如何使用Python获取电脑的位置坐标
要使用Python获取电脑的位置坐标,可以使用geopy库、requests库、ipinfo.io API、Geocoder库。 其中,使用geopy库是一个比较常见的方法。下面我们将详细解释如何使用geopy库获取位置坐标。
一、安装所需库
首先,需要安装geopy库和requests库。可以使用以下命令进行安装:
pip install geopy
pip install requests
二、获取IP地址
为了获取电脑的地理位置,我们首先需要获取电脑的公网IP地址。可以使用requests库来获取IP地址。以下是代码示例:
import requests
def get_public_ip():
response = requests.get('https://api.ipify.org?format=json')
ip_address = response.json()['ip']
return ip_address
ip_address = get_public_ip()
print(f'Public IP Address: {ip_address}')
三、使用Geopy库获取位置坐标
使用geopy库来获取位置坐标。geopy提供了一个Nominatim工具,可以将地理名称转换为地理坐标(纬度和经度)。以下是代码示例:
from geopy.geocoders import Nominatim
def get_location_by_ip(ip_address):
geolocator = Nominatim(user_agent="geoapiExercises")
location = geolocator.geocode(ip_address)
return location
location = get_location_by_ip(ip_address)
print(f'Location: {location.address}')
print(f'Latitude: {location.latitude}, Longitude: {location.longitude}')
四、使用IPinfo.io API获取位置坐标
IPinfo.io是一个提供IP地址地理位置服务的API。可以通过该API获取详细的地理位置信息。以下是代码示例:
import requests
def get_location_from_ipinfo(ip_address):
response = requests.get(f'https://ipinfo.io/{ip_address}/json')
data = response.json()
location = data['loc'].split(',')
return {
'latitude': location[0],
'longitude': location[1],
'city': data.get('city'),
'region': data.get('region'),
'country': data.get('country')
}
location_info = get_location_from_ipinfo(ip_address)
print(f'City: {location_info["city"]}')
print(f'Region: {location_info["region"]}')
print(f'Country: {location_info["country"]}')
print(f'Latitude: {location_info["latitude"]}, Longitude: {location_info["longitude"]}')
五、使用Geocoder库获取位置坐标
Geocoder库是一个简单的地理编码库,可以将地址转换为地理坐标。以下是代码示例:
import geocoder
def get_location_by_geocoder(ip_address):
location = geocoder.ip(ip_address)
return {
'latitude': location.latlng[0],
'longitude': location.latlng[1],
'address': location.address
}
location_info = get_location_by_geocoder(ip_address)
print(f'Address: {location_info["address"]}')
print(f'Latitude: {location_info["latitude"]}, Longitude: {location_info["longitude"]}')
六、总结
通过上述方法,我们可以使用Python获取电脑的位置坐标。总结如下:
- 使用geopy库: 需要先获取IP地址,然后使用Nominatim工具获取位置坐标。
- 使用requests库: 获取电脑的公网IP地址。
- 使用IPinfo.io API: 通过IPinfo.io API获取详细的地理位置信息。
- 使用Geocoder库: 使用Geocoder库将IP地址转换为地理坐标。
无论使用哪种方法,都需要注意网络请求的可靠性和数据的准确性。在实际应用中,可以根据具体需求选择合适的方法来获取地理位置。
七、扩展内容
除了上述方法外,还有其他一些方法可以获取地理位置,例如使用Google Maps API、Here API等。以下是一个使用Google Maps API的示例:
import requests
def get_location_from_google_maps(api_key, address):
url = f'https://maps.googleapis.com/maps/api/geocode/json?address={address}&key={api_key}'
response = requests.get(url)
data = response.json()
if 'results' in data and len(data['results']) > 0:
location = data['results'][0]['geometry']['location']
return {
'latitude': location['lat'],
'longitude': location['lng'],
'address': data['results'][0]['formatted_address']
}
else:
return None
api_key = 'YOUR_GOOGLE_MAPS_API_KEY'
address = '1600 Amphitheatre Parkway, Mountain View, CA'
location_info = get_location_from_google_maps(api_key, address)
if location_info:
print(f'Address: {location_info["address"]}')
print(f'Latitude: {location_info["latitude"]}, Longitude: {location_info["longitude"]}')
else:
print('Location not found')
使用Google Maps API需要提供API密钥,可以通过Google Cloud Platform获取。
八、使用Here API获取位置坐标
