Python绘图转换成动图的方法有多种,主要通过使用Matplotlib、Plotly、或Manim等库来实现,具体步骤包括生成静态图像、合成动画、保存动图。 本文将详细讲解其中一种方法,即使用Matplotlib和其动画模块实现Python绘图转换成动图的具体步骤。
下面将详细介绍如何使用Matplotlib库将静态图转换成动图,并提供代码示例和应用场景。
一、MATPLOTLIB库简介
Matplotlib是Python中最著名的绘图库之一,广泛用于数据可视化。通过其子模块matplotlib.animation
,我们可以很方便地创建动画。该模块提供了丰富的功能,包括创建动态图、保存动画等。下面我们将通过一个实际的示例来演示如何使用Matplotlib创建动画。
1、安装和导入Matplotlib
首先,确保已安装Matplotlib库。如果未安装,可以使用以下命令进行安装:
pip install matplotlib
安装完成后,我们可以在代码中导入Matplotlib及其动画模块:
import matplotlib.pyplot as plt
import matplotlib.animation as animation
2、创建一个简单的动画
下面是一个简单的示例,演示如何使用Matplotlib创建一个动态的正弦曲线。
import numpy as np
import matplotlib.pyplot as plt
import matplotlib.animation as animation
创建一个新的图表
fig, ax = plt.subplots()
x = np.linspace(0, 2*np.pi, 100)
line, = ax.plot(x, np.sin(x))
初始化函数
def init():
line.set_ydata(np.sin(x))
return line,
动画函数
def animate(i):
line.set_ydata(np.sin(x + i / 10.0)) # 更新y数据
return line,
创建动画
ani = animation.FuncAnimation(fig, animate, frames=100, init_func=init, interval=20, blit=True)
plt.show()
在这个示例中,我们首先创建一个正弦曲线的静态图,然后通过更新曲线的y数据来生成动画。animation.FuncAnimation
函数是创建动画的核心。
二、保存动画
创建动画后,我们通常希望将其保存为文件。Matplotlib支持多种格式的动画保存,包括GIF、MP4等。下面是将上面的动画保存为GIF文件的示例:
ani.save('sine_wave.gif', writer='imagemagick')
要保存为MP4格式,可以使用ffmpeg
作为写入器:
ani.save('sine_wave.mp4', writer='ffmpeg')
确保已经安装了imagemagick
或ffmpeg
,可以通过以下命令进行安装:
sudo apt-get install imagemagick
sudo apt-get install ffmpeg
三、复杂动画示例
1、双摆系统动画
下面是一个更复杂的示例,演示如何使用Matplotlib创建一个双摆系统的动画。
import numpy as np
import matplotlib.pyplot as plt
import matplotlib.animation as animation
双摆系统参数
L1, L2 = 1.0, 1.0 # 摆长
M1, M2 = 1.0, 1.0 # 摆锤质量
G = 9.8 # 重力加速度
初始条件
theta1, theta2 = np.pi / 2, np.pi / 2
omega1, omega2 = 0.0, 0.0
时间步长
dt = 0.05
t_max = 20
数值积分(Runge-Kutta方法)
def derivs(state, t):
dydx = np.zeros_like(state)
dydx[0] = state[1]
delta = state[2] - state[0]
den1 = (M1 + M2) * L1 - M2 * L1 * np.cos(delta) * np.cos(delta)
dydx[1] = ((M2 * L1 * state[1] * state[1] * np.sin(delta) * np.cos(delta)
+ M2 * G * np.sin(state[2]) * np.cos(delta)
+ M2 * L2 * state[3] * state[3] * np.sin(delta)
- (M1 + M2) * G * np.sin(state[0]))
/ den1)
dydx[2] = state[3]
den2 = (L2 / L1) * den1
dydx[3] = ((- M2 * L2 * state[3] * state[3] * np.sin(delta) * np.cos(delta)
+ (M1 + M2) * G * np.sin(state[0]) * np.cos(delta)
- (M1 + M2) * L1 * state[1] * state[1] * np.sin(delta)
- (M1 + M2) * G * np.sin(state[2]))
/ den2)
return dydx
初始化状态向量
state = np.radians([theta1, omega1, theta2, omega2])
时间数组
t = np.arange(0, t_max, dt)
