Python里面的配置文件如何读取
Python里面的配置文件读取有几种常见方法:使用configparser模块、使用json模块、使用yaml模块。其中,configparser模块是最常用的,它用于读取.ini格式的配置文件。它能够将配置文件中的各个部分解析为字典,并且支持默认值、插值等高级特性。下面将详细介绍如何使用configparser模块读取配置文件。
一、CONFIGPARSER模块
1、安装和导入CONFIGPARSER模块
ConfigParser模块是Python标准库的一部分,因此不需要额外安装。可以直接使用以下代码导入:
import configparser
2、创建配置文件
首先,需要创建一个.ini格式的配置文件,例如config.ini:
[DEFAULT]
ServerAliveInterval = 45
Compression = yes
CompressionLevel = 9
[bitbucket.org]
User = hg
[topsecret.server.com]
Port = 50022
ForwardX11 = no
3、读取配置文件
使用ConfigParser读取配置文件并获取配置项:
import configparser
创建ConfigParser对象
config = configparser.ConfigParser()
读取配置文件
config.read('config.ini')
获取默认部分的配置项
server_alive_interval = config['DEFAULT']['ServerAliveInterval']
compression = config['DEFAULT']['Compression']
compression_level = config['DEFAULT']['CompressionLevel']
获取特定部分的配置项
user = config['bitbucket.org']['User']
port = config['topsecret.server.com']['Port']
forward_x11 = config['topsecret.server.com']['ForwardX11']
print(f"ServerAliveInterval: {server_alive_interval}")
print(f"Compression: {compression}")
print(f"CompressionLevel: {compression_level}")
print(f"User: {user}")
print(f"Port: {port}")
print(f"ForwardX11: {forward_x11}")
4、修改和保存配置文件
ConfigParser还支持修改和保存配置文件:
# 修改配置项
config['DEFAULT']['ServerAliveInterval'] = '50'
config['bitbucket.org']['User'] = 'new_user'
将修改后的配置写回文件
with open('config.ini', 'w') as configfile:
config.write(configfile)
二、JSON模块
1、安装和导入JSON模块
JSON模块也是Python标准库的一部分,可以直接导入:
import json
2、创建配置文件
创建一个.json格式的配置文件,例如config.json:
{
"DEFAULT": {
"ServerAliveInterval": 45,
"Compression": "yes",
"CompressionLevel": 9
},
"bitbucket.org": {
"User": "hg"
},
"topsecret.server.com": {
"Port": 50022,
"ForwardX11": "no"
}
}
3、读取配置文件
使用JSON模块读取配置文件:
import json
读取配置文件
with open('config.json', 'r') as f:
config = json.load(f)
获取默认部分的配置项
server_alive_interval = config['DEFAULT']['ServerAliveInterval']
compression = config['DEFAULT']['Compression']
compression_level = config['DEFAULT']['CompressionLevel']
获取特定部分的配置项
user = config['bitbucket.org']['User']
port = config['topsecret.server.com']['Port']
forward_x11 = config['topsecret.server.com']['ForwardX11']
print(f"ServerAliveInterval: {server_alive_interval}")
print(f"Compression: {compression}")
