使用Python统计字符串的行数有多种方法,包括使用split()方法、count()方法、以及通过循环遍历字符串来统计。 其中,最常用且最简单的方法是使用split()方法,因为它可以直接将字符串按行分割成列表,然后通过len()函数来获取列表的长度,从而得到行数。下面我们详细介绍这几种方法的实现。
一、使用split()方法
使用split()方法是统计字符串行数的最简单和最常用的方法。首先,通过split()方法将字符串按换行符分割成一个列表,然后通过len()函数获取列表的长度,即为字符串的行数。
def count_lines_using_split(input_string):
lines = input_string.split('\n')
return len(lines)
示例
input_string = "Hello, World!\nWelcome to Python programming.\nEnjoy coding!"
line_count = count_lines_using_split(input_string)
print(f"Number of lines: {line_count}")
在上面的示例中,字符串被按换行符分割成一个列表,列表的长度即为字符串的行数。这个方法简单明了,适用于大多数情况。
二、使用count()方法
另一种方法是使用count()方法来统计换行符的数量。由于每一个换行符代表一行,统计换行符的数量再加1即可得到字符串的行数。
def count_lines_using_count(input_string):
return input_string.count('\n') + 1
示例
input_string = "Hello, World!\nWelcome to Python programming.\nEnjoy coding!"
line_count = count_lines_using_count(input_string)
print(f"Number of lines: {line_count}")
在这个示例中,我们使用count('\n')来统计字符串中的换行符数量,然后加1得到行数。这种方法也非常简洁,但需要注意的是,如果字符串最后一个字符是换行符,这种方法可能会多统计一行。
三、使用循环遍历字符串
对于一些特殊情况,比如需要对每一行进行额外处理,可以通过循环遍历字符串来统计行数。这种方法虽然相对复杂,但在需要逐行处理字符串时非常有用。
def count_lines_using_loop(input_string):
line_count = 0
for char in input_string:
if char == '\n':
line_count += 1
return line_count + 1
示例
input_string = "Hello, World!\nWelcome to Python programming.\nEnjoy coding!"
line_count = count_lines_using_loop(input_string)
print(f"Number of lines: {line_count}")
在这个示例中,我们通过遍历字符串中的每一个字符来统计换行符的数量,从而得到行数。这种方法适用于需要对每一行进行特殊处理的情况。
四、综合使用多种方法
在实际应用中,有时候需要综合使用多种方法来确保统计结果的准确性。例如,可以先使用split()方法获取大致的行数,然后再使用循环遍历方法对每一行进行进一步处理。
def count_lines_comprehensive(input_string):
lines = input_string.split('\n')
line_count = len(lines)
# 进一步处理每一行(示例:统计每行的字符数)
for line in lines:
char_count = len(line)
print(f"Line: '{line}' has {char_count} characters")
return line_count
示例
input_string = "Hello, World!\nWelcome to Python programming.\nEnjoy coding!"
line_count = count_lines_comprehensive(input_string)
print(f"Number of lines: {line_count}")
在这个示例中,我们首先使用split()方法获取行数,然后对每一行进行字符统计。这种方法结合了多种方法的优点,适用于需要对字符串进行复杂处理的情况。
五、处理特殊情况
在实际应用中,有时候会遇到一些特殊情况,比如字符串中包含多个连续的换行符,或者字符串为空。这些情况都需要在代码中进行处理。
def count_lines_with_special_cases(input_string):
if not input_string:
return 0
lines = input_string.split('\n')
line_count = len(lines)
# 处理多余的换行符
if input_string.endswith('\n'):
line_count -= 1
return line_count
示例
input_string = "Hello, World!\n\nWelcome to Python programming.\nEnjoy coding!\n"
line_count = count_lines_with_special_cases(input_string)
print(f"Number of lines: {line_count}")
在这个示例中,我们首先检查字符串是否为空,然后处理字符串末尾多余的换行符。这种方法适用于需要处理特殊情况的统计需求。
总的来说,统计字符串的行数有多种方法可供选择,包括split()方法、count()方法、循环遍历方法等。 根据具体需求选择合适的方法,可以确保统计结果的准确性和代码的简洁性。在实际应用中,结合使用多种方法处理特殊情况,可以提高代码的健壮性和可靠性。
相关问答FAQs:
如何在Python中读取文件并统计其中的行数?
在Python中,可以使用内置的open()
函数打开文件,并通过读取每一行来统计行数。可以使用with
语句确保文件正确关闭。示例代码如下:
with open('yourfile.txt', 'r') as file:
line_count = sum(1 for line in file)
print(f'文件的行数为: {line_count}')
这种方法高效且易于理解,适合处理大文件。
Python中如何处理多行字符串并计算行数?
如果你有一个多行字符串,可以使用splitlines()
方法将其分割成行,并返回行数。示例代码如下:
multiline_string = """第一行
第二行
第三行"""
line_count = len(multiline_string.splitlines())
print(f'字符串的行数为: {line_count}')
这种方法适用于在内存中处理文本,而无需读取外部文件。
有哪些常用的Python库可以用于统计文本行数?
除了基本的字符串操作,Python的pandas
库也可以高效地读取大文件并统计行数。使用read_csv()
或read_table()
方法可以轻松实现。以下是一个示例:
import pandas as pd
df = pd.read_csv('yourfile.csv')
line_count = len(df)
print(f'文件的行数为: {line_count}')
这种方法特别适合需要处理表格数据的场景,同时也便于后续的数据分析。
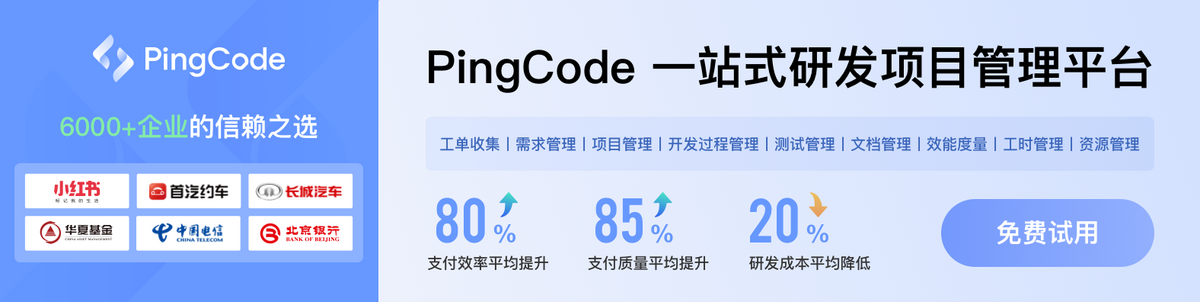