如何使用Python抓取彼岸桌面图片
使用Python抓取彼岸桌面图片的方法包括:使用requests库下载网页、使用BeautifulSoup解析HTML、提取图片URL、下载图片、处理反爬虫机制。首先,我们会用requests库下载彼岸桌面的网站HTML内容,然后用BeautifulSoup解析HTML来提取图片的URL,接着使用requests库下载图片,并应对网站可能的反爬虫机制。接下来,我们将详细说明每一步的操作。
一、使用requests库下载网页
使用Python的requests库可以轻松下载网页内容。requests库是一个简单而强大的HTTP库,用于发送HTTP请求并获取响应。
import requests
目标URL
url = 'http://www.netbian.com/index.htm'
发送HTTP请求
response = requests.get(url)
检查请求是否成功
if response.status_code == 200:
html_content = response.text
else:
print('Failed to retrieve the webpage.')
二、使用BeautifulSoup解析HTML
BeautifulSoup是一个用于解析HTML和XML文档的库。它可以帮助我们轻松地提取所需的信息。
from bs4 import BeautifulSoup
解析HTML
soup = BeautifulSoup(html_content, 'html.parser')
查找所有图片标签
image_tags = soup.find_all('img')
提取图片URL
image_urls = [img['src'] for img in image_tags if 'src' in img.attrs]
三、提取图片URL
在彼岸桌面网站中,图片的URL通常嵌在img
标签的src
属性中。我们可以通过BeautifulSoup轻松提取这些URL。
# 提取图片URL
image_urls = []
for img in image_tags:
src = img.get('src')
if src and src.startswith('http'):
image_urls.append(src)
四、下载图片
使用requests库下载图片并保存到本地。
import os
创建保存图片的目录
os.makedirs('images', exist_ok=True)
下载并保存图片
for url in image_urls:
image_name = os.path.basename(url)
image_path = os.path.join('images', image_name)
# 发送请求下载图片
image_response = requests.get(url)
if image_response.status_code == 200:
with open(image_path, 'wb') as file:
file.write(image_response.content)
else:
print(f'Failed to download {url}')
五、处理反爬虫机制
网站通常会采用一些反爬虫机制,比如限制请求频率、使用验证码等。以下是一些常见的应对方法:
1. 设置请求头:
通过设置User-Agent等请求头,可以模拟浏览器请求,避免被识别为爬虫。
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/91.0.4472.124 Safari/537.36'
}
response = requests.get(url, headers=headers)
2. 添加延迟:
添加随机延迟,避免频繁请求被封禁。
import time
import random
for url in image_urls:
time.sleep(random.uniform(1, 3)) # 随机延迟1到3秒
# 下载图片的代码
3. 使用代理:
通过代理服务器发送请求,可以隐藏真实的IP地址。
proxies = {
'http': 'http://your_proxy_ip:port',
'https': 'http://your_proxy_ip:port'
}
response = requests.get(url, headers=headers, proxies=proxies)
六、完整代码
以下是完整的Python脚本,用于抓取彼岸桌面图片。
import os
import time
import random
import requests
from bs4 import BeautifulSoup
目标URL
url = 'http://www.netbian.com/index.htm'
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/91.0.4472.124 Safari/537.36'
}
发送HTTP请求
response = requests.get(url, headers=headers)
if response.status_code == 200:
html_content = response.text
else:
print('Failed to retrieve the webpage.')
exit()
解析HTML
soup = BeautifulSoup(html_content, 'html.parser')
image_tags = soup.find_all('img')
提取图片URL
image_urls = []
for img in image_tags:
src = img.get('src')
if src and src.startswith('http'):
image_urls.append(src)
创建保存图片的目录
os.makedirs('images', exist_ok=True)
下载并保存图片
for url in image_urls:
time.sleep(random.uniform(1, 3)) # 随机延迟1到3秒
image_name = os.path.basename(url)
image_path = os.path.join('images', image_name)
# 发送请求下载图片
image_response = requests.get(url, headers=headers)
if image_response.status_code == 200:
with open(image_path, 'wb') as file:
file.write(image_response.content)
else:
print(f'Failed to download {url}')
总结
通过上述步骤,我们可以使用Python抓取彼岸桌面图片。这些步骤包括使用requests库下载网页、使用BeautifulSoup解析HTML、提取图片URL、下载图片、处理反爬虫机制。希望这篇文章对你有所帮助。
相关问答FAQs:
如何使用Python抓取彼岸桌面的图片?
要抓取彼岸桌面的图片,您可以使用Python中的requests库来获取网页内容,并利用BeautifulSoup库解析HTML。首先,确保安装了这两个库。接着,通过分析彼岸桌面网站的结构,找到图片的链接,然后用requests下载这些图片并保存到本地。
抓取彼岸桌面图片需要哪些Python库?
进行抓取工作时,推荐使用requests库来处理网络请求,BeautifulSoup用于解析HTML文档。此外,如果您需要处理图片格式,可以使用Pillow库(PIL)来处理和保存下载的图片。这些库能有效协助您完成抓取任务。
抓取图片时如何处理反爬虫机制?
在抓取彼岸桌面图片时,可能会遇到反爬虫机制。您可以尝试设置请求头以模拟浏览器行为,例如添加User-Agent信息。此外,可以考虑使用时间间隔随机化请求频率,避免频繁请求同一页面。同时,使用代理IP也是一种有效的方式来规避检测。
如何确保抓取到的图片质量?
抓取时,确保选择合适的图片源和清晰度。您可以在网页中查看图片的分辨率,并选择高质量的版本进行下载。此外,在下载图片时,可使用requests的content属性直接获取二进制数据,确保保存的图片质量不受影响。
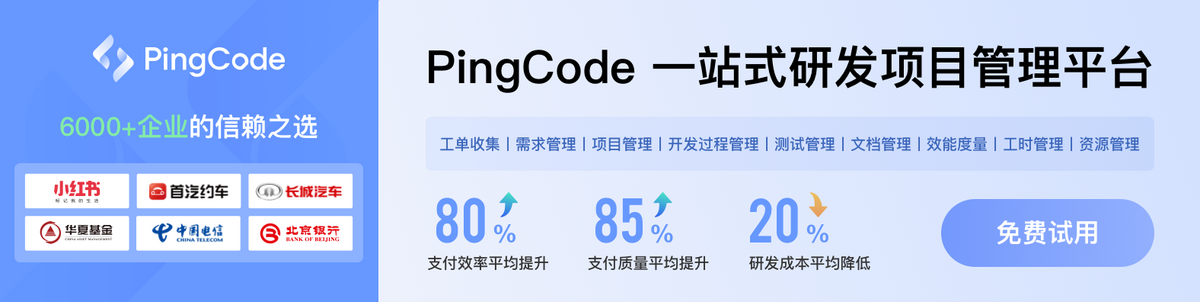