Python如何朋友圈自动点赞:通过Python实现朋友圈自动点赞,可以使用模拟用户操作的方式来完成,如使用自动化测试工具Selenium、使用Appium进行移动端自动化控制、通过微信公众平台接口进行操作。这些方法各有优缺点,具体的选择需要根据实际的需求和环境来决定。本文将详细介绍如何使用Selenium和Appium来实现自动点赞,并对其中的关键点进行详细说明。
一、使用Selenium进行网页自动化操作
Selenium是一款广泛使用的Web应用程序自动化测试工具,它可以在浏览器上执行用户操作,如点击、输入文本等。通过Selenium,我们可以模拟用户在微信网页版上的操作,从而实现自动点赞功能。
1、安装与配置Selenium
首先,需要安装Selenium库和浏览器驱动。以Chrome为例,使用以下命令安装Selenium库:
pip install selenium
接下来,下载ChromeDriver并将其路径添加到系统环境变量中。ChromeDriver可以从以下地址下载:
2、编写自动点赞脚本
下面是一个使用Selenium模拟微信网页版自动点赞的示例代码:
from selenium import webdriver
from selenium.webdriver.common.keys import Keys
import time
初始化Chrome浏览器
driver = webdriver.Chrome()
打开微信网页版
driver.get('https://wx.qq.com/')
等待用户扫描二维码登录
print("请扫描二维码登录微信网页版")
time.sleep(30) # 根据实际情况调整时间
查找朋友圈入口并点击
friend_circle = driver.find_element_by_xpath('//span[@class="icon-sns"]')
friend_circle.click()
time.sleep(5)
自动点赞
def auto_like():
posts = driver.find_elements_by_xpath('//div[@class="msg"]')
for post in posts:
like_button = post.find_element_by_xpath('.//a[@class="like"]')
like_button.click()
time.sleep(1)
执行自动点赞
auto_like()
关闭浏览器
driver.quit()
注意:由于微信网页版的限制,该代码可能无法完全实现自动点赞功能,仅供参考。
二、使用Appium进行移动端自动化操作
Appium是一款用于移动应用程序的自动化测试工具,可以在不修改应用程序的情况下对其进行自动化测试。通过Appium,我们可以模拟用户在微信移动端上的操作,从而实现自动点赞功能。
1、安装与配置Appium
首先,需要安装Appium和Appium-Python-Client。使用以下命令安装:
npm install -g appium
pip install Appium-Python-Client
接下来,启动Appium服务器:
appium
2、编写自动点赞脚本
下面是一个使用Appium模拟微信移动端自动点赞的示例代码:
from appium import webdriver
import time
配置Appium参数
desired_caps = {
'platformName': 'Android',
'deviceName': 'YourDeviceName',
'appPackage': 'com.tencent.mm',
'appActivity': '.ui.LauncherUI',
'noReset': True,
}
初始化Appium驱动
driver = webdriver.Remote('http://localhost:4723/wd/hub', desired_caps)
等待微信启动
time.sleep(10)
进入朋友圈
friend_circle = driver.find_element_by_xpath('//android.widget.TextView[@text="朋友圈"]')
friend_circle.click()
time.sleep(5)
自动点赞
def auto_like():
posts = driver.find_elements_by_xpath('//android.widget.FrameLayout')
for post in posts:
like_button = post.find_element_by_xpath('.//android.widget.ImageView[@content-desc="赞"]')
like_button.click()
time.sleep(1)
执行自动点赞
auto_like()
关闭Appium驱动
driver.quit()
