在Python中编写温度转换程序主要包括摄氏度与华氏度的转换、简单易用的函数封装、以及用户交互等要素。通过使用Python编写温度转换程序,可以轻松实现摄氏度与华氏度的互相转换,确保输入输出的准确性,并能提供清晰的用户界面。其中,关键在于理解和应用温度转换公式:摄氏度转华氏度的公式是F = C * 9/5 + 32
,而华氏度转摄氏度的公式是C = (F - 32) * 5/9
。以下是详细的步骤和代码示例。
一、理解温度转换公式
理解温度转换的基本公式是编写转换程序的第一步。这里我们将详细解释这两个公式。
-
摄氏度转华氏度公式
摄氏度(Celsius)转华氏度(Fahrenheit)的公式是:
F = C * 9/5 + 32
。这个公式表示要将摄氏温度乘以9/5,再加上32即可得到华氏温度。这是因为华氏度的刻度设置为每一个华氏度的变化对应摄氏度的5/9。 -
华氏度转摄氏度公式
华氏度转摄氏度的公式是:
C = (F - 32) * 5/9
。该公式表示需要从华氏温度中减去32,再乘以5/9即可得到摄氏温度。这主要是为了抵消之前加上去的32和刻度的换算。
二、编写Python函数
在理解了公式后,我们可以用Python编写简单的函数来实现温度转换。这些函数可以接收一个温度值并返回转换后的结果。
-
摄氏度转华氏度函数
def celsius_to_fahrenheit(celsius):
return celsius * 9/5 + 32
此函数接收一个摄氏度的温度值,使用公式计算并返回对应的华氏度温度。
-
华氏度转摄氏度函数
def fahrenheit_to_celsius(fahrenheit):
return (fahrenheit - 32) * 5/9
此函数接收一个华氏度的温度值,使用公式计算并返回对应的摄氏度温度。
三、用户交互与输入验证
为了使程序更具实用性,我们需要添加用户交互功能。让用户选择他们希望进行的转换类型并输入温度值,同时我们还需要验证用户输入的有效性。
-
用户交互界面
可以使用
input()
函数获取用户输入,并根据用户的选择调用相应的转换函数。def main():
print("Welcome to the Temperature Converter!")
print("1. Convert Celsius to Fahrenheit")
print("2. Convert Fahrenheit to Celsius")
choice = input("Please select a conversion option (1 or 2): ")
if choice == '1':
celsius = float(input("Enter temperature in Celsius: "))
fahrenheit = celsius_to_fahrenheit(celsius)
print(f"{celsius}°C is equal to {fahrenheit}°F")
elif choice == '2':
fahrenheit = float(input("Enter temperature in Fahrenheit: "))
celsius = fahrenheit_to_celsius(fahrenheit)
print(f"{fahrenheit}°F is equal to {celsius}°C")
else:
print("Invalid option. Please enter 1 or 2.")
-
输入验证
在获取用户输入后,确保输入的是有效的数字是非常重要的。可以使用
try-except
块来捕获异常并提示用户重新输入。def get_valid_temperature(prompt):
while True:
try:
return float(input(prompt))
except ValueError:
print("Invalid input. Please enter a numerical value.")
def main():
print("Welcome to the Temperature Converter!")
print("1. Convert Celsius to Fahrenheit")
print("2. Convert Fahrenheit to Celsius")
choice = input("Please select a conversion option (1 or 2): ")
if choice == '1':
celsius = get_valid_temperature("Enter temperature in Celsius: ")
fahrenheit = celsius_to_fahrenheit(celsius)
print(f"{celsius}°C is equal to {fahrenheit}°F")
elif choice == '2':
fahrenheit = get_valid_temperature("Enter temperature in Fahrenheit: ")
celsius = fahrenheit_to_celsius(fahrenheit)
print(f"{fahrenheit}°F is equal to {celsius}°C")
else:
print("Invalid option. Please enter 1 or 2.")
四、扩展功能
为了提高程序的实用性和用户体验,可以考虑增加一些扩展功能。
-
循环执行
可以让程序在转换完成后询问用户是否希望进行另一次转换,从而避免程序终止后需要重新启动。
def main():
while True:
print("Welcome to the Temperature Converter!")
print("1. Convert Celsius to Fahrenheit")
print("2. Convert Fahrenheit to Celsius")
choice = input("Please select a conversion option (1 or 2): ")
if choice == '1':
celsius = get_valid_temperature("Enter temperature in Celsius: ")
fahrenheit = celsius_to_fahrenheit(celsius)
print(f"{celsius}°C is equal to {fahrenheit}°F")
elif choice == '2':
fahrenheit = get_valid_temperature("Enter temperature in Fahrenheit: ")
celsius = fahrenheit_to_celsius(fahrenheit)
print(f"{fahrenheit}°F is equal to {celsius}°C")
else:
print("Invalid option. Please enter 1 or 2.")
again = input("Would you like to perform another conversion? (yes/no): ")
if again.lower() != 'yes':
break
-
支持其他温度单位
除了摄氏度和华氏度,还可以扩展支持开尔文(Kelvin)等其他温度单位的转换。开尔文与摄氏度的转换公式为:
K = C + 273.15
。def celsius_to_kelvin(celsius):
return celsius + 273.15
def kelvin_to_celsius(kelvin):
return kelvin - 273.15
然后在用户交互部分添加新的选项。
def main():
while True:
print("Welcome to the Temperature Converter!")
