Python中两台电机如何同时控制
同时控制两台电机在Python中,可以使用多线程、多进程、异步编程、控制器库等技术。本文将详细介绍其中的多线程方法。
在控制电机的场景中,经常需要同时控制多台电机以实现复杂的机械动作。Python提供了多种方法来实现这一需求,其中多线程是最常用且易于理解的方法之一。通过多线程,我们可以让两台电机在同一时间内执行不同的任务,从而达到同步控制的效果。
一、多线程控制
多线程是一种并发执行的方式,它允许程序在同一时间内运行多个线程,从而提高程序的效率。在Python中,可以使用threading
模块来实现多线程控制。
1、创建线程
在使用多线程控制电机时,首先需要创建线程。每个线程对应一个电机的控制任务。以下是一个简单的例子,展示了如何创建和启动两个线程来控制两台电机:
import threading
import time
def control_motor_1():
while True:
print("Motor 1 is running")
time.sleep(1)
def control_motor_2():
while True:
print("Motor 2 is running")
time.sleep(1)
创建线程
thread1 = threading.Thread(target=control_motor_1)
thread2 = threading.Thread(target=control_motor_2)
启动线程
thread1.start()
thread2.start()
在这个例子中,我们定义了两个函数control_motor_1
和control_motor_2
,分别用于控制电机1和电机2。然后,我们创建了两个线程,并将这两个函数作为目标函数传递给线程。最后,通过调用start()
方法启动线程。
2、线程同步
在多线程控制电机时,有时需要确保两个电机的动作同步进行。可以使用threading.Event
对象来实现线程同步。以下是一个例子,展示了如何使用Event
对象来同步两个电机的启动:
import threading
import time
start_event = threading.Event()
def control_motor_1():
start_event.wait() # 等待启动信号
while True:
print("Motor 1 is running")
time.sleep(1)
def control_motor_2():
start_event.wait() # 等待启动信号
while True:
print("Motor 2 is running")
time.sleep(1)
创建线程
thread1 = threading.Thread(target=control_motor_1)
thread2 = threading.Thread(target=control_motor_2)
启动线程
thread1.start()
thread2.start()
发出启动信号
start_event.set()
在这个例子中,我们创建了一个Event
对象start_event
,并在两个线程中使用wait()
方法等待启动信号。主线程在启动两个线程后,通过调用set()
方法发出启动信号,从而使两个电机同步启动。
二、多进程控制
除了多线程外,多进程也是一种实现多任务并行的方法。在Python中,可以使用multiprocessing
模块来创建和管理多个进程。与多线程相比,多进程可以利用多核CPU的优势,提高程序的执行效率。
1、创建进程
以下是一个例子,展示了如何使用multiprocessing
模块创建和启动两个进程来控制两台电机:
import multiprocessing
import time
def control_motor_1():
while True:
print("Motor 1 is running")
time.sleep(1)
def control_motor_2():
while True:
print("Motor 2 is running")
time.sleep(1)
if __name__ == '__main__':
# 创建进程
process1 = multiprocessing.Process(target=control_motor_1)
process2 = multiprocessing.Process(target=control_motor_2)
# 启动进程
process1.start()
process2.start()
# 等待进程结束
process1.join()
process2.join()
在这个例子中,我们定义了两个函数control_motor_1
和control_motor_2
,分别用于控制电机1和电机2。然后,我们创建了两个进程,并将这两个函数作为目标函数传递给进程。最后,通过调用start()
方法启动进程,并使用join()
方法等待进程结束。
2、进程同步
与多线程类似,在多进程控制电机时,也需要确保两个电机的动作同步进行。可以使用multiprocessing.Event
对象来实现进程同步。以下是一个例子,展示了如何使用Event
对象来同步两个电机的启动:
