Python调用文件夹内图片的方法有多种,可以使用不同的库和方法来实现。以下是一些常见的方法:使用os库遍历文件夹、使用PIL库读取图片、使用OpenCV库读取图片。这里将详细介绍如何使用这些方法来实现读取文件夹内的图片,并给出具体的代码示例。
一、使用os库遍历文件夹
os库是Python标准库中的一个模块,可以用来处理文件和目录的操作。我们可以使用os.listdir()函数遍历文件夹中的所有文件,并使用os.path.join()函数构建文件的完整路径。
1. 遍历文件夹中的文件
import os
def list_images(directory):
images = []
for filename in os.listdir(directory):
if filename.endswith(".jpg") or filename.endswith(".png"):
images.append(os.path.join(directory, filename))
return images
image_directory = "path/to/your/image/folder"
images = list_images(image_directory)
print(images)
二、使用PIL库读取图片
PIL(Python Imaging Library)是一个强大的图像处理库,后来被Pillow库所取代。我们可以使用Pillow库来读取和处理图片。首先,确保已安装Pillow库,可以使用以下命令安装:
pip install pillow
1. 读取图片并显示
from PIL import Image
import os
def load_images(directory):
images = []
for filename in os.listdir(directory):
if filename.endswith(".jpg") or filename.endswith(".png"):
img_path = os.path.join(directory, filename)
img = Image.open(img_path)
images.append(img)
return images
image_directory = "path/to/your/image/folder"
images = load_images(image_directory)
for img in images:
img.show()
2. 读取图片并处理
from PIL import Image
import os
def process_images(directory):
for filename in os.listdir(directory):
if filename.endswith(".jpg") or filename.endswith(".png"):
img_path = os.path.join(directory, filename)
img = Image.open(img_path)
# Perform some image processing, e.g., resize
img = img.resize((100, 100))
img.save(os.path.join(directory, "processed_" + filename))
image_directory = "path/to/your/image/folder"
process_images(image_directory)
三、使用OpenCV库读取图片
OpenCV是一个开源计算机视觉库,具有强大的图像处理功能。我们可以使用OpenCV库来读取和处理图片。首先,确保已安装OpenCV库,可以使用以下命令安装:
pip install opencv-python
1. 读取图片并显示
import cv2
import os
def load_images_cv(directory):
images = []
for filename in os.listdir(directory):
if filename.endswith(".jpg") or filename.endswith(".png"):
img_path = os.path.join(directory, filename)
img = cv2.imread(img_path)
images.append(img)
return images
image_directory = "path/to/your/image/folder"
images = load_images_cv(image_directory)
for img in images:
cv2.imshow('Image', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
2. 读取图片并处理
import cv2
import os
def process_images_cv(directory):
for filename in os.listdir(directory):
if filename.endswith(".jpg") or filename.endswith(".png"):
img_path = os.path.join(directory, filename)
img = cv2.imread(img_path)
# Perform some image processing, e.g., convert to grayscale
gray_img = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
cv2.imwrite(os.path.join(directory, "processed_" + filename), gray_img)
image_directory = "path/to/your/image/folder"
process_images_cv(image_directory)
四、使用glob库查找文件
glob库是Python标准库中的一个模块,用于查找符合特定模式的文件。我们可以使用glob库来查找文件夹中的所有图片文件。
1. 查找图片文件
import glob
import os
def list_images_glob(directory):
image_files = glob.glob(os.path.join(directory, "*.jpg")) + glob.glob(os.path.join(directory, "*.png"))
return image_files
image_directory = "path/to/your/image/folder"
images = list_images_glob(image_directory)
print(images)
五、结合多种方法
在实际应用中,我们可以结合多种方法来实现更复杂的功能。例如,使用os库遍历文件夹,使用PIL库读取图片,并进行处理后保存。
1. 结合os和PIL库
from PIL import Image
import os
def process_images_combined(directory):
for filename in os.listdir(directory):
if filename.endswith(".jpg") or filename.endswith(".png"):
img_path = os.path.join(directory, filename)
img = Image.open(img_path)
# Perform some image processing, e.g., rotate
img = img.rotate(90)
img.save(os.path.join(directory, "processed_" + filename))
image_directory = "path/to/your/image/folder"
process_images_combined(image_directory)
以上是Python调用文件夹内图片的几种常见方法。根据具体需求,可以选择合适的方法来实现图片的读取和处理。希望这些示例代码能对你有所帮助。
相关问答FAQs:
如何在Python中读取文件夹内的所有图片?
