要用Python制作黑白菊花图像,可以使用Python的图像处理库,例如Pillow或OpenCV。 这些库提供了丰富的功能,可以轻松地对图像进行各种操作,如颜色转换、图像绘制等。使用Pillow,加载彩色图像、将图像转换为黑白、绘制菊花图案 是实现这一目标的主要步骤。下面将详细描述如何使用Pillow库来完成这个任务。
一、安装Pillow库
首先,确保你已经安装了Pillow库。如果没有安装,可以使用以下命令进行安装:
pip install Pillow
二、加载和转换图像
使用Pillow库加载图像,并将其转换为黑白图像。以下是基本的代码示例:
from PIL import Image
加载图像
image = Image.open('flower.jpg')
将图像转换为黑白
bw_image = image.convert('L')
保存黑白图像
bw_image.save('bw_flower.jpg')
三、绘制菊花图案
绘制菊花图案需要使用Pillow库中的ImageDraw
模块。以下是绘制菊花图案的基本步骤:
from PIL import Image, ImageDraw
创建一个空白图像
width, height = 800, 800
image = Image.new('L', (width, height), 255)
draw = ImageDraw.Draw(image)
绘制菊花图案
center_x, center_y = width // 2, height // 2
num_petals = 20
petal_length = 300
petal_width = 10
for i in range(num_petals):
angle = i * (360 / num_petals)
end_x = center_x + petal_length * cos(radians(angle))
end_y = center_y + petal_length * sin(radians(angle))
draw.line((center_x, center_y, end_x, end_y), fill=0, width=petal_width)
保存图像
image.save('bw_chrysanthemum.jpg')
四、详细讲解绘制菊花图案
1、计算菊花花瓣的角度和位置
为了绘制菊花图案,我们需要计算每个花瓣的角度和位置。通过将360度平均分成指定数量的花瓣,可以确定每个花瓣的起始角度。然后使用三角函数计算花瓣的终点坐标。
import math
center_x, center_y = width // 2, height // 2
num_petals = 20
petal_length = 300
petal_width = 10
for i in range(num_petals):
angle = i * (360 / num_petals)
end_x = center_x + petal_length * math.cos(math.radians(angle))
end_y = center_y + petal_length * math.sin(math.radians(angle))
draw.line((center_x, center_y, end_x, end_y), fill=0, width=petal_width)
2、使用ImageDraw模块绘制花瓣
通过ImageDraw.Draw
类中的line
方法,可以绘制直线来表示花瓣。设置线条的起点为图像的中心点,终点为前面计算得到的坐标。
draw.line((center_x, center_y, end_x, end_y), fill=0, width=petal_width)
五、添加细节和优化图像
1、增加花瓣的细节
为了使菊花图案更加真实,可以增加花瓣的细节,例如弯曲的花瓣、渐变的颜色等。可以使用贝塞尔曲线或其他复杂的图形算法来实现这些细节。
from PIL import Image, ImageDraw
import math
def draw_chrysanthemum(draw, center_x, center_y, num_petals, petal_length, petal_width):
for i in range(num_petals):
angle = i * (360 / num_petals)
end_x = center_x + petal_length * math.cos(math.radians(angle))
end_y = center_y + petal_length * math.sin(math.radians(angle))
