在Python中,返回变量类型可以通过使用内置函数type()
、isinstance()
、__class__
属性来实现。其中,type()
函数是最常用的方法,因为它能够直接返回对象的类型;isinstance()
可以用于检查对象是否是特定类型或其子类的实例;__class__
属性可用于获取对象的类。下面将详细介绍type()
函数的使用方法。
type()
函数是Python的内置函数,用于返回对象的类型。其基本使用方法是将需要检查的变量作为参数传递给type()
函数,然后返回该变量的类型。以下是一个简单的示例:
x = 42
print(type(x)) # 输出:<class 'int'>
在上述示例中,变量x
的类型为整数,因此type(x)
返回<class 'int'>
。type()
函数不仅可以用于基本数据类型,如整数、字符串和列表,还可以用于自定义对象和类的实例,从而广泛适用于各种场景。
一、TYPE()函数的详细使用
type()
函数是Python中用于返回对象类型的基础工具。它的功能不仅仅限于简单的数据类型检查,还可以用于更复杂的类型判断。
1、基本数据类型的使用
对于基本数据类型,type()
函数可以轻松判断变量的类型,包括整数、浮点数、字符串、列表、元组、字典和集合等。
# 整数
num = 10
print(type(num)) # 输出:<class 'int'>
浮点数
flt = 10.5
print(type(flt)) # 输出:<class 'float'>
字符串
text = "Hello, World!"
print(type(text)) # 输出:<class 'str'>
列表
lst = [1, 2, 3]
print(type(lst)) # 输出:<class 'list'>
元组
tup = (1, 2, 3)
print(type(tup)) # 输出:<class 'tuple'>
字典
dct = {"a": 1, "b": 2}
print(type(dct)) # 输出:<class 'dict'>
集合
st = {1, 2, 3}
print(type(st)) # 输出:<class 'set'>
2、检查自定义对象的类型
对于自定义类的实例,可以使用type()
函数来获取对象的类型信息。
class MyClass:
pass
obj = MyClass()
print(type(obj)) # 输出:<class '__main__.MyClass'>
在这个示例中,obj
是MyClass
类的一个实例,通过type(obj)
可以获取到它所属的类。
3、与比较运算结合使用
type()
函数的返回值可以与数据类型进行比较,以实现更复杂的逻辑控制。
def check_type(variable):
if type(variable) == int:
return "This is an integer"
elif type(variable) == str:
return "This is a string"
else:
return "Unknown type"
print(check_type(42)) # 输出:This is an integer
print(check_type("Python")) # 输出:This is a string
二、ISINSTANCE()函数的应用
虽然type()
函数功能强大,但在某些情况下,isinstance()
函数可能更为合适。isinstance()
不仅能判断变量是否属于某个类型,还能判断变量是否属于该类型的子类。
1、基本用法
isinstance()
接收两个参数,第一个是待检查的对象,第二个是类型或类型的元组。它返回布尔值,指示对象是否为指定类型的实例。
# 检查整数
num = 10
print(isinstance(num, int)) # 输出:True
检查字符串
text = "Hello"
print(isinstance(text, str)) # 输出:True
2、检查多个类型
使用类型元组可以同时检查多个类型,isinstance()
只要对象是元组中任意一个类型的实例,就返回True
。
num = 10
print(isinstance(num, (int, float))) # 输出:True
flt = 10.5
print(isinstance(flt, (int, float))) # 输出:True
text = "Hello"
print(isinstance(text, (int, float))) # 输出:False
3、与继承配合使用
isinstance()
特别适合用于检查继承关系,因为它可以识别子类的实例。
class Animal:
pass
class Dog(Animal):
pass
dog = Dog()