在Python中,可以使用os
库和PIL
(Pillow)库来读取文件夹内的所有图片。首先,使用os.listdir()
获取文件夹内的所有文件名,然后通过循环遍历这些文件,使用PIL.Image.open()
打开并处理图片。以下是一个简单的示例代码:
import os
from PIL import Image
folder_path = 'your_folder_path' # 替换为你的文件夹路径
for file_name in os.listdir(folder_path):
if file_name.endswith(('.png', '.jpg', '.jpeg', '.gif')): # 根据需要添加更多格式
img_path = os.path.join(folder_path, file_name)
img = Image.open(img_path)
img.show() # 显示图片
在Python中如何批量处理文件夹中的图片?
批量处理文件夹中的图片可以通过遍历文件夹内的所有图片文件,并对每张图片进行相应的操作。常见的操作包括调整大小、转换格式、应用滤镜等。使用PIL
库,可以轻松实现这些功能。例如,以下代码将所有图片调整为指定的大小:
from PIL import Image
import os
folder_path = 'your_folder_path' # 替换为你的文件夹路径
output_folder = 'output_folder' # 输出文件夹路径
if not os.path.exists(output_folder):
os.makedirs(output_folder)
for file_name in os.listdir(folder_path):
if file_name.endswith(('.png', '.jpg', '.jpeg', '.gif')):
img_path = os.path.join(folder_path, file_name)
img = Image.open(img_path)
img_resized = img.resize((100, 100)) # 调整为100x100大小
img_resized.save(os.path.join(output_folder, file_name))
使用Python如何显示文件夹中的图片?
可以使用matplotlib
库来显示文件夹中的图片。通过循环读取文件夹中的每一张图片,并使用plt.imshow()
函数显示。以下是相关示例代码:
import os
import matplotlib.pyplot as plt
from PIL import Image
folder_path = 'your_folder_path' # 替换为你的文件夹路径
for file_name in os.listdir(folder_path):
if file_name.endswith(('.png', '.jpg', '.jpeg', '.gif')):
img_path = os.path.join(folder_path, file_name)
img = Image.open(img_path)
plt.imshow(img)
plt.axis('off') # 不显示坐标轴
plt.show() # 显示图片
这些方法和代码片段可以帮助您在Python中高效地调用和处理文件夹内的图片。
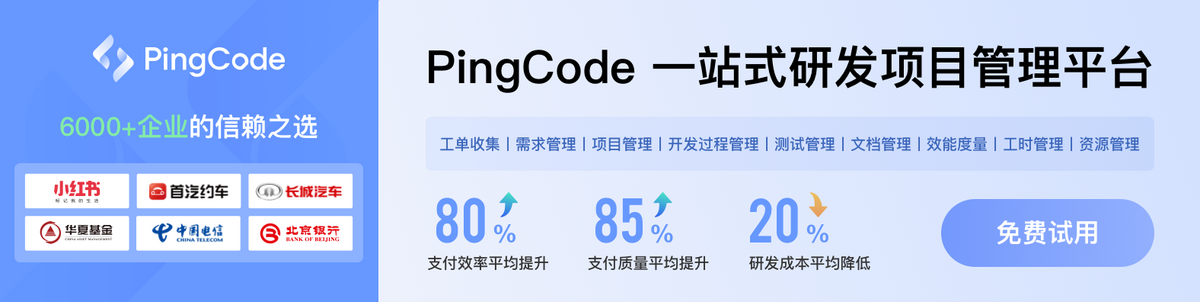