import multiprocessing
import time
start_event = multiprocessing.Event()
def control_motor_1():
start_event.wait() # 等待启动信号
while True:
print("Motor 1 is running")
time.sleep(1)
def control_motor_2():
start_event.wait() # 等待启动信号
while True:
print("Motor 2 is running")
time.sleep(1)
if __name__ == '__main__':
# 创建进程
process1 = multiprocessing.Process(target=control_motor_1)
process2 = multiprocessing.Process(target=control_motor_2)
# 启动进程
process1.start()
process2.start()
# 发出启动信号
start_event.set()
# 等待进程结束
process1.join()
process2.join()
在这个例子中,我们创建了一个Event
对象start_event
,并在两个进程中使用wait()
方法等待启动信号。主进程在启动两个子进程后,通过调用set()
方法发出启动信号,从而使两个电机同步启动。
三、异步编程
异步编程是一种更加高效的并发执行方式,特别适用于I/O密集型任务。在Python中,可以使用asyncio
模块来实现异步编程。通过异步编程,我们可以在单线程中实现并发执行,从而提高程序的效率。
1、异步函数
在使用asyncio
模块时,需要将电机控制任务定义为异步函数。以下是一个例子,展示了如何使用asyncio
模块来控制两台电机:
import asyncio
async def control_motor_1():
while True:
print("Motor 1 is running")
await asyncio.sleep(1)
async def control_motor_2():
while True:
print("Motor 2 is running")
await asyncio.sleep(1)
async def main():
# 创建任务
task1 = asyncio.create_task(control_motor_1())
task2 = asyncio.create_task(control_motor_2())
# 等待任务完成
await task1
await task2
运行主函数
asyncio.run(main())
在这个例子中,我们定义了两个异步函数control_motor_1
和control_motor_2
,分别用于控制电机1和电机2。然后,我们在主函数main
中创建了两个任务,并使用await
关键字等待任务完成。最后,通过调用asyncio.run()
方法运行主函数。
2、任务同步
在异步编程中,可以使用asyncio.Event
对象来实现任务同步。以下是一个例子,展示了如何使用Event
对象来同步两个电机的启动:
import asyncio
start_event = asyncio.Event()
async def control_motor_1():
await start_event.wait() # 等待启动信号
while True:
print("Motor 1 is running")
await asyncio.sleep(1)
async def control_motor_2():
await start_event.wait() # 等待启动信号
while True:
print("Motor 2 is running")
await asyncio.sleep(1)
async def main():
# 创建任务
task1 = asyncio.create_task(control_motor_1())
task2 = asyncio.create_task(control_motor_2())
# 发出启动信号
start_event.set()
# 等待任务完成
await task1
await task2
运行主函数
asyncio.run(main())
在这个例子中,我们创建了一个Event
对象start_event
,并在两个异步函数中使用await
关键字等待启动信号。主函数在创建任务后,通过调用set()
方法发出启动信号,从而使两个电机同步启动。
四、控制器库
除了使用多线程、多进程和异步编程外,还可以使用专门的控制器库来实现电机的同步控制。以下是一些常用的控制器库:
1、Raspberry Pi GPIO库
对于使用Raspberry Pi控制电机的场景,可以使用RPi.GPIO
库来控制GPIO引脚。以下是一个例子,展示了如何使用RPi.GPIO
库来控制两台电机:
import RPi.GPIO as GPIO
import time
设置GPIO模式
GPIO.setmode(GPIO.BCM)
定义电机引脚
motor_1_pin = 17
