要用一串代码了解Python,可以通过编写一个综合性的脚本,展示Python的基本语法、数据结构、函数、面向对象编程、文件操作和错误处理等特点。 通过这样的代码,初学者可以快速了解Python的各个方面。下面,我们将详细介绍如何通过一段代码来学习和理解Python的各个方面。
一、Python基础语法
Python的基础语法包括变量的定义、基本的数据类型、运算符以及基本的输入输出操作。以下是一个简单的示例代码:
# 变量定义
name = "Alice"
age = 30
height = 1.75
基本数据类型
is_student = False
scores = [85, 90, 78, 92]
info = {"name": "Alice", "age": 30, "height": 1.75}
输入输出操作
print(f"Name: {name}, Age: {age}, Height: {height}")
print(f"Is student: {is_student}")
print(f"Scores: {scores}")
print(f"Info: {info}")
二、控制结构
Python提供了多种控制结构,包括条件语句、循环语句和异常处理。以下是示例代码:
# 条件语句
if age > 18:
print("Adult")
else:
print("Minor")
循环语句
for score in scores:
print(f"Score: {score}")
异常处理
try:
result = 10 / 0
except ZeroDivisionError:
print("Cannot divide by zero")
三、函数和模块
函数和模块是Python的核心特性之一。以下是一个简单的示例代码:
# 函数定义
def greet(name):
return f"Hello, {name}!"
调用函数
message = greet("Alice")
print(message)
导入模块
import math
使用模块中的函数
print(f"Square root of 16 is {math.sqrt(16)}")
四、面向对象编程
Python支持面向对象编程,可以定义类和创建对象。以下是示例代码:
# 类定义
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def introduce(self):
return f"My name is {self.name}, and I am {self.age} years old."
创建对象
person = Person("Alice", 30)
调用对象的方法
print(person.introduce())
五、文件操作
Python提供了强大的文件操作功能,可以读取和写入文件。以下是示例代码:
# 写入文件
with open("example.txt", "w") as file:
file.write("Hello, World!\n")
file.write("This is a test file.")
读取文件
with open("example.txt", "r") as file:
content = file.read()
print(content)
六、综合示例
为了更好地理解Python的各个方面,我们可以将上述内容综合到一个完整的示例代码中:
# 综合示例代码
import math
变量定义
name = "Alice"
age = 30
height = 1.75
is_student = False
scores = [85, 90, 78, 92]
info = {"name": "Alice", "age": 30, "height": 1.75}
函数定义
def greet(name):
return f"Hello, {name}!"
类定义
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def introduce(self):
return f"My name is {self.name}, and I am {self.age} years old."
创建对象
person = Person(name, age)
输入输出操作
print(f"Name: {name}, Age: {age}, Height: {height}")
print(f"Is student: {is_student}")
print(f"Scores: {scores}")
print(f"Info: {info}")
调用函数
message = greet(name)
print(message)
调用对象的方法
print(person.introduce())
条件语句
if age > 18:
print("Adult")
else:
print("Minor")
循环语句
for score in scores:
print(f"Score: {score}")
异常处理
try:
result = 10 / 0
except ZeroDivisionError:
print("Cannot divide by zero")
使用模块中的函数
print(f"Square root of 16 is {math.sqrt(16)}")
写入文件
with open("example.txt", "w") as file:
file.write("Hello, World!\n")
file.write("This is a test file.")
读取文件
with open("example.txt", "r") as file:
content = file.read()
print(content)
通过以上综合示例代码,我们可以看到Python的基础语法、控制结构、函数和模块、面向对象编程以及文件操作的实际应用。这种方式不仅有助于初学者快速了解Python的各个方面,还能通过实际操作加深理解和掌握。
七、深入理解与实践
1、Python的内置数据结构
Python提供了多种内置的数据结构,如列表、元组、集合和字典。这些数据结构在处理数据时非常方便。以下是示例代码:
# 列表
fruits = ["apple", "banana", "cherry"]
fruits.append("orange")
print(fruits)
元组
coordinates = (10, 20)
print(coordinates)
集合
unique_numbers = {1, 2, 3, 4, 4, 5}
print(unique_numbers)
字典
student = {"name": "Alice", "age": 30, "scores": [85, 90, 78]}
print(student)
2、列表推导式
列表推导式是Python的一种简洁语法,用于生成新的列表。以下是示例代码:
# 使用列表推导式生成平方数列表
squares = [x 2 for x in range(10)]
print(squares)
3、生成器
生成器是一种特殊的迭代器,用于生成序列。以下是示例代码:
# 生成器函数
def fibonacci(n):
a, b = 0, 1
for _ in range(n):
yield a
a, b = b, a + b
