Python选择电脑文件并上传文件的方法包括:使用tkinter库选择文件、使用requests库上传文件、使用Flask创建文件上传服务器。 其中,使用tkinter库选择文件是比较常用和方便的一种方法。
使用tkinter库选择文件可以非常方便地创建一个文件选择对话框,让用户从本地文件系统中选择文件。以下是详细描述:
Tkinter是Python的标准GUI(图形用户界面)库。使用Tkinter可以快速创建简单的GUI应用程序,包括文件选择对话框。文件选择对话框会弹出一个窗口,允许用户浏览文件系统并选择一个或多个文件。选择完文件后,可以获取文件的路径,然后进行后续的操作,如上传文件。
下面的代码示例展示了如何使用Tkinter库选择文件,并打印选中的文件路径:
import tkinter as tk
from tkinter import filedialog
def select_file():
root = tk.Tk()
root.withdraw() # 隐藏主窗口
file_path = filedialog.askopenfilename() # 打开文件选择对话框
print("Selected file:", file_path)
if __name__ == "__main__":
select_file()
在这个代码示例中,我们导入了tkinter
和filedialog
模块,定义了一个select_file
函数来显示文件选择对话框,并打印选中的文件路径。
接下来,我们将详细介绍如何在Python中选择电脑文件并上传文件,包括使用tkinter选择文件、使用requests库上传文件、以及使用Flask创建文件上传服务器的完整过程。
一、使用Tkinter选择文件
1. 安装Tkinter
Tkinter是Python的标准库,一般情况下不需要单独安装。如果你的Python环境中没有Tkinter库,可以通过以下命令安装:
pip install tk
2. 创建文件选择对话框
下面是一个使用Tkinter创建文件选择对话框的完整示例:
import tkinter as tk
from tkinter import filedialog
def select_file():
root = tk.Tk()
root.withdraw() # 隐藏主窗口
file_path = filedialog.askopenfilename(title="Select a file", filetypes=[("All files", "*.*")])
return file_path
if __name__ == "__main__":
file_path = select_file()
if file_path:
print("Selected file:", file_path)
else:
print("No file selected")
在这个示例中,filedialog.askopenfilename
函数打开一个文件选择对话框,用户可以选择一个文件。title
参数设置对话框的标题,filetypes
参数设置文件类型过滤器。
二、使用Requests库上传文件
1. 安装Requests库
Requests是一个简单易用的HTTP库,可以通过以下命令安装:
pip install requests
2. 上传文件
下面是一个使用Requests库上传文件的完整示例:
import requests
def upload_file(file_path, url):
with open(file_path, 'rb') as file:
files = {'file': file}
response = requests.post(url, files=files)
return response
if __name__ == "__main__":
file_path = "path/to/your/file"
upload_url = "https://example.com/upload"
response = upload_file(file_path, upload_url)
print("Response status code:", response.status_code)
print("Response text:", response.text)
在这个示例中,upload_file
函数接受文件路径和上传URL作为参数,通过HTTP POST请求上传文件。文件以二进制模式打开,并通过files
参数传递给requests.post
函数。
三、使用Flask创建文件上传服务器
1. 安装Flask
Flask是一个轻量级的Web框架,可以通过以下命令安装:
pip install flask
2. 创建文件上传服务器
下面是一个使用Flask创建文件上传服务器的完整示例:
from flask import Flask, request, redirect, url_for
import os
app = Flask(__name__)
UPLOAD_FOLDER = 'uploads'
os.makedirs(UPLOAD_FOLDER, exist_ok=True)
app.config['UPLOAD_FOLDER'] = UPLOAD_FOLDER
@app.route('/')
def index():
return '''
<!doctype html>
<title>Upload File</title>
<h1>Upload File</h1>
<form method=post enctype=multipart/form-data>
<input type=file name=file>
<input type=submit value=Upload>
</form>
'''
@app.route('/upload', methods=['POST'])
def upload_file():
if 'file' not in request.files:
return "No file part"
file = request.files['file']
if file.filename == '':
return "No selected file"
file.save(os.path.join(app.config['UPLOAD_FOLDER'], file.filename))
return "File uploaded successfully"
if __name__ == "__main__":
app.run(debug=True)
在这个示例中,我们创建了一个简单的Flask应用,包含一个文件上传表单和一个处理文件上传的路由。用户可以通过表单选择文件并上传到服务器。上传的文件将保存在UPLOAD_FOLDER
目录中。
四、整合选择文件和上传文件功能
最后,我们将选择文件和上传文件的功能整合在一起,创建一个完整的Python程序,让用户选择文件并上传到服务器。
import tkinter as tk
from tkinter import filedialog
import requests
def select_file():
root = tk.Tk()
root.withdraw() # 隐藏主窗口
file_path = filedialog.askopenfilename(title="Select a file", filetypes=[("All files", "*.*")])
return file_path
def upload_file(file_path, url):
with open(file_path, 'rb') as file:
files = {'file': file}
response = requests.post(url, files=files)
return response
if __name__ == "__main__":
file_path = select_file()
if file_path:
upload_url = "https://example.com/upload"
response = upload_file(file_path, upload_url)
print("Response status code:", response.status_code)
print("Response text:", response.text)
else:
print("No file selected")
在这个最终示例中,用户首先选择文件,然后程序将选中的文件上传到指定的URL。上传成功后,程序打印服务器的响应状态码和响应内容。
通过本文的详细介绍,相信你已经掌握了如何在Python中选择电脑文件并上传文件的方法。希望这些内容对你有所帮助!
