使用Python显示网络图片大小的方法包括请求库、Pillow库、通过获取HTTP头部信息。下面将详细描述其中一种方法。
要详细描述的方法是通过Python的requests库来下载图片,再结合Pillow库来获取图片的大小。首先,我们需要安装这两个库:
pip install requests pillow
使用requests库和Pillow库显示网络图片大小
一、安装必要的Python库
在开始之前,需要确保安装了requests和Pillow库。可以使用pip命令进行安装:
pip install requests pillow
二、使用requests库获取图片数据
首先,使用requests库来下载网络图片的数据。requests库是一个用于发送HTTP请求的库,它非常简单和易用。
import requests
url = 'https://example.com/path/to/image.jpg'
response = requests.get(url)
if response.status_code == 200:
image_data = response.content
else:
print("Failed to retrieve the image.")
在上面的代码中,我们使用requests.get()函数来发送HTTP GET请求,并检查请求是否成功(状态码为200)。如果成功,图片数据将存储在response.content中。
三、使用Pillow库获取图片大小
接下来,我们使用Pillow库来打开图片数据,并获取图片的大小(宽度和高度)。
from PIL import Image
from io import BytesIO
image = Image.open(BytesIO(image_data))
width, height = image.size
print(f"The size of the image is {width}x{height} pixels.")
在上面的代码中,BytesIO用于将图片数据转换为类似文件的对象,这样Pillow库的Image.open()函数就可以打开它。image.size返回一个包含宽度和高度的元组。
四、完整代码示例
下面是完整的示例代码,结合上面的步骤:
import requests
from PIL import Image
from io import BytesIO
def get_image_size(url):
# 发送HTTP GET请求
response = requests.get(url)
if response.status_code == 200:
# 获取图片数据
image_data = response.content
# 打开图片并获取大小
image = Image.open(BytesIO(image_data))
width, height = image.size
return width, height
else:
print("Failed to retrieve the image.")
return None
示例URL
url = 'https://example.com/path/to/image.jpg'
size = get_image_size(url)
if size:
print(f"The size of the image is {size[0]}x{size[1]} pixels.")
这段代码定义了一个函数get_image_size(),它接受一个图片URL作为输入,并返回图片的宽度和高度。如果请求失败,它将返回None。
五、其他方法
除了上述方法外,还有一些其他的方法可以获取网络图片的大小,例如通过获取HTTP头部信息。某些服务器会在响应的头部信息中包含Content-Length字段,指示图片的大小(以字节为单位)。不过,这种方法只能获取文件大小,不能获取图片的分辨率。
import requests
url = 'https://example.com/path/to/image.jpg'
response = requests.head(url)
if response.status_code == 200:
file_size = response.headers.get('Content-Length')
print(f"The file size of the image is {file_size} bytes.")
else:
print("Failed to retrieve the image.")
在这段代码中,我们使用requests.head()函数发送HTTP HEAD请求来获取响应头部信息,并从中提取Content-Length字段的值。
总结
Python提供了多种方法来显示网络图片的大小。最常用的方法是使用requests库来下载图片数据,然后使用Pillow库来获取图片的宽度和高度。这种方法简单且有效,适用于大多数情况。通过掌握这些技术,您可以轻松地处理和分析网络图片。
相关问答FAQs:
如何使用Python获取网络图片的尺寸?
可以使用Python的requests
库下载图片,再通过PIL
(Pillow)库来获取图片的尺寸。下面是一个简单的示例:
import requests
from PIL import Image
from io import BytesIO
url = "图片的URL"
response = requests.get(url)
img = Image.open(BytesIO(response.content))
print(img.size) # 输出图片的宽度和高度
这段代码将显示下载的网络图片的宽度和高度。
在Python中如何处理不同格式的网络图片?
Python的Pillow库支持多种图片格式,包括JPEG、PNG、GIF等。在使用Image.open()
方法时,它会自动识别图片格式,并正确加载图像。确保在下载图片时,requests
库可以处理不同的MIME类型,以避免格式兼容性问题。
是否可以直接从网络获取图片大小而不下载?
可以通过HTTP头信息获取图片的大小。使用requests.head()
方法发送HEAD请求,可以返回响应头,其中包含Content-Length
字段,指示文件大小。以下是获取图片大小的示例代码:
import requests
url = "图片的URL"
response = requests.head(url)
size = response.headers.get('Content-Length')
if size:
print(f"图片大小为: {int(size) / 1024} KB")
else:
print("无法获取图片大小")
这种方法不需要下载整个图片,适合快速获取文件大小信息。
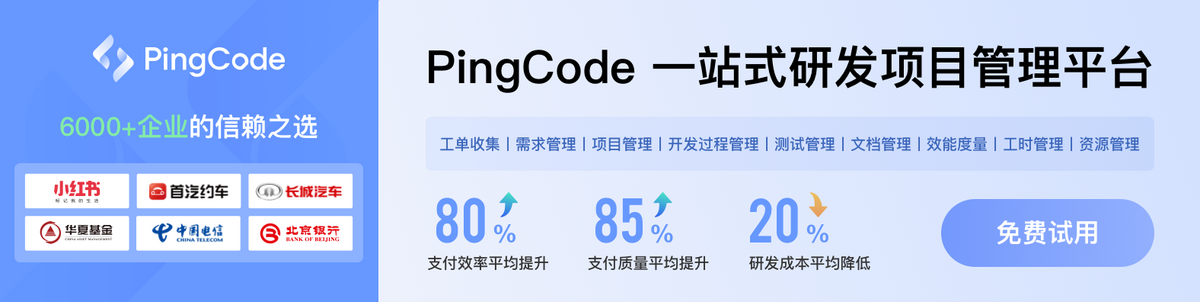