这个示例中,我们使用了 hashlib
库来计算密码的 MD5 哈希值。通过遍历字典文件中的每个密码,并计算其哈希值与目标哈希值进行比较,找到匹配的密码。
二、暴力破解
暴力破解是通过尝试所有可能的密码组合来进行匹配的破解方法。这种方法非常耗时,但理论上可以破解任何密码。
2.1、生成所有可能的密码组合
我们可以使用 itertools
库中的 product
函数来生成所有可能的密码组合。
import itertools
import string
import hashlib
def hash_password(password):
return hashlib.md5(password.encode()).hexdigest()
def brute_force_attack(hash_to_crack, max_length):
characters = string.ascii_lowercase + string.digits
for length in range(1, max_length + 1):
for password in itertools.product(characters, repeat=length):
password = ''.join(password)
hashed_password = hash_password(password)
if hashed_password == hash_to_crack:
print(f"Password found: {password}")
return password
print("Password not found.")
return None
if __name__ == "__main__":
hash_to_crack = "5f4dcc3b5aa765d61d8327deb882cf99" # MD5 hash for 'password'
max_length = 5
brute_force_attack(hash_to_crack, max_length)
在这个示例中,我们使用了字母和数字的组合,并限制了密码的最大长度。通过遍历所有可能的密码组合,并计算其哈希值与目标哈希值进行比较,找到匹配的密码。
三、哈希破解
哈希破解是通过预先计算并存储大量密码的哈希值来进行匹配的破解方法。这种方法需要大量的计算和存储资源,但可以大大提高破解速度。
3.1、生成彩虹表
彩虹表是预先计算并存储大量密码的哈希值的表格。我们可以使用 hashlib
库来生成彩虹表。
import hashlib
import itertools
import string
import os
def generate_rainbow_table(filename, max_length):
characters = string.ascii_lowercase + string.digits
with open(filename, 'w') as file:
for length in range(1, max_length + 1):
for password in itertools.product(characters, repeat=length):
password = ''.join(password)
hashed_password = hashlib.md5(password.encode()).hexdigest()
file.write(f"{password}:{hashed_password}\n")
print("Rainbow table generated.")
if __name__ == "__main__":
filename = "rainbow_table.txt"
max_length = 5
generate_rainbow_table(filename, max_length)
3.2、使用彩虹表进行哈希破解
我们可以通过读取彩虹表,并将目标哈希值与表中的哈希值进行比较,找到匹配的密码。
def hash_crack_with_rainbow_table(hash_to_crack, rainbow_table_file):
with open(rainbow_table_file, 'r') as file:
for line in file:
password, hashed_password = line.strip().split(':')
if hashed_password == hash_to_crack:
print(f"Password found: {password}")
return password
print("Password not found in rainbow table.")
return None
if __name__ == "__main__":
hash_to_crack = "5f4dcc3b5aa765d61d8327deb882cf99" # MD5 hash for 'password'
rainbow_table_file = "rainbow_table.txt"
hash_crack_with_rainbow_table(hash_to_crack, rainbow_table_file)
通过以上步骤,我们可以生成彩虹表,并使用彩虹表进行哈希破解。这种方法在实际应用中效果显著,但需要占用大量的存储空间和计算资源。
四、总结
在本文中,我们介绍了使用Python写工具破解密码的几种方法,包括字典攻击、暴力破解和哈希破解。每种方法都有其优缺点,具体选择哪种方法取决于实际需求和场景。需要注意的是,密码破解技术应仅用于合法和道德的用途,切勿滥用这些技术进行非法活动。希望本文能帮助你更好地理解密码破解的原理和技术。
相关问答FAQs:
如何在Python中实现密码破解的基础知识?
在Python中实现密码破解通常涉及了解密码学的基础知识以及如何利用编程语言的特性来自动化这一过程。常用的方法包括字典攻击和暴力破解,前者依赖于一个包含常见密码的字典文件,而后者则尝试所有可能的组合。使用Python的itertools
库可以生成所有可能的密码组合,而hashlib
库则可以用于处理密码的哈希值。
使用Python破解密码是否合法?
在任何情况下,未经授权地破解密码都是非法的。密码破解工具应仅用于合法用途,例如测试自己的系统安全性或教育目的。在使用此类工具时,务必遵循相关法律法规,并获得适当的许可。
如何提高Python密码破解工具的效率?
提高密码破解工具效率的方式有很多。例如,可以使用多线程或异步编程来同时运行多个破解尝试。还可以优化字典文件,确保其中包含高频率使用的密码。此外,使用GPU加速计算也是一种有效的方式,可以大幅缩短破解时间。
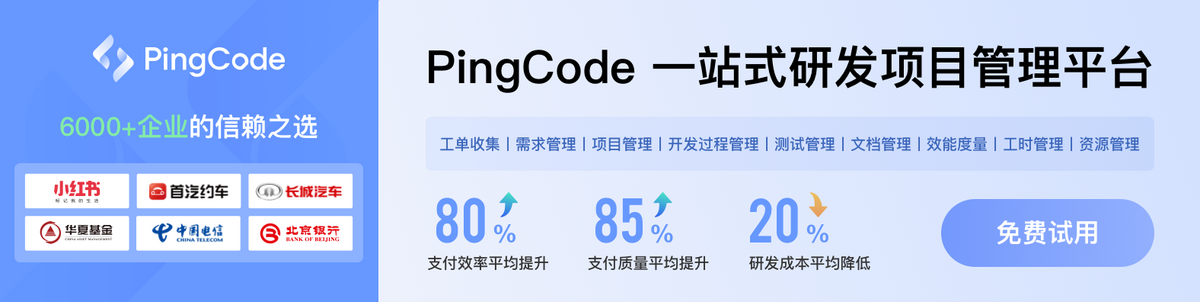