编写Python配置文件的方法包括使用configparser、yaml和json模块。 使用这些模块可以使配置文件更加清晰易读、易于维护,并且可以在不同环境中方便地进行配置管理。下面将详细介绍这几种方法中的一种:configparser,并提供相关示例。
一、CONFIGPARSER模块
ConfigParser模块是Python标准库的一部分,用于处理配置文件。配置文件通常是.ini格式的文本文件,包含键值对。使用ConfigParser可以方便地读取和写入这些配置文件。
1、创建配置文件
创建一个名为config.ini的文件,并添加以下内容:
[DEFAULT]
ServerAliveInterval = 45
Compression = yes
CompressionLevel = 9
ForwardX11 = yes
[bitbucket.org]
User = hg
[topsecret.server.com]
Port = 50022
ForwardX11 = no
2、读取配置文件
使用ConfigParser模块读取配置文件并获取其中的值:
import configparser
config = configparser.ConfigParser()
config.read('config.ini')
读取DEFAULT部分的值
server_alive_interval = config['DEFAULT']['ServerAliveInterval']
compression = config['DEFAULT']['Compression']
compression_level = config['DEFAULT']['CompressionLevel']
读取bitbucket.org部分的值
user = config['bitbucket.org']['User']
读取topsecret.server.com部分的值
port = config['topsecret.server.com']['Port']
forward_x11 = config['topsecret.server.com']['ForwardX11']
print(f"ServerAliveInterval: {server_alive_interval}")
print(f"Compression: {compression}")
print(f"CompressionLevel: {compression_level}")
print(f"User: {user}")
print(f"Port: {port}")
print(f"ForwardX11: {forward_x11}")
3、写入配置文件
使用ConfigParser模块写入配置文件:
import configparser
config = configparser.ConfigParser()
config['DEFAULT'] = {
'ServerAliveInterval': '45',
'Compression': 'yes',
'CompressionLevel': '9',
'ForwardX11': 'yes'
}
config['bitbucket.org'] = {'User': 'hg'}
config['topsecret.server.com'] = {
'Port': '50022',
'ForwardX11': 'no'
}
with open('config.ini', 'w') as configfile:
config.write(configfile)
二、YAML模块
YAML(YAML Ain't Markup Language)是一种人类可读的数据序列化标准,广泛用于配置文件。Python提供了PyYAML库来处理YAML文件。
1、安装PyYAML
首先,安装PyYAML库:
pip install pyyaml
2、创建YAML配置文件
创建一个名为config.yaml的文件,并添加以下内容:
default:
server_alive_interval: 45
compression: yes
compression_level: 9
forward_x11: yes
bitbucket.org:
user: hg
topsecret.server.com:
port: 50022
forward_x11: no
3、读取YAML配置文件
使用PyYAML库读取YAML配置文件并获取其中的值:
import yaml
with open('config.yaml', 'r') as file:
config = yaml.safe_load(file)
读取default部分的值
server_alive_interval = config['default']['server_alive_interval']
compression = config['default']['compression']
compression_level = config['default']['compression_level']
forward_x11 = config['default']['forward_x11']
读取bitbucket.org部分的值
user = config['bitbucket.org']['user']
读取topsecret.server.com部分的值
port = config['topsecret.server.com']['port']
forward_x11_topsecret = config['topsecret.server.com']['forward_x11']
print(f"ServerAliveInterval: {server_alive_interval}")
print(f"Compression: {compression}")
print(f"CompressionLevel: {compression_level}")
print(f"User: {user}")
