Python如何向ES的索引添加类型
在Python中向ES(Elasticsearch)的索引添加类型的方法有:创建索引并定义映射、使用Elasticsearch的Python客户端、通过PUT映射到现有索引、确保数据类型的一致性。 其中,创建索引并定义映射是非常重要的一步,因为它确保了索引中每个字段的数据类型和结构是正确的。
要详细描述这一点,首先需要理解Elasticsearch的索引和映射。索引相当于数据库,映射则相当于数据库中的表结构。通过定义映射,可以确保每个字段的数据类型和分析方法是适当的。例如,可以为一个字符串字段指定不同的分析器,以便更好地处理文本数据。以下是详细步骤和代码示例。
一、创建索引并定义映射
在向Elasticsearch添加数据之前,通常需要先创建索引并定义字段的映射。这确保了Elasticsearch知道如何处理和存储数据。可以使用Elasticsearch的Python客户端(Elasticsearch-py)来完成这项任务。
from elasticsearch import Elasticsearch
连接Elasticsearch服务器
es = Elasticsearch(['http://localhost:9200'])
定义索引名称
index_name = 'my_index'
定义映射
mapping = {
"mappings": {
"properties": {
"title": {"type": "text"},
"author": {"type": "keyword"},
"published_date": {"type": "date"},
"content": {"type": "text"}
}
}
}
创建索引并应用映射
if not es.indices.exists(index=index_name):
es.indices.create(index=index_name, body=mapping)
在上述代码中,我们首先连接到Elasticsearch服务器,然后定义索引名称和映射结构。映射结构中包含了索引中每个字段的类型,如text
、keyword
、date
等。接着,我们检查索引是否存在,如果不存在则创建索引并应用映射。
二、使用Elasticsearch的Python客户端
Elasticsearch的Python客户端(Elasticsearch-py)是与Elasticsearch交互的官方库。它提供了一系列方法来执行索引创建、数据插入、查询等操作。
from elasticsearch import Elasticsearch
连接Elasticsearch服务器
es = Elasticsearch(['http://localhost:9200'])
数据
document = {
"title": "Elasticsearch Guide",
"author": "John Doe",
"published_date": "2023-01-01",
"content": "This is a comprehensive guide to Elasticsearch."
}
插入数据到索引
es.index(index="my_index", document=document)
在这段代码中,我们插入了一条数据到my_index
索引中。Elasticsearch会根据之前定义的映射来处理和存储数据。
三、通过PUT映射到现有索引
如果已经有一个索引,并且需要更新其映射,可以使用PUT请求来添加或修改映射。
from elasticsearch import Elasticsearch
连接Elasticsearch服务器
es = Elasticsearch(['http://localhost:9200'])
定义新的映射
new_mapping = {
"properties": {
"summary": {"type": "text"}
}
}
更新现有索引的映射
es.indices.put_mapping(index="my_index", body=new_mapping)
在这个例子中,我们为现有的my_index
索引添加了一个新的字段summary
,其类型为text
。这种操作允许在不删除索引的情况下动态地扩展其结构。
四、确保数据类型的一致性
在使用Elasticsearch时,确保数据的一致性非常重要。映射定义了字段的数据类型,如果插入的数据类型与映射不匹配,Elasticsearch会抛出错误。因此,在数据插入之前,应该确保数据的类型和结构与映射定义一致。
def validate_document(document, mapping):
for field, field_type in mapping["mappings"]["properties"].items():
if field in document:
if not isinstance(document[field], get_python_type(field_type["type"])):
raise ValueError(f"Field {field} should be of type {field_type['type']}")
def get_python_type(es_type):
type_mapping = {
"text": str,
"keyword": str,
"date": str,
"integer": int,
"float": float,
"boolean": bool
}
return type_mapping.get(es_type, str)
示例文档
document = {
"title": "Elasticsearch Guide",
"author": "John Doe",
"published_date": "2023-01-01",
"content": "This is a comprehensive guide to Elasticsearch."