motor_2_pin = 18
设置引脚为输出模式
GPIO.setup(motor_1_pin, GPIO.OUT)
GPIO.setup(motor_2_pin, GPIO.OUT)
def control_motor_1():
while True:
GPIO.output(motor_1_pin, GPIO.HIGH)
time.sleep(1)
GPIO.output(motor_1_pin, GPIO.LOW)
time.sleep(1)
def control_motor_2():
while True:
GPIO.output(motor_2_pin, GPIO.HIGH)
time.sleep(1)
GPIO.output(motor_2_pin, GPIO.LOW)
time.sleep(1)
创建线程
thread1 = threading.Thread(target=control_motor_1)
thread2 = threading.Thread(target=control_motor_2)
启动线程
thread1.start()
thread2.start()
在这个例子中,我们使用RPi.GPIO
库设置了GPIO引脚的模式,并定义了两个函数control_motor_1
和control_motor_2
用于控制电机的开关。然后,我们创建了两个线程,并将这两个函数作为目标函数传递给线程。最后,通过调用start()
方法启动线程。
2、Adafruit Motor库
对于使用Adafruit电机驱动器的场景,可以使用Adafruit_MotorHAT
库来控制电机。以下是一个例子,展示了如何使用Adafruit_MotorHAT
库来控制两台电机:
from Adafruit_MotorHAT import Adafruit_MotorHAT, Adafruit_DCMotor
import time
import atexit
创建电机帽对象
mh = Adafruit_MotorHAT(addr=0x60)
自动释放资源
def turnOffMotors():
mh.getMotor(1).run(Adafruit_MotorHAT.RELEASE)
mh.getMotor(2).run(Adafruit_MotorHAT.RELEASE)
atexit.register(turnOffMotors)
获取电机对象
motor1 = mh.getMotor(1)
motor2 = mh.getMotor(2)
def control_motor_1():
while True:
motor1.run(Adafruit_MotorHAT.FORWARD)
motor1.setSpeed(150)
time.sleep(1)
motor1.run(Adafruit_MotorHAT.RELEASE)
time.sleep(1)
def control_motor_2():
while True:
motor2.run(Adafruit_MotorHAT.FORWARD)
motor2.setSpeed(150)
time.sleep(1)
motor2.run(Adafruit_MotorHAT.RELEASE)
time.sleep(1)
创建线程
thread1 = threading.Thread(target=control_motor_1)
thread2 = threading.Thread(target=control_motor_2)
启动线程
thread1.start()
thread2.start()
在这个例子中,我们使用Adafruit_MotorHAT
库创建了电机帽对象,并通过getMotor()
方法获取电机对象。然后,我们定义了两个函数control_motor_1
和control_motor_2
用于控制电机的运行。最后,我们创建了两个线程,并将这两个函数作为目标函数传递给线程,通过调用start()
方法启动线程。
通过以上方法,我们可以在Python中实现两台电机的同步控制。根据具体的应用场景,可以选择使用多线程、多进程、异步编程或控制器库来实现并发执行。无论选择哪种方法,都需要确保电机的动作同步,以达到预期的控制效果。
相关问答FAQs:
在Python中如何控制两台电机同时运行?
要同时控制两台电机,可以使用多线程或异步编程的方法。通过Python的threading
模块,可以为每台电机创建一个线程,这样它们就可以并行运行。同时,使用asyncio
模块也可以实现非阻塞的异步控制。确保您的电机控制库支持这些方法。
使用Python控制电机时需要注意哪些问题?
在控制电机时,确保电机的电源和驱动器能够满足同时运行的需求。此外,注意电机的负载和速度设置,避免出现过载或过热的情况。调试时,可以逐步增加负载,以确保系统的稳定性。
有没有推荐的Python库可以用来控制多台电机?
常用的Python库包括Raspberry Pi GPIO
,适用于树莓派控制电机;PyFirmata
,可以通过Arduino控制电机;以及pigpio
,提供更精细的控制和监控功能。根据您的硬件平台选择合适的库,并查看相关文档以获取详细的使用指南。
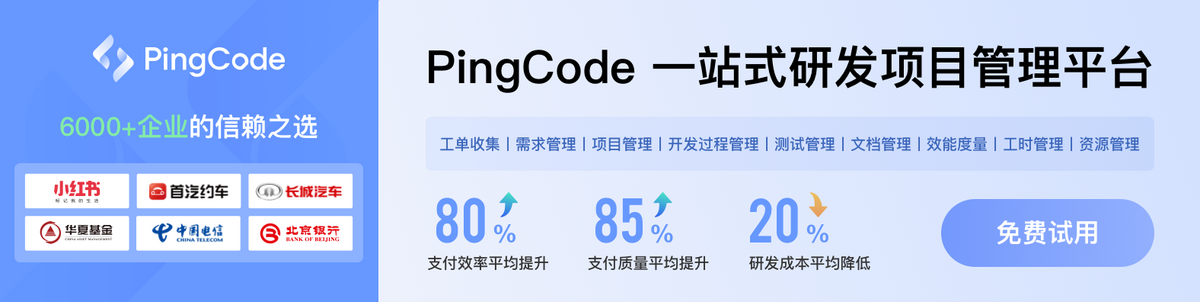