print(f"CompressionLevel: {compression_level}")
print(f"User: {user}")
print(f"Port: {port}")
print(f"ForwardX11: {forward_x11}")
4、修改和保存配置文件
JSON模块也支持修改和保存配置文件:
# 修改配置项
config['DEFAULT']['ServerAliveInterval'] = 50
config['bitbucket.org']['User'] = 'new_user'
将修改后的配置写回文件
with open('config.json', 'w') as f:
json.dump(config, f, indent=4)
三、YAML模块
1、安装和导入YAML模块
YAML模块不是Python标准库的一部分,需要通过pip安装:
pip install pyyaml
导入YAML模块:
import yaml
2、创建配置文件
创建一个.yaml格式的配置文件,例如config.yaml:
DEFAULT:
ServerAliveInterval: 45
Compression: yes
CompressionLevel: 9
bitbucket.org:
User: hg
topsecret.server.com:
Port: 50022
ForwardX11: no
3、读取配置文件
使用YAML模块读取配置文件:
import yaml
读取配置文件
with open('config.yaml', 'r') as f:
config = yaml.safe_load(f)
获取默认部分的配置项
server_alive_interval = config['DEFAULT']['ServerAliveInterval']
compression = config['DEFAULT']['Compression']
compression_level = config['DEFAULT']['CompressionLevel']
获取特定部分的配置项
user = config['bitbucket.org']['User']
port = config['topsecret.server.com']['Port']
forward_x11 = config['topsecret.server.com']['ForwardX11']
print(f"ServerAliveInterval: {server_alive_interval}")
print(f"Compression: {compression}")
print(f"CompressionLevel: {compression_level}")
print(f"User: {user}")
print(f"Port: {port}")
print(f"ForwardX11: {forward_x11}")
4、修改和保存配置文件
YAML模块也支持修改和保存配置文件:
# 修改配置项
config['DEFAULT']['ServerAliveInterval'] = 50
config['bitbucket.org']['User'] = 'new_user'
将修改后的配置写回文件
with open('config.yaml', 'w') as f:
yaml.safe_dump(config, f, default_flow_style=False)
四、总结
在Python中,读取配置文件有多种方法。configparser模块适用于.ini格式的配置文件,json模块适用于.json格式的配置文件,yaml模块适用于.yaml格式的配置文件。根据实际需求选择合适的模块和格式,以便更好地管理和读取配置文件。无论使用哪种方法,都能够方便地读取、修改和保存配置文件,从而提高程序的灵活性和可维护性。
相关问答FAQs:
如何在Python中读取不同格式的配置文件?
Python支持多种格式的配置文件,包括INI、JSON、YAML和XML等。对于INI文件,可以使用configparser
模块;对于JSON文件,Python的标准库中提供了json
模块;YAML文件通常使用PyYAML
库,而XML文件则可以通过xml.etree.ElementTree
来解析。选择合适的库和格式可以提高代码的可读性和可维护性。
在读取配置文件时,如何处理文件路径问题?
确保文件路径正确是读取配置文件的重要步骤。可以使用Python的os
模块来构建文件路径,使用os.path.join
来确保路径的正确性。此外,通过os.path.exists
可以检查文件是否存在,以避免因路径错误导致的异常。
配置文件中如何使用注释,以便于后续维护?
在大多数配置文件格式中,都可以通过特定的符号添加注释,例如在INI文件中,使用分号(;)或井号(#)来添加注释。JSON文件不支持注释,YAML文件则使用#符号。注释可以帮助开发者理解配置项的用途,增强可维护性。
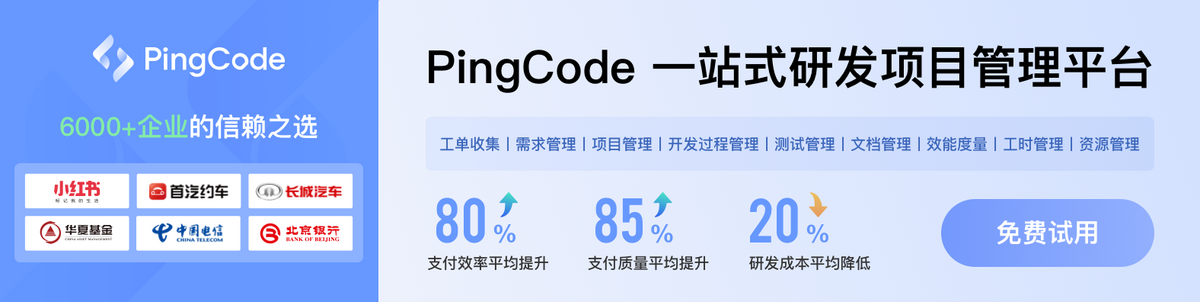