print(f"Port: {port}")
print(f"ForwardX11: {forward_x11_topsecret}")
4、写入YAML配置文件
使用PyYAML库写入YAML配置文件:
import yaml
config = {
'default': {
'server_alive_interval': 45,
'compression': 'yes',
'compression_level': 9,
'forward_x11': 'yes'
},
'bitbucket.org': {
'user': 'hg'
},
'topsecret.server.com': {
'port': 50022,
'forward_x11': 'no'
}
}
with open('config.yaml', 'w') as file:
yaml.dump(config, file)
三、JSON模块
JSON(JavaScript Object Notation)是一种轻量级的数据交换格式,易于人类阅读和编写,也易于机器解析和生成。Python提供了json模块来处理JSON文件。
1、创建JSON配置文件
创建一个名为config.json的文件,并添加以下内容:
{
"default": {
"server_alive_interval": 45,
"compression": "yes",
"compression_level": 9,
"forward_x11": "yes"
},
"bitbucket.org": {
"user": "hg"
},
"topsecret.server.com": {
"port": 50022,
"forward_x11": "no"
}
}
2、读取JSON配置文件
使用json模块读取JSON配置文件并获取其中的值:
import json
with open('config.json', 'r') as file:
config = json.load(file)
读取default部分的值
server_alive_interval = config['default']['server_alive_interval']
compression = config['default']['compression']
compression_level = config['default']['compression_level']
forward_x11 = config['default']['forward_x11']
读取bitbucket.org部分的值
user = config['bitbucket.org']['user']
读取topsecret.server.com部分的值
port = config['topsecret.server.com']['port']
forward_x11_topsecret = config['topsecret.server.com']['forward_x11']
print(f"ServerAliveInterval: {server_alive_interval}")
print(f"Compression: {compression}")
print(f"CompressionLevel: {compression_level}")
print(f"User: {user}")
print(f"Port: {port}")
print(f"ForwardX11: {forward_x11_topsecret}")
3、写入JSON配置文件
使用json模块写入JSON配置文件:
import json
config = {
'default': {
'server_alive_interval': 45,
'compression': 'yes',
'compression_level': 9,
'forward_x11': 'yes'
},
'bitbucket.org': {
'user': 'hg'
},
'topsecret.server.com': {
'port': 50022,
'forward_x11': 'no'
}
}
with open('config.json', 'w') as file:
json.dump(config, file, indent=4)
四、总结
通过使用ConfigParser、YAML和JSON模块,可以轻松地创建和管理Python配置文件。这些方法各有优缺点,可以根据具体需求选择合适的方式。ConfigParser适用于简单的.ini格式配置文件,YAML适用于更复杂的层次结构配置文件,JSON则适用于需要与其他系统进行数据交换的情况。
无论选择哪种方式,保持配置文件的清晰、易读和易于维护是关键。通过合理的配置文件管理,可以使代码更具可移植性和可维护性,从而提高开发效率和代码质量。
相关问答FAQs:
如何选择适合的配置文件格式?
在编写Python配置文件时,可以选择多种格式,例如JSON、YAML、INI或TOML。每种格式都有其优缺点,例如,JSON易于阅读和解析,但不支持注释,而YAML则支持更复杂的结构和注释。根据项目需求和团队的熟悉程度,选择最合适的格式将有助于提高可读性和可维护性。
配置文件应该包含哪些基本信息?
一个优秀的Python配置文件通常应包含应用程序的核心设置,例如数据库连接信息、API密钥、文件路径、日志级别等。确保对这些关键参数进行合理的组织,以便于后续的管理和修改。同时,考虑将敏感信息(如密码)存储在安全的地方,避免直接写入配置文件。
如何在Python中读取和解析配置文件?
在Python中,可以使用内置的configparser
模块读取INI格式的配置文件,使用json
模块解析JSON文件,或使用PyYAML
库处理YAML格式的文件。具体步骤包括导入相应的模块、打开配置文件并读取内容,然后根据需要将数据转换为适合的格式。通过这种方式,您可以轻松地在代码中访问配置项。
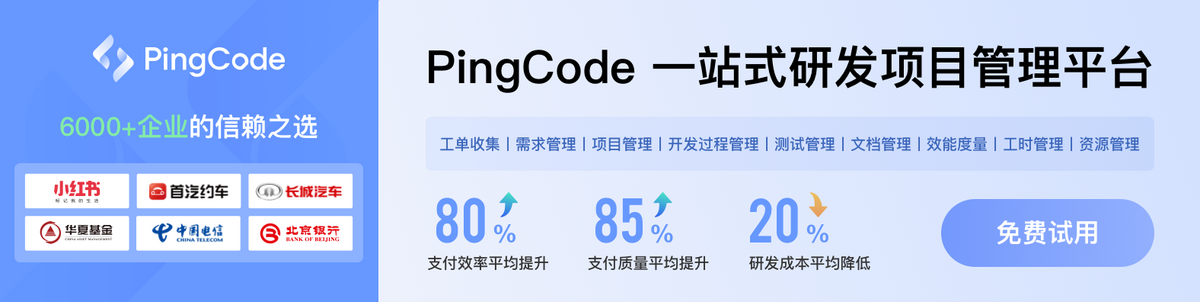