draw.line((center_x, center_y, end_x, end_y), fill=0, width=petal_width)
width, height = 800, 800
image = Image.new('L', (width, height), 255)
draw = ImageDraw.Draw(image)
draw_chrysanthemum(draw, width // 2, height // 2, 20, 300, 10)
image.save('detailed_chrysanthemum.jpg')
2、优化图像质量
可以应用一些图像处理技术来优化生成的图像,例如抗锯齿、模糊处理等。Pillow库提供了多种滤镜,可以用来改善图像质量。
from PIL import ImageFilter
应用模糊滤镜
image = image.filter(ImageFilter.GaussianBlur(2))
保存优化后的图像
image.save('optimized_chrysanthemum.jpg')
六、总结
通过使用Pillow库,我们可以轻松地加载、转换和处理图像,并使用ImageDraw
模块绘制复杂的图形,如黑白菊花图案。主要步骤包括加载图像、转换为黑白、计算花瓣位置、绘制花瓣以及优化图像质量。这些步骤可以根据具体需求进行调整和优化,以生成更加复杂和精美的图像。
七、完整代码示例
以下是完整的代码示例,展示了如何使用Python和Pillow库制作一个黑白菊花图像:
from PIL import Image, ImageDraw, ImageFilter
import math
def draw_chrysanthemum(draw, center_x, center_y, num_petals, petal_length, petal_width):
for i in range(num_petals):
angle = i * (360 / num_petals)
end_x = center_x + petal_length * math.cos(math.radians(angle))
end_y = center_y + petal_length * math.sin(math.radians(angle))
draw.line((center_x, center_y, end_x, end_y), fill=0, width=petal_width)
width, height = 800, 800
image = Image.new('L', (width, height), 255)
draw = ImageDraw.Draw(image)
draw_chrysanthemum(draw, width // 2, height // 2, 20, 300, 10)
应用模糊滤镜
image = image.filter(ImageFilter.GaussianBlur(2))
保存图像
image.save('final_chrysanthemum.jpg')
通过以上步骤,你可以使用Python和Pillow库制作出一个精美的黑白菊花图像。根据具体需求,还可以进一步调整和优化代码,以生成更加复杂和精美的图案。
相关问答FAQs:
如何使用Python创建黑白菊花图案?
要使用Python创建黑白菊花图案,可以使用库如Matplotlib和NumPy进行绘图。首先,确保安装了这些库。可以通过pip install matplotlib numpy
命令进行安装。接下来,利用极坐标绘制菊花形状,并通过调整参数来实现黑白效果。以下是一个简单的示例代码,展示了如何绘制这样的图案。
我需要具备什么基础才能使用Python绘制菊花?
为了顺利使用Python绘制黑白菊花,建议具备Python的基本知识,包括数据类型、控制结构和函数定义。此外,熟悉NumPy和Matplotlib库的基本用法将有助于理解绘图过程。可以通过在线教程或书籍来提升相关技能。
有哪些技巧可以让我的黑白菊花图案更具艺术感?
为了让你的黑白菊花图案更具艺术感,可以尝试以下几种方法:使用不同的线条粗细和样式来增强图案的层次感,调整图案的对称性和比例,以创造更独特的效果。此外,可以在菊花周围添加阴影或背景元素,以增强整体视觉效果。使用渐变色或者不同的黑白色调组合,也可以提升艺术性。
如何保存我绘制的黑白菊花图案?
在Matplotlib中,可以使用savefig()
函数将绘制的图案保存为不同格式的文件,比如PNG、JPEG等。只需在绘图完成后调用plt.savefig('filename.png')
,并指定所需的文件名和格式。确保在调用此函数之前,已完成所有绘图设置,以确保图像质量最佳。
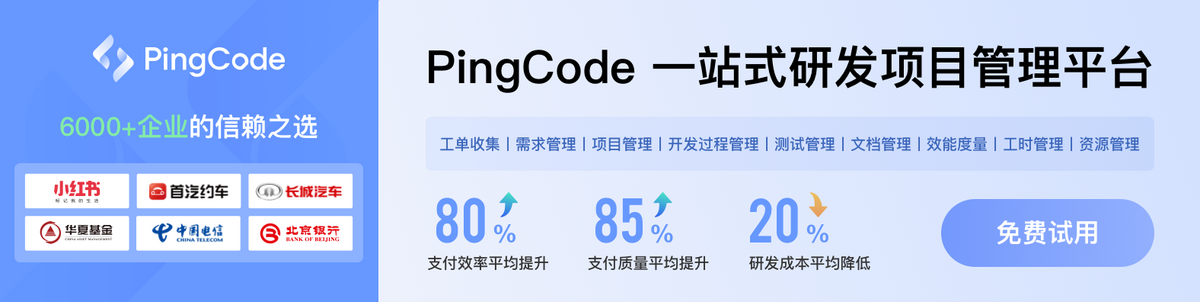