使用生成器生成斐波那契数列
for num in fibonacci(10):
print(num)
4、装饰器
装饰器是一种高级特性,用于在不修改原函数的情况下扩展其功能。以下是示例代码:
# 定义装饰器函数
def logger(func):
def wrapper(*args, kwargs):
print(f"Calling function {func.__name__} with arguments {args} and {kwargs}")
result = func(*args, kwargs)
print(f"Function {func.__name__} returned {result}")
return result
return wrapper
使用装饰器
@logger
def add(a, b):
return a + b
调用装饰器函数
print(add(3, 5))
5、多线程与多进程
Python支持多线程和多进程编程,以提高程序的并发性能。以下是示例代码:
import threading
import multiprocessing
线程函数
def thread_task(name):
print(f"Thread {name} is running")
创建并启动线程
threads = []
for i in range(5):
t = threading.Thread(target=thread_task, args=(i,))
threads.append(t)
t.start()
进程函数
def process_task(name):
print(f"Process {name} is running")
创建并启动进程
processes = []
for i in range(5):
p = multiprocessing.Process(target=process_task, args=(i,))
processes.append(p)
p.start()
八、总结
通过以上内容,我们可以看到Python的基础语法、控制结构、函数和模块、面向对象编程、文件操作、内置数据结构、列表推导式、生成器、装饰器以及多线程与多进程的实际应用。这些内容不仅涵盖了Python的各个方面,还通过实际示例帮助读者更好地理解和掌握Python编程。
九、实践与应用
在实际项目中,Python的应用非常广泛,包括数据分析、机器学习、Web开发、自动化脚本等。以下是一些示例项目:
1、数据分析
使用Pandas和Matplotlib库进行数据分析和可视化:
import pandas as pd
import matplotlib.pyplot as plt
加载数据
data = pd.read_csv("data.csv")
数据分析
print(data.describe())
数据可视化
data.plot(kind="bar")
plt.show()
2、机器学习
使用Scikit-Learn库进行机器学习模型的训练和预测:
from sklearn.datasets import load_iris
from sklearn.model_selection import train_test_split
from sklearn.ensemble import RandomForestClassifier
from sklearn.metrics import accuracy_score
加载数据
iris = load_iris()
X = iris.data
y = iris.target
划分训练集和测试集
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
训练模型
model = RandomForestClassifier()
model.fit(X_train, y_train)
预测
y_pred = model.predict(X_test)
评估模型
accuracy = accuracy_score(y_test, y_pred)
print(f"Accuracy: {accuracy}")
3、Web开发
使用Flask框架开发一个简单的Web应用:
from flask import Flask, render_template
app = Flask(__name__)
@app.route('/')
def home():
return render_template('index.html')
if __name__ == '__main__':
app.run(debug=True)
4、自动化脚本
编写一个自动化脚本,定时备份文件:
import shutil
import os
import time
source_dir = "/path/to/source"
backup_dir = "/path/to/backup"
while True:
for filename in os.listdir(source_dir):
source_file = os.path.join(source_dir, filename)
backup_file = os.path.join(backup_dir, filename)
shutil.copy(source_file, backup_file)
print("Backup completed")
time.sleep(86400) # 每天备份一次
十、继续学习与提升
Python的学习之路是一个持续的过程。通过不断实践和应用,提升编程能力。以下是一些建议:
1、阅读官方文档
Python的官方文档是学习Python的最佳资源,包含了详细的语法说明和示例代码。
2、参与开源项目
通过参与开源项目,可以接触到真实的项目需求和代码规范,提升编程能力。
3、参加编程比赛
参加编程比赛,如LeetCode、Kaggle等,可以提高算法和数据结构的理解和应用能力。
4、持续学习新技术
Python的生态系统非常丰富,不断学习新技术,如机器学习、深度学习、数据分析等,可以拓展应用领域。
通过以上内容的学习和实践,相信读者可以快速掌握Python编程,并在实际项目中应用所学知识。Python不仅是一门简单易学的编程语言,还是一个强大的工具,可以解决各种实际问题,提升工作效率。
相关问答FAQs:
如何通过简单的代码示例学习Python编程语言?
可以从一些基础的代码示例开始,例如打印“Hello, World!”。这段代码不仅简单易懂,而且是学习任何编程语言的传统入门示例。接下来,可以尝试编写一些简单的数学运算,使用变量和数据类型,逐步深入了解Python的基本语法和功能。
有哪些在线资源可以帮助我更深入地理解Python代码?
网络上有许多优秀的学习平台和社区,例如Codecademy、Coursera和W3Schools等。这些平台提供了互动教程和实际编码练习,让你能够在实践中学习Python。此外,GitHub上也有许多开源项目,你可以通过阅读他人的代码来提升自己的理解能力。
我应该如何选择合适的Python学习项目来提高我的编程技能?
选择学习项目时,可以从自己的兴趣出发,比如开发小游戏、自动化日常任务或数据分析等。确保项目的难度适合你的当前水平,并能逐步挑战自己。通过完成实际项目,你不仅能巩固所学知识,还能增强解决实际问题的能力。
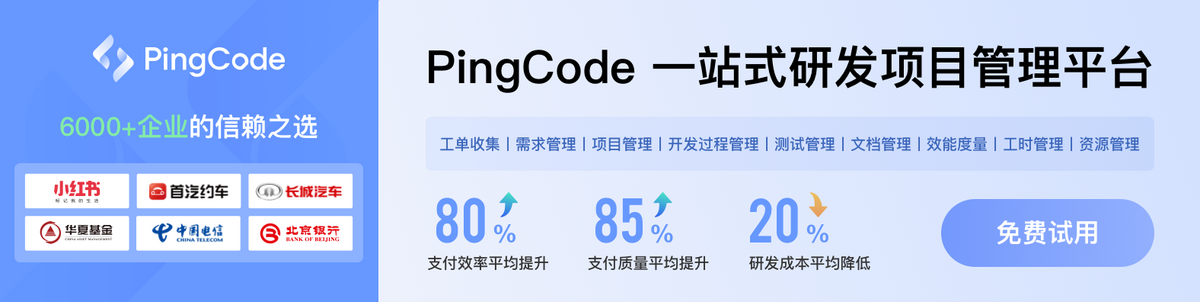