使用Python抓取新浪财经网数据的步骤包括:选择合适的库、解析HTML内容、处理反爬机制、存储数据。 首先,我们选择合适的库,如requests
、BeautifulSoup
和pandas
。接着,使用requests
库获取网页HTML内容,然后利用BeautifulSoup
解析并提取所需数据。接下来,要处理反爬机制,如设置User-Agent或使用代理IP。最后,将数据存储到本地文件或数据库中。下面详细介绍其中的一个步骤,即如何使用BeautifulSoup
解析HTML内容。
要解析HTML内容,首先安装并导入BeautifulSoup
库。然后使用requests
获取网页内容,并将其传递给BeautifulSoup
进行解析。通过指定HTML元素的标签、类名或ID,可以提取出所需的数据。以下是一个示例代码:
import requests
from bs4 import BeautifulSoup
url = 'https://finance.sina.com.cn/'
headers = {'User-Agent': 'Mozilla/5.0'}
response = requests.get(url, headers=headers)
soup = BeautifulSoup(response.content, 'html.parser')
titles = soup.find_all('h2', class_='news-title')
for title in titles:
print(title.get_text())
一、安装和导入所需库
在使用Python进行网页抓取时,需要安装并导入一些必要的库。这些库包括requests
、BeautifulSoup
和pandas
。以下是安装和导入这些库的步骤:
pip install requests
pip install beautifulsoup4
pip install pandas
安装完成后,在代码中导入这些库:
import requests
from bs4 import BeautifulSoup
import pandas as pd
二、获取网页内容
使用requests
库发送HTTP请求,并获取网页的HTML内容。为了避免被反爬机制阻挡,可以在请求头中设置User-Agent。以下是获取新浪财经网首页内容的示例代码:
url = 'https://finance.sina.com.cn/'
headers = {'User-Agent': 'Mozilla/5.0'}
response = requests.get(url, headers=headers)
if response.status_code == 200:
html_content = response.content
else:
print("Failed to retrieve the webpage.")
三、解析网页内容
使用BeautifulSoup
库解析获取到的HTML内容。通过指定HTML元素的标签、类名或ID,可以提取出所需的数据。以下是解析新浪财经网首页新闻标题的示例代码:
soup = BeautifulSoup(html_content, 'html.parser')
titles = soup.find_all('h2', class_='news-title')
for title in titles:
print(title.get_text())
四、处理反爬机制
为了应对网站的反爬机制,可以采取以下措施:设置User-Agent、使用代理IP、模拟浏览器行为等。设置User-Agent的代码示例如上所示。以下是使用代理IP的示例代码:
proxies = {
'http': 'http://your_proxy_ip:your_proxy_port',
'https': 'https://your_proxy_ip:your_proxy_port'
}
response = requests.get(url, headers=headers, proxies=proxies)
五、存储数据
将抓取到的数据存储到本地文件或数据库中。可以使用pandas
库将数据保存为CSV文件,或者使用sqlite3
库将数据存储到SQLite数据库中。以下是存储数据的示例代码:
data = {'Title': [title.get_text() for title in titles]}
df = pd.DataFrame(data)
df.to_csv('sina_finance_titles.csv', index=False)
六、示例代码汇总
将上述步骤整合在一起,得到一个完整的示例代码:
import requests
from bs4 import BeautifulSoup
import pandas as pd
def get_sina_finance_titles():
url = 'https://finance.sina.com.cn/'
headers = {'User-Agent': 'Mozilla/5.0'}
response = requests.get(url, headers=headers)
if response.status_code == 200:
html_content = response.content
soup = BeautifulSoup(html_content, 'html.parser')
titles = soup.find_all('h2', class_='news-title')
data = {'Title': [title.get_text() for title in titles]}
df = pd.DataFrame(data)
df.to_csv('sina_finance_titles.csv', index=False)
print("Data has been saved to sina_finance_titles.csv")
else:
print("Failed to retrieve the webpage.")
