在Python中,使用os库并不是下载文件的最佳选择。更推荐使用的库是requests、urllib或wget,但可以结合os库来处理文件的存放路径、创建目录等操作。下面,我们将详细介绍如何使用这些库下载文件,并结合os库进行文件处理。
一、使用requests库下载文件
requests库是Python中最常用的HTTP库,非常适合用于下载文件。
1.1 安装requests库
首先,你需要安装requests库。如果你还没有安装它,可以使用pip进行安装:
pip install requests
1.2 下载文件并保存
import os
import requests
def download_file(url, save_path):
response = requests.get(url, stream=True)
if response.status_code == 200:
with open(save_path, 'wb') as file:
for chunk in response.iter_content(chunk_size=1024):
file.write(chunk)
print(f"File downloaded successfully and saved to {save_path}")
else:
print(f"Failed to download file. Status code: {response.status_code}")
Example usage
url = 'https://example.com/file.zip'
save_path = os.path.join('downloads', 'file.zip')
os.makedirs(os.path.dirname(save_path), exist_ok=True)
download_file(url, save_path)
在这段代码中:
- requests.get:用于发送HTTP GET请求。
- response.iter_content:以流的方式下载文件,避免占用过多内存。
- os.path.join 和 os.makedirs:用于处理文件路径和创建目录。
二、使用urllib库下载文件
urllib库是Python自带的标准库之一,功能强大且无需额外安装。
2.1 下载文件并保存
import os
import urllib.request
def download_file(url, save_path):
try:
urllib.request.urlretrieve(url, save_path)
print(f"File downloaded successfully and saved to {save_path}")
except Exception as e:
print(f"Failed to download file. Error: {e}")
Example usage
url = 'https://example.com/file.zip'
save_path = os.path.join('downloads', 'file.zip')
os.makedirs(os.path.dirname(save_path), exist_ok=True)
download_file(url, save_path)
在这段代码中:
- urllib.request.urlretrieve:直接下载文件并保存。
三、使用wget库下载文件
wget库是一个用于下载文件的小工具,非常易用。
3.1 安装wget库
首先,你需要安装wget库:
pip install wget
3.2 下载文件并保存
import os
import wget
def download_file(url, save_path):
try:
wget.download(url, save_path)
print(f"\nFile downloaded successfully and saved to {save_path}")
except Exception as e:
print(f"Failed to download file. Error: {e}")
Example usage
url = 'https://example.com/file.zip'
save_path = os.path.join('downloads', 'file.zip')
os.makedirs(os.path.dirname(save_path), exist_ok=True)
download_file(url, save_path)
在这段代码中:
- wget.download:用于下载文件并保存。
四、结合os库进行文件处理
除了下载文件之外,os库在处理文件路径和目录操作方面也非常有用。以下是一些常见的os库操作:
4.1 创建目录
import os
directory = 'downloads'
if not os.path.exists(directory):
os.makedirs(directory)
print(f"Directory {directory} created")
else:
print(f"Directory {directory} already exists")
4.2 检查文件是否存在
import os
file_path = 'downloads/file.zip'
if os.path.exists(file_path):
print(f"File {file_path} exists")
else:
print(f"File {file_path} does not exist")
4.3 删除文件
import os
file_path = 'downloads/file.zip'
if os.path.exists(file_path):
os.remove(file_path)
print(f"File {file_path} deleted")
else:
print(f"File {file_path} does not exist")
五、综合示例
结合以上内容,以下是一个完整的示例,展示了如何使用requests库下载文件,并结合os库进行文件和目录操作:
import os
import requests
def download_file(url, save_path):
response = requests.get(url, stream=True)
if response.status_code == 200:
with open(save_path, 'wb') as file:
for chunk in response.iter_content(chunk_size=1024):
file.write(chunk)
print(f"File downloaded successfully and saved to {save_path}")
else:
print(f"Failed to download file. Status code: {response.status_code}")
def main():
url = 'https://example.com/file.zip'
save_path = os.path.join('downloads', 'file.zip')
# Create directory if it doesn't exist
if not os.path.exists(os.path.dirname(save_path)):
os.makedirs(os.path.dirname(save_path))
print(f"Directory {os.path.dirname(save_path)} created")
# Check if file already exists
if os.path.exists(save_path):
print(f"File {save_path} already exists")
else:
download_file(url, save_path)
if __name__ == "__main__":
main()
在这个示例中:
- os.path.exists:检查目录和文件是否存在。
- os.makedirs:创建目录。
- requests.get:下载文件。
通过以上介绍,希望你能够更好地理解如何在Python中使用各种库下载文件,并结合os库进行文件和目录操作。
相关问答FAQs:
如何使用Python的os库下载文件?
os库本身并不提供直接下载文件的功能,它主要用于与操作系统交互。如果您想下载文件,通常需要结合其他库,例如requests库来进行网络请求。可以使用requests库下载文件,然后利用os库保存到本地文件系统。以下是一个简单的示例:
import requests
import os
url = 'http://example.com/file.zip'
response = requests.get(url)
# 确保文件夹存在
os.makedirs('downloads', exist_ok=True)
with open(os.path.join('downloads', 'file.zip'), 'wb') as f:
f.write(response.content)
os库在文件管理中有哪些重要的功能?
os库提供了多种功能,比如创建、删除、重命名文件或文件夹,获取文件路径等。通过os库,可以使用os.listdir()列出目录中的文件,使用os.remove()删除文件,以及使用os.rename()重命名文件。这些功能使得文件管理变得更加高效。
如何在Python中处理下载的文件?
下载文件后,可以使用os库中的功能对其进行处理。例如,使用os.path来检查文件是否存在,使用os.remove()来删除不需要的文件,或使用os.rename()来重命名文件。此外,还可以使用os.chdir()更改当前工作目录,以便在不同的目录中操作文件。
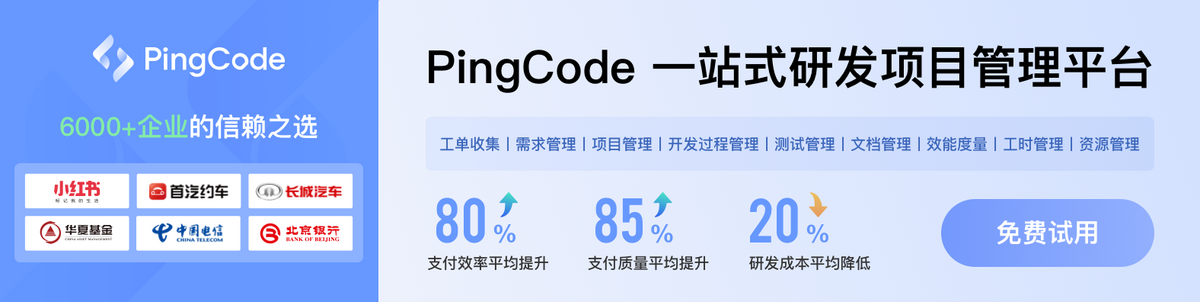