数组存储解
theta1_sol = []
theta2_sol = []
数值积分
from scipy.integrate import odeint
sol = odeint(derivs, state, t)
theta1_sol = sol[:, 0]
theta2_sol = sol[:, 2]
转换坐标
x1 = L1 * np.sin(theta1_sol)
y1 = -L1 * np.cos(theta1_sol)
x2 = L2 * np.sin(theta2_sol) + x1
y2 = -L2 * np.cos(theta2_sol) + y1
创建图表
fig, ax = plt.subplots()
ax.set_xlim(-2, 2)
ax.set_ylim(-2, 2)
line, = ax.plot([], [], 'o-', lw=2)
time_template = 'time = %.1fs'
time_text = ax.text(0.05, 0.9, '', transform=ax.transAxes)
初始化函数
def init():
line.set_data([], [])
time_text.set_text('')
return line, time_text
动画函数
def animate(i):
thisx = [0, x1[i], x2[i]]
thisy = [0, y1[i], y2[i]]
line.set_data(thisx, thisy)
time_text.set_text(time_template % (i * dt))
return line, time_text
创建动画
ani = animation.FuncAnimation(fig, animate, frames=len(t),
interval=dt*1000, blit=True, init_func=init)
plt.show()
在这个示例中,我们使用Runge-Kutta方法进行数值积分,计算双摆系统的运动轨迹。然后,我们使用Matplotlib的动画模块将轨迹转换为动画。
四、其他动画库
除了Matplotlib,Python还有其他一些强大的动画库,比如Plotly和Manim。
1、Plotly
Plotly是一个强大的交互式绘图库,支持多种图表类型和动画。下面是使用Plotly创建动画的简单示例:
import plotly.graph_objects as go
import numpy as np
x = np.linspace(0, 2*np.pi, 100)
y = np.sin(x)
fig = go.Figure(
data=[go.Scatter(x=x, y=y, mode='lines')],
layout=go.Layout(
title="Sine Wave Animation",
updatemenus=[dict(type="buttons",
buttons=[dict(label="Play",
method="animate",
args=[None])])]
),
frames=[go.Frame(data=[go.Scatter(x=x, y=np.sin(x + i/10.0))])
for i in range(100)]
)
fig.show()
2、Manim
Manim是一个动画引擎,特别适合制作数学动画。Manim由3Blue1Brown创建,用于生成高质量的数学视频。下面是使用Manim创建动画的示例:
from manim import *
class SineWave(Scene):
def construct(self):
axes = Axes(
x_range=[0, 2 * PI, PI / 4],
y_range=[-1, 1, 0.5],
axis_config={"color": BLUE}
)
sin_graph = axes.plot(lambda x: np.sin(x), color=YELLOW)
self.play(Create(axes), Create(sin_graph))
self.wait()
在这个示例中,我们使用Manim创建了一个简单的正弦波动画。
总结
Python绘图转换成动图的方法有很多,本文主要介绍了使用Matplotlib库创建动画的详细步骤,并提供了Plotly和Manim的简要示例。通过这些方法,我们可以轻松地将静态图转换成动态动画,用于数据可视化、科学计算、教育等多个领域。
相关问答FAQs:
如何将Python绘图结果保存为动图格式?
将Python中的绘图结果转换成动图通常可以使用matplotlib
库配合PIL
或imageio
库来实现。首先,使用matplotlib
创建所需的静态图像,然后将这些图像保存为GIF格式。可以通过循环生成多个图像帧并使用imageio
中的get_writer
函数将它们合并为一个动图。
在Python中制作动图需要哪些库?
制作动图时,通常需要安装matplotlib
和imageio
两个库。matplotlib
用于绘制图形,而imageio
用于处理图像的读取和写入。可以通过pip install matplotlib imageio
命令快速安装这两个库。
动图的帧速率如何调整?
在使用imageio
创建动图时,可以通过duration
参数来调整每帧之间的时间间隔,从而控制动图的播放速度。具体来说,imageio.mimsave('output.gif', images, duration=0.1)
将每帧之间的时间设置为0.1秒,您可以根据需要进行调整。
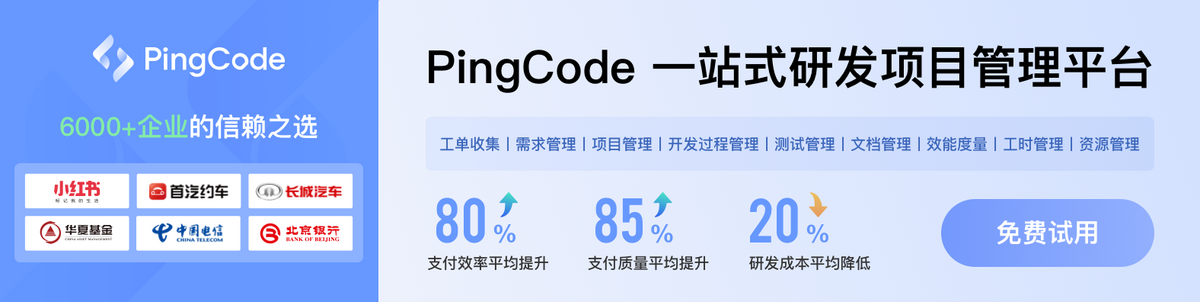