Python里查数据类型的方法有:使用type()函数、使用isinstance()函数、使用内置类型检查方法
在Python编程中,了解变量的数据类型是至关重要的。type()函数可以直接返回变量的类型,isinstance()函数则可以用来检查一个变量是否是某个特定类型。下面详细介绍这些方法。
一、使用type()函数
type()函数是Python内置函数之一,能够返回变量的类型。无论是基本数据类型(如int、float、str等)还是复杂数据类型(如list、dict、set等),都可以使用type()函数来检测。
1. 基本使用方法
type()函数的基本使用方法非常简单,只需将变量名传递给type()函数即可。
# 示例代码
a = 10
b = 3.14
c = "Hello, World!"
d = [1, 2, 3]
print(type(a)) # <class 'int'>
print(type(b)) # <class 'float'>
print(type(c)) # <class 'str'>
print(type(d)) # <class 'list'>
2. 与类型比较
有时我们不仅需要知道变量的类型,还需要将其与特定类型进行比较。这时可以用type()函数返回的结果与类型进行比较。
# 示例代码
a = 10
if type(a) == int:
print("a is an integer")
else:
print("a is not an integer")
二、使用isinstance()函数
isinstance()函数也是Python内置函数之一,与type()函数不同的是,isinstance()不仅可以检查变量的类型,还可以检查变量是否是某个类型的子类。
1. 基本使用方法
isinstance()函数的基本使用方法是将变量和类型作为参数传递给函数。
# 示例代码
a = 10
b = 3.14
c = "Hello, World!"
d = [1, 2, 3]
print(isinstance(a, int)) # True
print(isinstance(b, float)) # True
print(isinstance(c, str)) # True
print(isinstance(d, list)) # True
2. 检查多个类型
isinstance()函数还允许我们检查一个变量是否属于多个类型中的一个。只需将类型作为元组传递给isinstance()函数。
# 示例代码
a = 10
if isinstance(a, (int, float)):
print("a is either an integer or a float")
else:
print("a is neither an integer nor a float")
三、使用内置类型检查方法
除了type()和isinstance()函数,Python还提供了一些内置类型检查方法。比如,对于字符串数据类型,我们可以使用str的内置方法。
1. 检查字符串类型
使用str的内置方法来检查变量是否是字符串类型。
# 示例代码
a = "Hello, World!"
if isinstance(a, str):
print("a is a string")
else:
print("a is not a string")
2. 检查列表类型
使用list的内置方法来检查变量是否是列表类型。
# 示例代码
a = [1, 2, 3]
if isinstance(a, list):
print("a is a list")
else:
print("a is not a list")
四、类型检查的实际应用
在实际编程中,类型检查有助于提高代码的健壮性和可读性。以下是几个实际应用中的例子。
1. 函数参数类型检查
在编写函数时,我们可以使用类型检查来确保传递给函数的参数是正确的类型。
# 示例代码
def add(a, b):
if not isinstance(a, (int, float)) or not isinstance(b, (int, float)):
raise TypeError("Both arguments must be integers or floats")
return a + b
result = add(10, 5.5)
print(result) # 15.5
2. 数据处理中的类型检查
在数据处理过程中,类型检查也非常重要。比如,在处理来自用户输入的数据时,我们需要确保数据的类型正确。
# 示例代码
user_input = input("Enter a number: ")
try:
number = float(user_input)
print(f"The number you entered is: {number}")
except ValueError:
print("Invalid input. Please enter a valid number.")
五、总结
通过本文的介绍,我们了解了Python中查数据类型的几种方法:type()函数、isinstance()函数、内置类型检查方法。这些方法在编写代码时非常有用,可以帮助我们确保变量的类型正确,提高代码的健壮性和可读性。在实际编程中,合理使用这些方法可以有效地避免类型错误,从而提升程序的稳定性和性能。
相关问答FAQs:
如何在Python中检查一个变量的数据类型?
在Python中,可以使用内置的type()
函数来检查一个变量的数据类型。例如,若要查看变量x
的类型,只需输入type(x)
,这将返回x
的类型信息,如<class 'int'>
表示整型。
Python中是否有其他方法可以判断数据类型?
除了type()
函数,Python还提供了isinstance()
函数,允许用户检查变量是否属于特定的数据类型。这种方法在处理类继承时尤其有用。例如,isinstance(x, int)
将返回True
或False
,表明x
是否为整型。
如何在Python中处理不同数据类型的操作?
在Python中,可以对不同类型的数据执行多种操作,但需要注意类型兼容性。例如,进行数学运算时,整型和浮点型可以直接相加,但字符串与数字相加时会抛出错误。在处理数据时,确保使用适当的转换函数(如int()
、float()
、str()
)来避免类型错误。
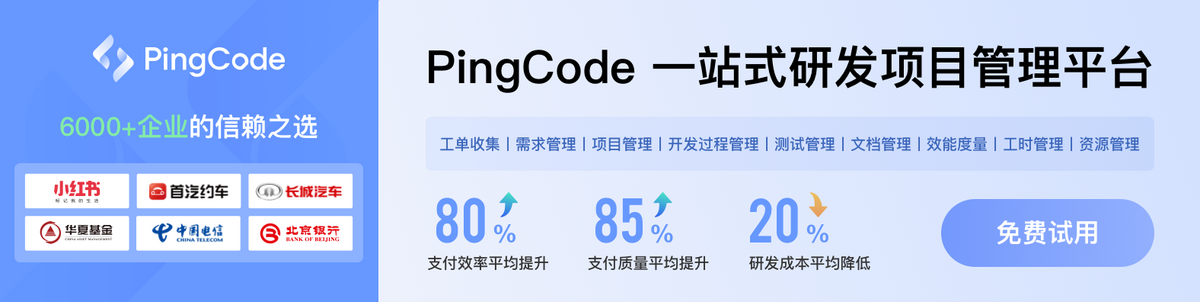