相关问答FAQs:
如何在Python中选择文件并进行上传?
在Python中,可以使用Tkinter库创建一个文件选择对话框,方便用户选择文件。选择文件后,可以使用requests库或其他相关库将文件上传到指定的服务器。示例代码如下:
import tkinter as tk
from tkinter import filedialog
import requests
def upload_file():
root = tk.Tk()
root.withdraw() # 隐藏主窗口
file_path = filedialog.askopenfilename() # 打开文件选择对话框
if file_path:
url = "http://example.com/upload" # 替换为你的上传地址
with open(file_path, 'rb') as f:
response = requests.post(url, files={'file': f}) # 上传文件
print("上传结果:", response.text)
upload_file()
如何处理文件上传时的错误?
在文件上传时,可能会遇到多种错误,例如网络连接问题或文件类型不被支持。可以通过捕获异常来处理这些错误,例如使用try-except块。确保在上传之前检查文件的类型和大小,以减少上传失败的可能性。
是否有图形用户界面(GUI)可用于文件选择和上传?
是的,可以使用Tkinter等库创建一个简单的GUI,用户可以通过按钮选择文件并上传。利用Tkinter的组件,如Button和Label,可以构建一个友好的界面,提升用户体验。以下是一个简单的示例:
import tkinter as tk
from tkinter import filedialog
import requests
def upload_file():
file_path = filedialog.askopenfilename()
if file_path:
url = "http://example.com/upload"
with open(file_path, 'rb') as f:
response = requests.post(url, files={'file': f})
result_label.config(text="上传结果: " + response.text)
app = tk.Tk()
app.title("文件上传")
upload_button = tk.Button(app, text="选择文件并上传", command=upload_file)
upload_button.pack()
result_label = tk.Label(app, text="")
result_label.pack()
app.mainloop()
通过这种方式,用户可以轻松选择文件并将其上传。
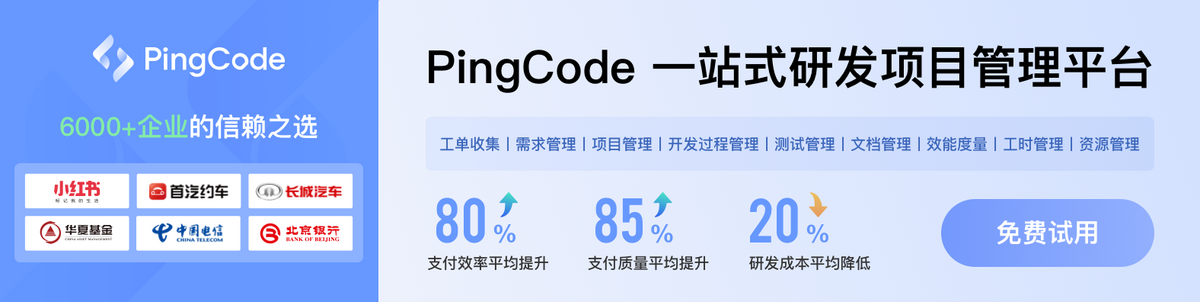