print(isinstance(dog, Dog)) # 输出:True
print(isinstance(dog, Animal)) # 输出:True
在这个示例中,dog
是Dog
类的实例,同时也是Animal
类的实例,因为Dog
继承自Animal
。
三、__CLASS__属性的使用
除了type()
和isinstance()
之外,Python对象还有一个__class__
属性,指向对象的类。这在某些情况下可以用于获取对象的类型。
1、基本用法
__class__
属性提供了另一种获取对象类型的方法。
num = 10
print(num.__class__) # 输出:<class 'int'>
2、与自定义类结合使用
对于自定义类的实例,__class__
属性同样有效。
class MyClass:
pass
obj = MyClass()
print(obj.__class__) # 输出:<class '__main__.MyClass'>
四、实际应用场景
在实际应用中,判断变量类型的需求非常普遍,比如在数据处理、类型转换和错误处理等场合,正确地判断变量类型可以提高程序的健壮性和可维护性。
1、数据处理
在处理数据时,我们可能需要根据数据类型选择不同的处理逻辑,比如在数据清洗和格式化过程中。
def process_data(data):
if isinstance(data, list):
return [str(item) for item in data]
elif isinstance(data, dict):
return {key: str(value) for key, value in data.items()}
else:
return str(data)
print(process_data([1, 2, 3])) # 输出:['1', '2', '3']
print(process_data({"a": 1, "b": 2})) # 输出:{'a': '1', 'b': '2'}
2、错误处理
类型检查在错误处理和调试中也发挥着重要作用,确保函数接收到期望的参数类型可以避免许多潜在的问题。
def add_numbers(a, b):
if not (isinstance(a, (int, float)) and isinstance(b, (int, float))):
raise TypeError("Both arguments must be numbers")
return a + b
try:
result = add_numbers(10, "20")
except TypeError as e:
print(e) # 输出:Both arguments must be numbers
3、类型转换
在进行类型转换时,确认变量的当前类型可以防止不必要的转换或错误。
def convert_to_string(value):
if not isinstance(value, str):
return str(value)
return value
print(convert_to_string(123)) # 输出:'123'
print(convert_to_string("abc")) # 输出:'abc'
五、总结
在Python编程中,了解变量的类型是进行有效编码的基础。通过type()
、isinstance()
和__class__
属性,我们可以准确判断变量的类型,从而编写出更健壮和高效的代码。无论是基本数据类型还是自定义类的实例,合理地利用这些工具可以帮助我们在开发过程中更好地管理和处理数据,提高代码的可靠性和可维护性。
相关问答FAQs:
如何在Python中检查变量的类型?
在Python中,可以使用内置的type()
函数来检查变量的类型。只需将变量作为参数传递给type()
,它将返回该变量的类型。例如,type(my_variable)
将返回<class 'int'>
,如果my_variable
是一个整数。
Python中有哪些常用的方法可以判断变量类型?
除了使用type()
函数外,Python还提供了isinstance()
函数,可以用来判断一个变量是否属于某个特定的类型。这种方法特别有用,可以同时检查多个类型。例如,isinstance(my_variable, (int, float))
将检查my_variable
是否是整数或浮点数。
在Python中,如何处理动态类型的变量?
Python是一种动态类型语言,这意味着变量的类型可以在运行时改变。如果需要跟踪变量类型的变化,可以使用type()
函数在不同的时间点检查变量的类型。此外,良好的代码实践包括使用注释和文档字符串来说明变量的预期类型,以提高代码的可读性和可维护性。
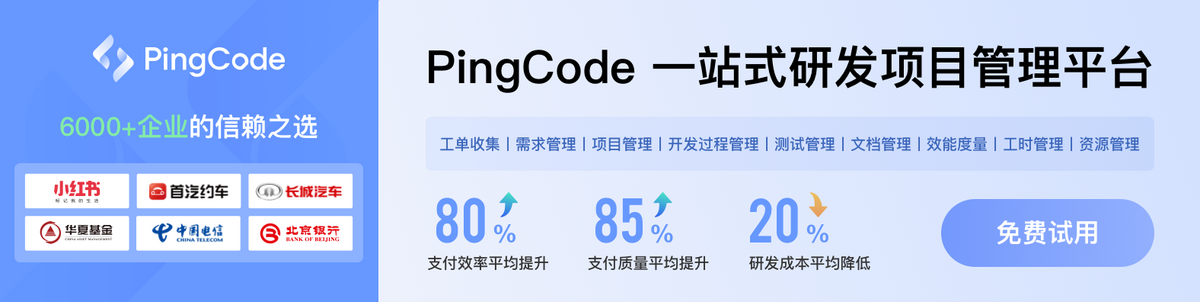