Python求最小二乘法的方法有多种,常见的方法包括使用Numpy、Scipy和Statsmodels库。使用Numpy进行线性回归、使用Scipy库中的curve_fit函数、使用Statsmodels库进行详细回归分析。在下面的文章中,我们将详细介绍如何使用这些方法来求最小二乘法,并给出相关代码示例。
一、使用Numpy进行线性回归
Numpy是Python中一个强大的数学库,提供了丰富的线性代数操作函数。我们可以使用Numpy的polyfit
函数进行线性回归,求得最小二乘法拟合的系数。
示例代码:
import numpy as np
import matplotlib.pyplot as plt
生成模拟数据
x = np.array([0, 1, 2, 3, 4, 5])
y = np.array([2, 3, 5, 7, 11, 14])
使用Numpy的polyfit函数进行线性回归
coefficients = np.polyfit(x, y, 1)
slope, intercept = coefficients
打印回归系数
print(f"Slope: {slope}, Intercept: {intercept}")
绘制数据点和回归直线
plt.scatter(x, y, color='red', label='Data Points')
plt.plot(x, slope * x + intercept, label='Fitted Line')
plt.legend()
plt.show()
在上面的代码中,我们使用numpy.polyfit
函数来计算线性回归的系数,其中1
表示一阶多项式(即线性)。我们得到斜率和截距后,可以绘制出数据点和回归直线。
二、使用Scipy库中的curve_fit函数
Scipy库是Python中一个用于科学计算的库,提供了许多优化和拟合函数。我们可以使用scipy.optimize.curve_fit
函数进行非线性最小二乘法拟合。
示例代码:
import numpy as np
from scipy.optimize import curve_fit
import matplotlib.pyplot as plt
定义一个线性函数
def linear_function(x, a, b):
return a * x + b
生成模拟数据
x = np.array([0, 1, 2, 3, 4, 5])
y = np.array([2, 3, 5, 7, 11, 14])
使用curve_fit函数进行拟合
params, covariance = curve_fit(linear_function, x, y)
打印拟合参数
print(f"Slope: {params[0]}, Intercept: {params[1]}")
绘制数据点和拟合曲线
plt.scatter(x, y, color='red', label='Data Points')
plt.plot(x, linear_function(x, *params), label='Fitted Line')
plt.legend()
plt.show()
在上面的代码中,我们首先定义了一个线性函数linear_function
,然后使用curve_fit
函数进行拟合。curve_fit
函数会返回拟合参数和协方差矩阵,我们可以从中提取出斜率和截距。
三、使用Statsmodels库进行详细回归分析
Statsmodels库是Python中一个专门用于统计建模的库,提供了详细的回归分析功能。我们可以使用Statsmodels库进行线性回归,并获得详细的回归结果和统计信息。
示例代码:
import numpy as np
import statsmodels.api as sm
import matplotlib.pyplot as plt
生成模拟数据
x = np.array([0, 1, 2, 3, 4, 5])
y = np.array([2, 3, 5, 7, 11, 14])
添加常数项
x = sm.add_constant(x)
创建回归模型
model = sm.OLS(y, x)
results = model.fit()
打印回归结果
print(results.summary())
绘制数据点和回归直线
plt.scatter(x[:, 1], y, color='red', label='Data Points')
plt.plot(x[:, 1], results.predict(x), label='Fitted Line')
plt.legend()
plt.show()
在上面的代码中,我们使用statsmodels.api.OLS
函数进行线性回归,并使用fit
方法拟合模型。results.summary()
方法会输出详细的回归结果,包括回归系数、统计显著性检验、R平方值等。
