用Python画一个老鼠的几种方法包括使用Turtle库、Pygame库和Matplotlib库等、每种方法都有其优缺点、推荐使用Turtle库因为它简单易用。
Turtle库是Python内置的图形库,特别适合初学者使用。它提供了一个简单的方式来绘制图形,甚至可以用来绘制一些复杂的图案,比如老鼠。以下将详细介绍如何用Turtle库画一个老鼠。
一、安装和设置
首先,确保你已经安装了Python。如果没有,可以从Python的官方网站下载并安装最新版本。Turtle库是Python的标准库之一,所以不需要单独安装。
import turtle
二、绘制老鼠的身体
老鼠的身体是最主要的部分,可以使用椭圆形或圆形来表示。以下是如何用Turtle库绘制一个老鼠的身体。
def draw_body():
turtle.penup()
turtle.goto(0, -50)
turtle.pendown()
turtle.begin_fill()
turtle.color("gray")
turtle.circle(100)
turtle.end_fill()
三、绘制老鼠的耳朵
老鼠的耳朵可以用两个小圆形来表示,放置在身体的顶部。
def draw_ears():
turtle.penup()
turtle.goto(-35, 120)
turtle.pendown()
turtle.begin_fill()
turtle.color("gray")
turtle.circle(30)
turtle.end_fill()
turtle.penup()
turtle.goto(35, 120)
turtle.pendown()
turtle.begin_fill()
turtle.color("gray")
turtle.circle(30)
turtle.end_fill()
四、绘制老鼠的眼睛
老鼠的眼睛可以用两个小小的圆形来表示,放置在耳朵下方。
def draw_eyes():
turtle.penup()
turtle.goto(-20, 80)
turtle.pendown()
turtle.begin_fill()
turtle.color("white")
turtle.circle(10)
turtle.end_fill()
turtle.penup()
turtle.goto(20, 80)
turtle.pendown()
turtle.begin_fill()
turtle.color("white")
turtle.circle(10)
turtle.end_fill()
turtle.penup()
turtle.goto(-20, 85)
turtle.pendown()
turtle.begin_fill()
turtle.color("black")
turtle.circle(5)
turtle.end_fill()
turtle.penup()
turtle.goto(20, 85)
turtle.pendown()
turtle.begin_fill()
turtle.color("black")
turtle.circle(5)
turtle.end_fill()
五、绘制老鼠的鼻子和胡须
老鼠的鼻子可以用一个小圆形来表示,胡须可以用直线来表示。
def draw_nose_and_whiskers():
turtle.penup()
turtle.goto(0, 60)
turtle.pendown()
turtle.begin_fill()
turtle.color("pink")
turtle.circle(10)
turtle.end_fill()
turtle.penup()
turtle.goto(-15, 55)
turtle.pendown()
turtle.color("black")
turtle.right(30)
for _ in range(3):
turtle.forward(30)
turtle.backward(30)
turtle.left(15)
turtle.penup()
turtle.goto(15, 55)
turtle.pendown()
turtle.right(45)
for _ in range(3):
turtle.forward(30)
turtle.backward(30)
turtle.left(15)
六、绘制老鼠的尾巴
老鼠的尾巴可以用一条长长的曲线来表示。
def draw_tail():
turtle.penup()
turtle.goto(0, -100)
turtle.pendown()
turtle.color("gray")
turtle.right(60)
turtle.circle(80, 120)
七、整合所有部分
最后,我们需要将所有部分整合在一起,形成一个完整的老鼠图案。
def draw_mouse():
draw_body()
draw_ears()
draw_eyes()
draw_nose_and_whiskers()
draw_tail()
turtle.hideturtle()
turtle.done()
draw_mouse()
总结
通过以上步骤,我们使用Python的Turtle库绘制了一个简单的老鼠图案。Turtle库非常适合用来绘制各种图形,不仅限于老鼠。通过不断练习和尝试,你可以绘制出更加复杂和精美的图案。
希望这篇文章能帮助你更好地理解如何用Python画一个老鼠。如果你有其他问题或需要进一步的指导,请随时向我提问。
相关问答FAQs:
如何使用Python绘制老鼠的基本步骤是什么?
要使用Python绘制老鼠,您可以利用图形库,如turtle
或matplotlib
。首先,确保安装了相关库。然后,创建一个新文件并导入库。在绘图的过程中,可以逐步定义老鼠的各个部分,比如头、耳朵、身体和尾巴,通过设置不同的颜色和形状来使老鼠更加生动。
Python中有哪些库适合绘制图形?
除了turtle
和matplotlib
,您还可以使用pygame
和PIL
(Python Imaging Library)进行图形绘制。pygame
适合更复杂的游戏图形和动画,而PIL
则适合图像处理和简单的绘图需求。选择合适的库可以让您更方便地实现想要的效果。
在绘制老鼠时,如何处理颜色和形状的搭配?
在绘制过程中,选择合适的颜色和形状对于表现老鼠的可爱形象至关重要。可以使用fillcolor()
函数设置填充颜色,同时使用begin_fill()
和end_fill()
来填充形状。建议使用柔和的颜色,如灰色、白色或棕色,再通过细节如眼睛和胡须增加趣味性,这样可以让绘制的老鼠更加生动可爱。
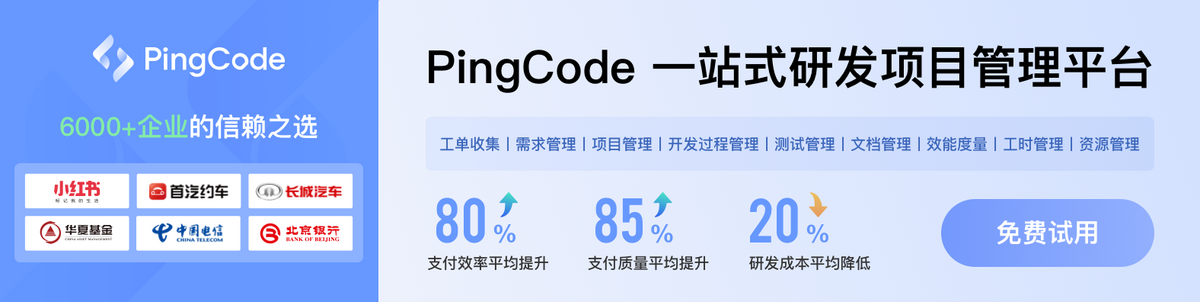