get_sina_finance_titles()
七、扩展功能
在实际应用中,可能需要抓取更多的内容,如新闻链接、发布时间等。可以通过进一步解析HTML元素,提取更多信息。例如,提取新闻链接的代码如下:
titles = soup.find_all('h2', class_='news-title')
links = [title.find('a')['href'] for title in titles if title.find('a')]
data = {
'Title': [title.get_text() for title in titles],
'Link': links
}
此外,还可以通过定时任务定期抓取数据,并对数据进行分析和可视化。使用apscheduler
库可以实现定时任务。以下是示例代码:
from apscheduler.schedulers.blocking import BlockingScheduler
scheduler = BlockingScheduler()
@scheduler.scheduled_job('interval', hours=1)
def scheduled_job():
get_sina_finance_titles()
scheduler.start()
八、处理动态加载内容
有些网页内容是通过JavaScript动态加载的,使用requests
库无法直接获取。这时可以使用selenium
库模拟浏览器行为,加载动态内容。以下是使用selenium
抓取动态内容的示例代码:
from selenium import webdriver
from bs4 import BeautifulSoup
def get_dynamic_content():
url = 'https://finance.sina.com.cn/'
driver = webdriver.Chrome()
driver.get(url)
html_content = driver.page_source
soup = BeautifulSoup(html_content, 'html.parser')
titles = soup.find_all('h2', class_='news-title')
data = {'Title': [title.get_text() for title in titles]}
df = pd.DataFrame(data)
df.to_csv('sina_finance_titles_dynamic.csv', index=False)
print("Data has been saved to sina_finance_titles_dynamic.csv")
driver.quit()
get_dynamic_content()
九、总结
通过以上步骤,我们可以使用Python抓取新浪财经网的数据,并将其存储到本地文件或数据库中。主要步骤包括:安装和导入所需库、获取网页内容、解析网页内容、处理反爬机制、存储数据、处理动态加载内容等。在实际应用中,可以根据需要扩展功能,如定期抓取数据、提取更多内容、进行数据分析和可视化等。通过不断优化和完善代码,可以提高抓取数据的效率和准确性。
相关问答FAQs:
如何用Python抓取新浪财经网的数据?
使用Python抓取新浪财经网可以通过利用第三方库如requests
来发送HTTP请求和BeautifulSoup
来解析HTML页面。首先,需确认你已经安装了这些库。可以使用pip install requests beautifulsoup4
来安装。通过发送请求到新浪财经网的特定页面,获取网页内容后,使用BeautifulSoup解析和提取需要的数据。
抓取新浪财经网时需要注意哪些法律和道德问题?
在抓取数据时,遵循网站的robots.txt
文件是非常重要的。这个文件指明了哪些内容可以被抓取,哪些内容不可以。此外,频繁的请求可能会对服务器造成负担,因此应该设置适当的请求间隔,避免被视为恶意抓取。保持尊重和合规,才能维护良好的网络环境。
如何处理抓取过程中遇到的反爬虫机制?
新浪财经网可能会实施一些反爬虫措施,比如限制请求频率或检测用户代理。为了应对这些情况,可以考虑使用代理服务器来更改IP地址,或随机生成用户代理字符串,使请求看起来更像是来自真实用户。此外,使用时间延迟和伪装浏览器行为等策略也能有效降低被识别的风险。
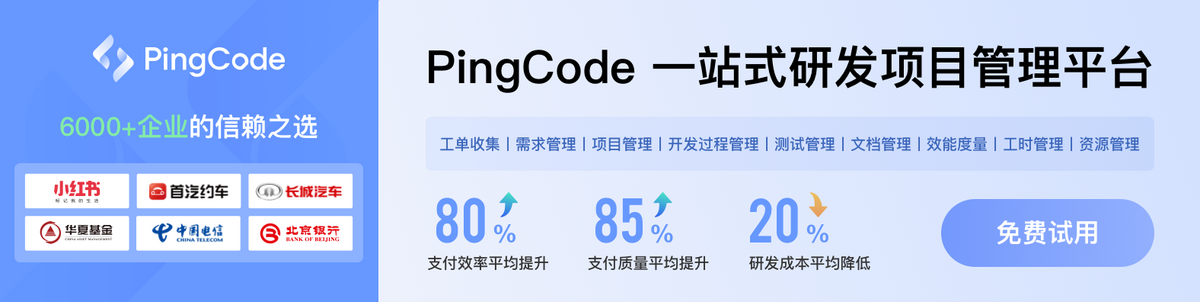