四、使用Pandas和Numpy结合进行回归分析
Pandas库是Python中一个强大的数据分析库,常用于数据处理和分析。我们可以将Pandas和Numpy结合使用,进行回归分析并绘制结果。
示例代码:
import numpy as np
import pandas as pd
import matplotlib.pyplot as plt
生成模拟数据
data = {
'x': [0, 1, 2, 3, 4, 5],
'y': [2, 3, 5, 7, 11, 14]
}
df = pd.DataFrame(data)
使用Numpy进行线性回归
coefficients = np.polyfit(df['x'], df['y'], 1)
slope, intercept = coefficients
打印回归系数
print(f"Slope: {slope}, Intercept: {intercept}")
绘制数据点和回归直线
plt.scatter(df['x'], df['y'], color='red', label='Data Points')
plt.plot(df['x'], slope * df['x'] + intercept, label='Fitted Line')
plt.legend()
plt.show()
在上面的代码中,我们首先创建一个包含数据的Pandas DataFrame,然后使用Numpy的polyfit
函数进行线性回归。最后,我们使用Matplotlib绘制数据点和回归直线。
五、使用Sklearn库进行回归分析
Sklearn库是Python中一个广泛使用的机器学习库,提供了丰富的回归分析工具。我们可以使用Sklearn库中的线性回归模型进行回归分析。
示例代码:
import numpy as np
import matplotlib.pyplot as plt
from sklearn.linear_model import LinearRegression
生成模拟数据
x = np.array([0, 1, 2, 3, 4, 5]).reshape(-1, 1)
y = np.array([2, 3, 5, 7, 11, 14])
创建线性回归模型
model = LinearRegression()
model.fit(x, y)
打印回归系数
print(f"Slope: {model.coef_[0]}, Intercept: {model.intercept_}")
绘制数据点和回归直线
plt.scatter(x, y, color='red', label='Data Points')
plt.plot(x, model.predict(x), label='Fitted Line')
plt.legend()
plt.show()
在上面的代码中,我们使用Sklearn的LinearRegression
类进行线性回归。我们首先将数据转换为二维数组,然后创建并训练线性回归模型。最后,我们使用Matplotlib绘制数据点和回归直线。
六、使用TensorFlow进行回归分析
TensorFlow是一个开源的机器学习框架,广泛用于深度学习和机器学习应用。我们可以使用TensorFlow进行回归分析,特别是在需要处理复杂模型时。
示例代码:
import numpy as np
import tensorflow as tf
import matplotlib.pyplot as plt
生成模拟数据
x = np.array([0, 1, 2, 3, 4, 5], dtype=np.float32)
y = np.array([2, 3, 5, 7, 11, 14], dtype=np.float32)
创建变量
slope = tf.Variable(0.0, dtype=tf.float32)
intercept = tf.Variable(0.0, dtype=tf.float32)
定义模型
def linear_model(x):
return slope * x + intercept
定义损失函数
def loss_fn(y_true, y_pred):
return tf.reduce_mean(tf.square(y_true - y_pred))
定义优化器
optimizer = tf.optimizers.SGD(learning_rate=0.01)
训练模型
for epoch in range(1000):
with tf.GradientTape() as tape:
y_pred = linear_model(x)
loss = loss_fn(y, y_pred)
gradients = tape.gradient(loss, [slope, intercept])