注意:由于微信的反自动化机制,该代码可能无法完全实现自动点赞功能,仅供参考。
三、通过微信公众平台接口进行操作
微信公众平台提供了一些API接口,可以用来实现自动化操作。然而,这些接口主要用于公众号和小程序的开发,对于个人微信账号的操作有一定的限制。
1、申请微信公众平台账号并获取接口权限
首先,需要申请一个微信公众平台账号,并获取相关接口的权限。可以参考微信公众平台的官方文档进行操作:
2、编写调用微信接口的代码
下面是一个使用Python调用微信公众平台接口的示例代码:
import requests
import json
获取access_token
def get_access_token(appid, appsecret):
url = f'https://api.weixin.qq.com/cgi-bin/token?grant_type=client_credential&appid={appid}&secret={appsecret}'
response = requests.get(url)
data = response.json()
return data['access_token']
获取用户列表
def get_user_list(access_token):
url = f'https://api.weixin.qq.com/cgi-bin/user/get?access_token={access_token}'
response = requests.get(url)
data = response.json()
return data['data']['openid']
发送模板消息
def send_template_message(access_token, openid, template_id, data):
url = f'https://api.weixin.qq.com/cgi-bin/message/template/send?access_token={access_token}'
payload = {
'touser': openid,
'template_id': template_id,
'data': data
}
response = requests.post(url, data=json.dumps(payload))
return response.json()
示例代码
appid = 'your_appid'
appsecret = 'your_appsecret'
access_token = get_access_token(appid, appsecret)
user_list = get_user_list(access_token)
template_id = 'your_template_id'
data = {
'first': {'value': 'Hello!'},
'remark': {'value': 'This is a test message.'}
}
发送模板消息给所有用户
for openid in user_list:
result = send_template_message(access_token, openid, template_id, data)
print(result)
四、总结
通过本文的介绍,我们了解了使用Selenium、Appium和微信公众平台接口实现朋友圈自动点赞的几种方法。每种方法都有其优缺点,需要根据实际需求进行选择。需要注意的是,微信平台对自动化操作有一定的限制和反制机制,因此在使用这些方法时需要谨慎,避免账号被封禁的风险。
重点总结:
- Selenium:适用于网页自动化操作,但微信网页版限制较多。
- Appium:适用于移动端自动化操作,但需要配置复杂的环境。
- 微信公众平台接口:适用于公众号和小程序开发,但对个人微信账号的操作有限。
希望本文能对您实现朋友圈自动点赞有所帮助。如果有任何问题或建议,欢迎在评论区留言交流。
相关问答FAQs:
如何使用Python实现朋友圈自动点赞功能?
要实现朋友圈自动点赞功能,您需要使用一些爬虫和自动化工具。常用的库有Selenium和Requests。Selenium可以模拟用户行为,而Requests可以用于发送HTTP请求。您需要先获取登录信息和点赞的目标链接,然后编写相应的代码进行操作。
实现自动点赞需要了解哪些基本知识?
在开始实现自动点赞之前,了解Python编程、网络请求的基本原理、以及如何使用Selenium和BeautifulSoup等库是非常重要的。这些知识将帮助您更好地理解如何与网页进行交互,以及如何解析网页内容。
使用自动点赞功能时需要注意哪些法律和道德问题?
使用自动点赞功能可能会违反社交平台的使用协议,因此在使用此功能时要特别小心。社交平台通常会对使用机器人行为的账户进行封禁,建议在实施之前详细阅读相关条款。此外,考虑到网络安全和隐私问题,确保不侵犯他人的权益也是非常重要的。
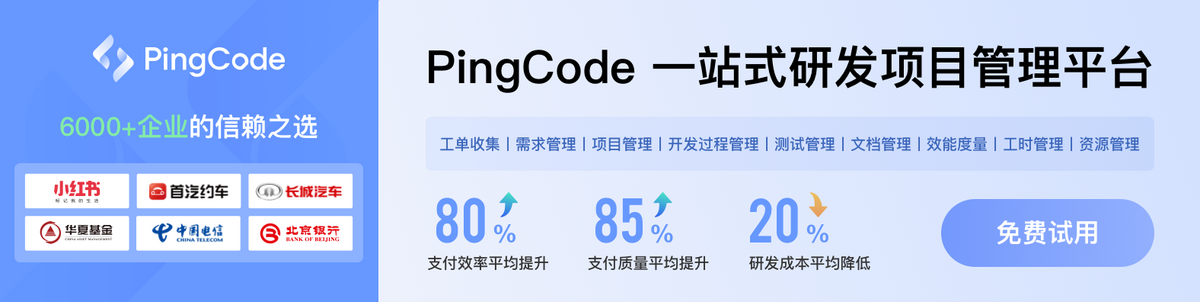