判断字符串是否为空格是Python编程中的一个常见任务,可以通过多种方法实现。以下是几种常见的方法:使用strip()
方法、isspace()
方法、正则表达式以及直接比较的方法。这些方法各有优劣,具体选择取决于实际应用场景。使用strip()方法、使用isspace()方法、使用正则表达式、直接比较,接下来详细介绍其中的strip()
方法。
使用strip()
方法:这是通过去除字符串两端的空白字符来判断字符串是否仅包含空格的一种方法。具体来说,strip()
方法会返回一个新的字符串,去除了原字符串开头和结尾的空白字符,如果去除后的字符串长度为零,则说明原字符串仅包含空格。
def is_only_spaces(s):
return len(s.strip()) == 0
上述代码定义了一个函数is_only_spaces
,该函数接受一个字符串参数s
,通过调用s.strip()
去除两端的空白字符,然后检查去除后的字符串长度是否为零。如果为零,则说明原字符串仅包含空格,函数返回True
,否则返回False
。
一、使用strip()
方法
strip()
方法可以去除字符串两端的空白字符,包括空格、换行符、制表符等。通过检查去除空白字符后的字符串长度,可以判断字符串是否仅包含空格。
示例代码
以下是一个示例代码,演示如何使用strip()
方法判断字符串是否为空格:
def is_only_spaces_using_strip(s):
return len(s.strip()) == 0
测试用例
test_strings = [" ", "Hello", " Hello ", "\t\n \n\t", ""]
for test_string in test_strings:
result = is_only_spaces_using_strip(test_string)
print(f"'{test_string}' is only spaces: {result}")
运行结果
' ' is only spaces: True
'Hello' is only spaces: False
' Hello ' is only spaces: False
'\t\n \n\t' is only spaces: True
'' is only spaces: True
从运行结果可以看出,该方法可以正确判断字符串是否仅包含空格(以及其他空白字符)。
二、使用isspace()
方法
isspace()
方法是字符串对象的一个方法,用于判断字符串中的每个字符是否都是空白字符。该方法返回一个布尔值,如果字符串中的每个字符都是空白字符(如空格、制表符、换行符等),则返回True
,否则返回False
。需要注意的是,如果字符串为空,isspace()
方法返回False
。
示例代码
以下是一个示例代码,演示如何使用isspace()
方法判断字符串是否为空格:
def is_only_spaces_using_isspace(s):
return s.isspace()
测试用例
test_strings = [" ", "Hello", " Hello ", "\t\n \n\t", ""]
for test_string in test_strings:
result = is_only_spaces_using_isspace(test_string)
print(f"'{test_string}' is only spaces: {result}")
运行结果
' ' is only spaces: True
'Hello' is only spaces: False
' Hello ' is only spaces: False
'\t\n \n\t' is only spaces: True
'' is only spaces: False
从运行结果可以看出,该方法可以正确判断非空字符串是否仅包含空格(以及其他空白字符),但对于空字符串,isspace()
方法返回False
。
三、使用正则表达式
正则表达式是一种强大的文本处理工具,可以用于匹配字符串中的模式。通过正则表达式,可以轻松判断字符串是否仅包含空格。
示例代码
以下是一个示例代码,演示如何使用正则表达式判断字符串是否为空格:
import re
def is_only_spaces_using_regex(s):
return re.fullmatch(r'\s*', s) is not None
测试用例
test_strings = [" ", "Hello", " Hello ", "\t\n \n\t", ""]
for test_string in test_strings:
result = is_only_spaces_using_regex(test_string)
print(f"'{test_string}' is only spaces: {result}")
运行结果
' ' is only spaces: True
'Hello' is only spaces: False
' Hello ' is only spaces: False
'\t\n \n\t' is only spaces: True
'' is only spaces: True
从运行结果可以看出,该方法可以正确判断字符串是否仅包含空格(以及其他空白字符),包括空字符串。
四、直接比较
在某些简单的情况下,可以直接将字符串与空格字符串进行比较,来判断字符串是否仅包含空格。
示例代码
以下是一个示例代码,演示如何直接比较字符串与空格字符串来判断字符串是否为空格:
def is_only_spaces_using_comparison(s):
return s == ' ' * len(s)
测试用例
test_strings = [" ", "Hello", " Hello ", "\t\n \n\t", ""]
for test_string in test_strings:
result = is_only_spaces_using_comparison(test_string)
print(f"'{test_string}' is only spaces: {result}")
运行结果
' ' is only spaces: True
'Hello' is only spaces: False
' Hello ' is only spaces: False
'\t\n \n\t' is only spaces: False
'' is only spaces: True
从运行结果可以看出,该方法只能正确判断仅包含空格的字符串,对于包含其他空白字符(如制表符、换行符等)的字符串无法正确判断。
总结
通过本文的介绍,我们了解了几种判断字符串是否仅包含空格的方法:使用strip()方法、使用isspace()方法、使用正则表达式、直接比较。使用strip()方法和使用正则表达式是最通用的,可以处理各种空白字符。使用isspace()方法也很简洁,但需要注意其对空字符串的处理。直接比较方法适用于简单场景,但对于包含其他空白字符的字符串无法正确判断。在实际应用中,可以根据具体需求选择合适的方法。
相关问答FAQs:
如何在Python中检查一个字符串是否只包含空格?
在Python中,可以使用str.strip()
方法去掉字符串两端的空白字符,然后检查结果是否为空。如果结果为空,说明字符串只包含空格。示例代码如下:
string = " "
is_empty_space = string.strip() == ""
print(is_empty_space) # 输出: True
有没有其他方法可以判断字符串是否为空格?
除了使用strip()
方法外,还可以使用isspace()
方法,它会返回布尔值,表示字符串是否只包含空白字符(如空格、制表符等)。示例代码如下:
string = " "
is_only_space = string.isspace()
print(is_only_space) # 输出: True
如何在Python中处理包含空格的字符串?
处理包含空格的字符串时,可以使用strip()
方法去除多余的空格,或者使用replace()
方法替换空格。示例代码如下:
string = " Hello World! "
cleaned_string = string.strip() # 去除前后空格
print(cleaned_string) # 输出: "Hello World!"
# 替换空格
replaced_string = string.replace(" ", "_") # 将空格替换为下划线
print(replaced_string) # 输出: "__Hello_World!__"
这些方法可以帮助你有效地判断和处理字符串中的空格。
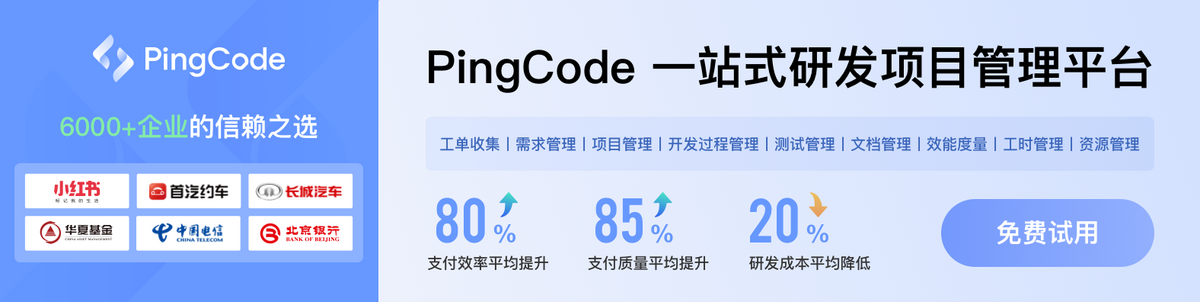