}
验证文档
validate_document(document, mapping)
上述代码中,我们定义了一个函数validate_document
来验证文档的数据类型是否与映射一致。通过这种方式,可以在数据插入之前进行检查,避免潜在的数据不一致问题。
五、示例项目:从头开始创建索引并添加数据
为了更好地理解这些步骤,我们可以通过一个完整的示例项目来展示如何从头开始创建索引、定义映射并添加数据。
from elasticsearch import Elasticsearch
连接Elasticsearch服务器
es = Elasticsearch(['http://localhost:9200'])
定义索引名称
index_name = 'library'
定义映射
mapping = {
"mappings": {
"properties": {
"book_title": {"type": "text"},
"author": {"type": "keyword"},
"publish_date": {"type": "date"},
"summary": {"type": "text"},
"isbn": {"type": "keyword"}
}
}
}
创建索引并应用映射
if not es.indices.exists(index=index_name):
es.indices.create(index=index_name, body=mapping)
示例数据
books = [
{
"book_title": "Learn Python Programming",
"author": "Fabrizio Romano",
"publish_date": "2018-04-27",
"summary": "A practical introduction to Python programming.",
"isbn": "9781788996662"
},
{
"book_title": "Elasticsearch: The Definitive Guide",
"author": "Clinton Gormley",
"publish_date": "2015-02-07",
"summary": "A comprehensive guide to Elasticsearch.",
"isbn": "9781449358549"
}
]
插入数据到索引
for book in books:
es.index(index=index_name, document=book)
在这个示例项目中,我们创建了一个名为library
的索引,并定义了映射结构。然后,我们插入了两本书的数据到索引中。通过这种方式,可以确保数据在插入之前已经正确定义了其结构和类型。
综上所述,向Elasticsearch的索引添加类型的关键步骤包括创建索引并定义映射、使用Elasticsearch的Python客户端插入数据、通过PUT请求更新映射以及确保数据类型的一致性。这些步骤可以帮助您在使用Elasticsearch时更好地管理和查询数据。
相关问答FAQs:
如何在Elasticsearch的索引中定义和添加数据类型?
在Elasticsearch中,数据类型的定义通常在索引的映射(mapping)中进行。您可以使用REST API来创建索引时指定映射,或者在索引创建后更新映射。对于Python用户,可以使用elasticsearch-py
库来实现这一过程。要添加数据类型,您可以在创建索引时提供一个JSON对象,定义每个字段的数据类型。例如,可以定义字符串、数字、日期等类型。
使用Python与Elasticsearch交互时需要注意哪些问题?
在使用Python与Elasticsearch进行交互时,确保使用兼容的elasticsearch-py
库版本,并遵循Elasticsearch的API规范。此外,要注意索引的创建和映射的设置需要在数据插入之前完成,防止因数据类型不匹配导致的错误。合理利用异常处理机制,可以提高代码的健壮性。
如何验证向Elasticsearch索引添加的数据类型是否正确?
可以通过Elasticsearch的GET
请求获取索引的映射信息,以验证数据类型是否正确。使用Python时,您可以调用相应的API,通过elasticsearch-py
库中的indices.get_mapping()
方法来获取当前索引的映射信息,并进行对比检查,确保添加的数据类型与预期一致。
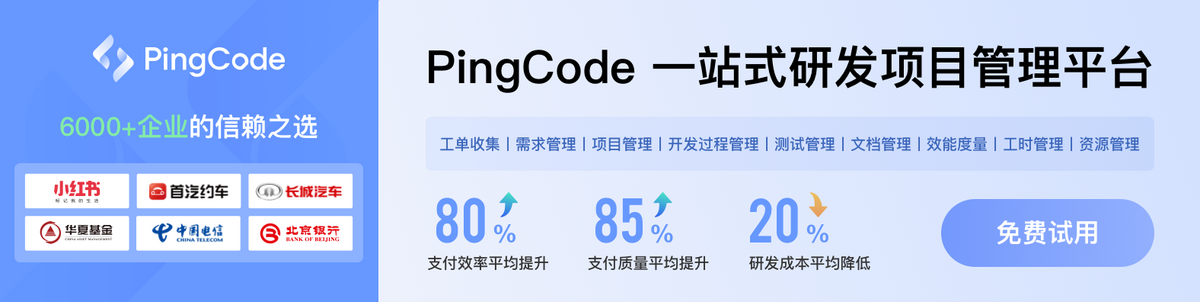