optimizer.apply_gradients(zip(gradients, [slope, intercept]))
打印回归系数
print(f"Slope: {slope.numpy()}, Intercept: {intercept.numpy()}")
绘制数据点和回归直线
plt.scatter(x, y, color='red', label='Data Points')
plt.plot(x, linear_model(x), label='Fitted Line')
plt.legend()
plt.show()
在上面的代码中,我们使用TensorFlow创建变量和模型,并定义损失函数和优化器。通过训练模型,我们可以得到回归系数,并使用Matplotlib绘制数据点和回归直线。
七、使用Pytorch进行回归分析
Pytorch是另一个流行的深度学习框架,提供了灵活的计算图和自动微分功能。我们可以使用Pytorch进行回归分析。
示例代码:
import torch
import numpy as np
import matplotlib.pyplot as plt
生成模拟数据
x = torch.tensor([0, 1, 2, 3, 4, 5], dtype=torch.float32).reshape(-1, 1)
y = torch.tensor([2, 3, 5, 7, 11, 14], dtype=torch.float32).reshape(-1, 1)
创建线性回归模型
model = torch.nn.Linear(1, 1)
定义损失函数和优化器
criterion = torch.nn.MSELoss()
optimizer = torch.optim.SGD(model.parameters(), lr=0.01)
训练模型
for epoch in range(1000):
optimizer.zero_grad()
outputs = model(x)
loss = criterion(outputs, y)
loss.backward()
optimizer.step()
打印回归系数
slope = model.weight.item()
intercept = model.bias.item()
print(f"Slope: {slope}, Intercept: {intercept}")
绘制数据点和回归直线
plt.scatter(x.numpy(), y.numpy(), color='red', label='Data Points')
plt.plot(x.numpy(), model(x).detach().numpy(), label='Fitted Line')
plt.legend()
plt.show()
在上面的代码中,我们使用Pytorch创建线性回归模型,并定义损失函数和优化器。通过训练模型,我们可以得到回归系数,并使用Matplotlib绘制数据点和回归直线。
八、总结
在本文中,我们介绍了多种在Python中求最小二乘法的方法,包括使用Numpy、Scipy、Statsmodels、Pandas、Sklearn、TensorFlow和Pytorch。每种方法都有其优缺点,选择合适的方法取决于具体的需求和应用场景。
使用Numpy进行线性回归适用于简单的线性回归分析,Scipy库中的curve_fit函数适用于非线性最小二乘法拟合,Statsmodels库提供了详细的回归分析功能,Pandas和Numpy结合使用可以方便地进行数据处理和回归分析,Sklearn库提供了丰富的机器学习工具,TensorFlow和Pytorch适用于复杂的模型和深度学习应用。
希望本文能帮助读者了解和掌握在Python中求最小二乘法的方法,并应用于实际问题中。
相关问答FAQs:
如何使用Python进行最小二乘法拟合?
在Python中,可以使用NumPy库和SciPy库来进行最小二乘法拟合。利用NumPy的polyfit()
函数可以轻松实现线性或多项式拟合,同时,SciPy的curve_fit()
函数则适用于更复杂的非线性拟合。具体步骤包括准备数据、选择合适的模型、调用相关函数并分析结果。
最小二乘法的应用场景有哪些?
最小二乘法广泛应用于数据科学、工程和经济学等多个领域。例如,在统计学中,它常用于回归分析,以预测未来的趋势;在工程领域,用于优化设计参数;在机器学习中,作为一种损失函数,帮助调整模型参数以提高预测准确性。
如何评估最小二乘法的拟合效果?
评估拟合效果可以通过多个指标进行,例如决定系数R²、均方误差(MSE)和残差分析。R²值可以用来衡量模型对数据变异的解释能力,而均方误差则反映了预测值与实际值的偏差。此外,通过绘制残差图可以直观地观察拟合的好坏,理想情况下残差应随机分布。
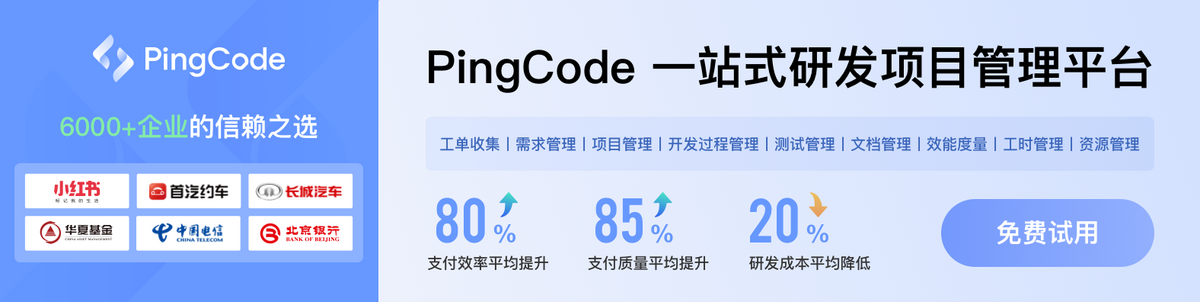