print("1. Convert Celsius to Fahrenheit")
print("2. Convert Fahrenheit to Celsius")
print("3. Convert Celsius to Kelvin")
print("4. Convert Kelvin to Celsius")
choice = input("Please select a conversion option (1-4): ")
if choice == '1':
celsius = get_valid_temperature("Enter temperature in Celsius: ")
fahrenheit = celsius_to_fahrenheit(celsius)
print(f"{celsius}°C is equal to {fahrenheit}°F")
elif choice == '2':
fahrenheit = get_valid_temperature("Enter temperature in Fahrenheit: ")
celsius = fahrenheit_to_celsius(fahrenheit)
print(f"{fahrenheit}°F is equal to {celsius}°C")
elif choice == '3':
celsius = get_valid_temperature("Enter temperature in Celsius: ")
kelvin = celsius_to_kelvin(celsius)
print(f"{celsius}°C is equal to {kelvin}K")
elif choice == '4':
kelvin = get_valid_temperature("Enter temperature in Kelvin: ")
celsius = kelvin_to_celsius(kelvin)
print(f"{kelvin}K is equal to {celsius}°C")
else:
print("Invalid option. Please enter a number between 1 and 4.")
again = input("Would you like to perform another conversion? (yes/no): ")
if again.lower() != 'yes':
break
五、总结
编写一个温度转换程序是学习Python编程的良好练习,它涉及到基本的数学运算、函数封装、用户交互、输入验证以及程序的扩展性设计。通过这种练习,我们不仅能够巩固Python基础知识,还能提升编写可用程序的能力。希望通过这篇文章,你能对如何在Python中实现温度转换有一个清晰的理解,并能够根据需要进行扩展和优化。
相关问答FAQs:
如何在Python中实现摄氏度与华氏度的转换?
在Python中,摄氏度与华氏度的转换公式为:华氏度 = 摄氏度 × 9/5 + 32。可以创建一个简单的函数来实现这一转换。示例代码如下:
def celsius_to_fahrenheit(celsius):
return celsius * 9/5 + 32
# 示例
temp_c = 25
temp_f = celsius_to_fahrenheit(temp_c)
print(f"{temp_c}°C is equal to {temp_f}°F")
如何处理用户输入以进行温度转换?
可以通过 input()
函数获取用户输入,并在转换之前进行数据验证。确保用户输入的是有效的数字。以下是一个基本示例:
def convert_temperature():
temp = input("请输入要转换的温度(带单位,例如 25C 或 77F):")
if temp[-1].upper() == 'C':
celsius = float(temp[:-1])
print(f"{celsius}°C = {celsius_to_fahrenheit(celsius)}°F")
elif temp[-1].upper() == 'F':
fahrenheit = float(temp[:-1])
print(f"{fahrenheit}°F = {(fahrenheit - 32) * 5/9}°C")
else:
print("无效的输入,请使用 'C' 或 'F' 作为单位。")
convert_temperature()
如何扩展温度转换功能以支持其他单位?
除了摄氏度和华氏度,您还可以添加对开尔文的支持。开尔文与摄氏度的转换公式为:开尔文 = 摄氏度 + 273.15,反之亦然。可以在现有的转换函数中增加额外的逻辑来实现这一点。以下是扩展后的示例:
def celsius_to_kelvin(celsius):
return celsius + 273.15
def fahrenheit_to_celsius(fahrenheit):
return (fahrenheit - 32) * 5/9
def convert_temperature():
temp = input("请输入要转换的温度(带单位,例如 25C 或 77F 或 300K):")
if temp[-1].upper() == 'C':
celsius = float(temp[:-1])
print(f"{celsius}°C = {celsius_to_fahrenheit(celsius)}°F")
print(f"{celsius}°C = {celsius_to_kelvin(celsius)}K")
elif temp[-1].upper() == 'F':
fahrenheit = float(temp[:-1])
celsius = fahrenheit_to_celsius(fahrenheit)
print(f"{fahrenheit}°F = {celsius}°C")
print(f"{fahrenheit}°F = {celsius_to_kelvin(celsius)}K")
elif temp[-1].upper() == 'K':
kelvin = float(temp[:-1])
celsius = kelvin - 273.15
print(f"{kelvin}K = {celsius}°C")
print(f"{kelvin}K = {celsius_to_fahrenheit(celsius)}°F")
else:
print("无效的输入,请使用 'C'、'F' 或 'K' 作为单位。")
convert_temperature()
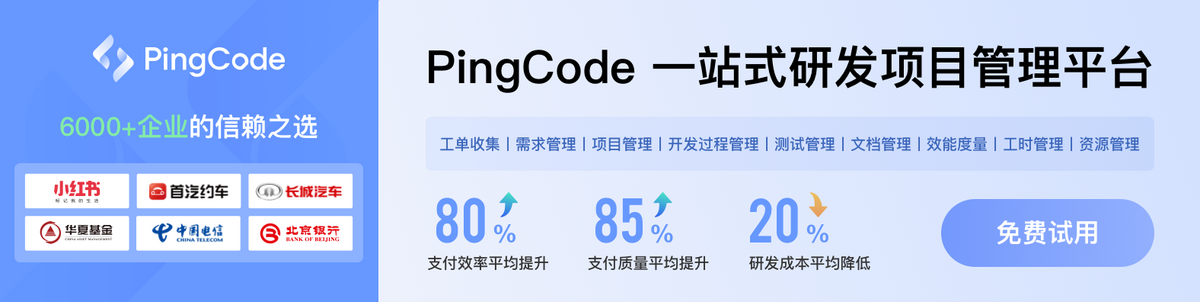