要下载音频文件夹,使用Python可以通过以下几种方式:使用requests库、使用BeautifulSoup库进行网页解析、使用os库管理文件目录。 其中,使用requests库下载音频文件是比较常用的方法,下面将详细描述这种方式。
一、使用requests库下载音频文件
requests库是Python中一个简单易用的HTTP库,它可以帮助我们轻松地发送HTTP请求。下面是一个简单的示例,展示如何使用requests库下载音频文件:
import requests
url = 'https://example.com/audiofile.mp3'
response = requests.get(url)
with open('audiofile.mp3', 'wb') as file:
file.write(response.content)
这种方法适用于单个音频文件的下载,如果需要下载整个音频文件夹,还需要额外的步骤,例如解析网页获取所有音频文件的链接。下面详细介绍如何下载整个音频文件夹。
二、解析网页获取所有音频文件链接
要下载整个音频文件夹,首先需要获取该文件夹中所有音频文件的链接。可以使用BeautifulSoup库来解析网页,提取所有音频文件的链接。
import requests
from bs4 import BeautifulSoup
url = 'https://example.com/audiofolder/'
response = requests.get(url)
soup = BeautifulSoup(response.content, 'html.parser')
audio_links = []
for link in soup.find_all('a'):
href = link.get('href')
if href.endswith('.mp3'): # Assuming audio files are in .mp3 format
audio_links.append(url + href)
print(audio_links)
三、下载解析出的音频文件
获取所有音频文件的链接后,可以循环这些链接并下载每个音频文件。
import os
Create a directory to save the audio files
os.makedirs('audio_folder', exist_ok=True)
for link in audio_links:
response = requests.get(link)
filename = os.path.join('audio_folder', link.split('/')[-1])
with open(filename, 'wb') as file:
file.write(response.content)
print(f'Downloaded {filename}')
四、处理大文件和断点续传
有时候,音频文件可能会比较大,下载过程中可能会出现网络中断等情况。可以使用断点续传来解决这个问题。以下是一个实现断点续传下载的示例:
import os
import requests
def download_file(url, folder):
local_filename = os.path.join(folder, url.split('/')[-1])
headers = {}
if os.path.exists(local_filename):
headers['Range'] = f"bytes={os.path.getsize(local_filename)}-"
response = requests.get(url, headers=headers, stream=True)
with open(local_filename, 'ab') as file:
for chunk in response.iter_content(chunk_size=8192):
if chunk:
file.write(chunk)
return local_filename
Create a directory to save the audio files
os.makedirs('audio_folder', exist_ok=True)
for link in audio_links:
filename = download_file(link, 'audio_folder')
print(f'Downloaded {filename}')
五、使用多线程加速下载
为了加快下载速度,可以使用多线程或多进程并发下载音频文件。下面是一个使用多线程加速下载的示例:
import os
import requests
from concurrent.futures import ThreadPoolExecutor
def download_file(url, folder):
local_filename = os.path.join(folder, url.split('/')[-1])
headers = {}
if os.path.exists(local_filename):
headers['Range'] = f"bytes={os.path.getsize(local_filename)}-"
response = requests.get(url, headers=headers, stream=True)
with open(local_filename, 'ab') as file:
for chunk in response.iter_content(chunk_size=8192):
if chunk:
file.write(chunk)
return local_filename
Create a directory to save the audio files
os.makedirs('audio_folder', exist_ok=True)
with ThreadPoolExecutor(max_workers=4) as executor:
futures = [executor.submit(download_file, link, 'audio_folder') for link in audio_links]
for future in futures:
filename = future.result()
print(f'Downloaded {filename}')
六、总结
通过上述步骤,可以使用Python下载整个音频文件夹。首先,需要使用requests库和BeautifulSoup库解析网页,获取所有音频文件的链接。然后,循环这些链接下载每个音频文件。为了提高下载效率,可以使用多线程或多进程并发下载。最后,为了应对大文件下载中的网络中断问题,可以实现断点续传功能。通过合理使用这些技术,可以高效地下载整个音频文件夹。
相关问答FAQs:
如何使用Python下载音频文件夹中的多个音频文件?
要下载音频文件夹中的多个音频文件,可以使用Python的requests库来处理HTTP请求,并结合os库创建本地目录。可以遍历文件夹中的音频文件链接并逐一下载。确保使用适当的文件扩展名和保存路径,以便于后续管理。
在下载音频文件时,如何处理不同格式的文件?
在下载音频文件时,应注意文件格式的兼容性。Python支持多种音频格式,如MP3、WAV等。根据文件的MIME类型,您可以选择使用不同的库来处理这些文件。例如,使用pydub库可以帮助您转换和处理音频文件格式,以确保下载后能够顺利播放。
下载音频文件夹时,如何提高下载速度?
提高下载速度的方法包括使用多线程或异步编程。使用Python的concurrent.futures模块可以轻松实现多线程下载,允许同时下载多个音频文件。此外,使用异步库如aiohttp可以有效地处理多个请求,从而提高整体下载效率。确保网络连接稳定也是影响下载速度的重要因素。
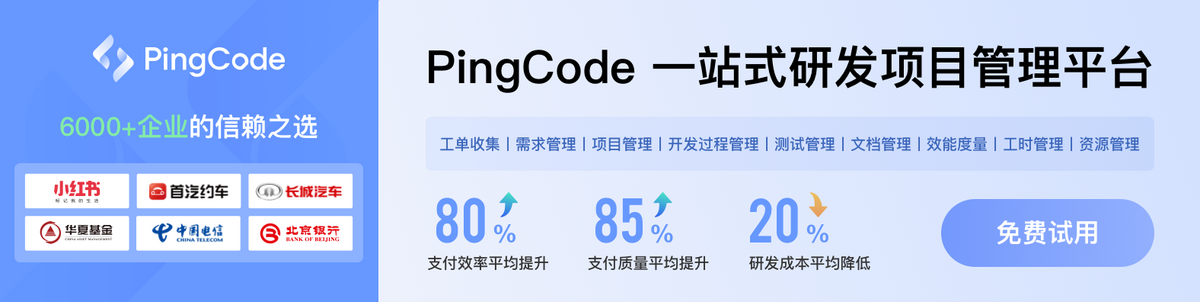