Here API是另一个强大的地理位置服务API。以下是使用Here API获取位置坐标的示例:
import requests
def get_location_from_here(api_key, address):
url = f'https://geocode.search.hereapi.com/v1/geocode?q={address}&apiKey={api_key}'
response = requests.get(url)
data = response.json()
if 'items' in data and len(data['items']) > 0:
location = data['items'][0]['position']
return {
'latitude': location['lat'],
'longitude': location['lng'],
'address': data['items'][0]['address']['label']
}
else:
return None
api_key = 'YOUR_HERE_API_KEY'
address = '1600 Amphitheatre Parkway, Mountain View, CA'
location_info = get_location_from_here(api_key, address)
if location_info:
print(f'Address: {location_info["address"]}')
print(f'Latitude: {location_info["latitude"]}, Longitude: {location_info["longitude"]}')
else:
print('Location not found')
使用Here API同样需要提供API密钥,可以通过Here Developer Portal获取。
九、处理异常和错误
在实际应用中,网络请求可能会遇到各种异常和错误。为了保证程序的稳定性,需要对异常和错误进行处理。以下是一个示例:
import requests
from geopy.geocoders import Nominatim
import geocoder
def get_public_ip():
try:
response = requests.get('https://api.ipify.org?format=json')
response.raise_for_status()
ip_address = response.json()['ip']
return ip_address
except requests.RequestException as e:
print(f'Error getting public IP: {e}')
return None
def get_location_by_ip(ip_address):
try:
geolocator = Nominatim(user_agent="geoapiExercises")
location = geolocator.geocode(ip_address)
return location
except Exception as e:
print(f'Error getting location: {e}')
return None
def get_location_from_ipinfo(ip_address):
try:
response = requests.get(f'https://ipinfo.io/{ip_address}/json')
response.raise_for_status()
data = response.json()
location = data['loc'].split(',')
return {
'latitude': location[0],
'longitude': location[1],
'city': data.get('city'),
'region': data.get('region'),
'country': data.get('country')
}
except requests.RequestException as e:
print(f'Error getting location from IPinfo: {e}')
return None
def get_location_by_geocoder(ip_address):
try:
location = geocoder.ip(ip_address)
return {
'latitude': location.latlng[0],
'longitude': location.latlng[1],
'address': location.address
}
except Exception as e:
print(f'Error getting location by Geocoder: {e}')
return None
ip_address = get_public_ip()
if ip_address:
print(f'Public IP Address: {ip_address}')
location = get_location_by_ip(ip_address)
if location:
print(f'Location: {location.address}')
print(f'Latitude: {location.latitude}, Longitude: {location.longitude}')
location_info = get_location_from_ipinfo(ip_address)
if location_info:
print(f'City: {location_info["city"]}')
print(f'Region: {location_info["region"]}')
print(f'Country: {location_info["country"]}')
print(f'Latitude: {location_info["latitude"]}, Longitude: {location_info["longitude"]}')
location_info = get_location_by_geocoder(ip_address)
if location_info:
print(f'Address: {location_info["address"]}')
print(f'Latitude: {location_info["latitude"]}, Longitude: {location_info["longitude"]}')
通过上述示例,我们可以更好地处理网络请求中的异常和错误,保证程序的稳定性和可靠性。
十、总结与建议
在实际应用中,可以根据具体需求选择合适的方法来获取地理位置。以下是一些建议:
- 选择合适的API: 根据需求选择合适的地理位置服务API,例如Google Maps API、Here API等。
- 处理异常和错误: 在程序中处理网络请求的异常和错误,保证程序的稳定性。
- 优化性能: 如果需要频繁获取地理位置,可以考虑使用缓存等优化性能的方法。
希望通过本文的介绍,能够帮助读者更好地理解如何使用Python获取电脑的位置坐标,并在实际项目中灵活应用。
相关问答FAQs:
如何在Python中获取地理位置坐标的精确度?
获取地理位置坐标的精确度通常依赖于所使用的定位方法。使用GPS模块可以提供高精度的坐标,而通过IP地址获取的坐标则可能存在误差。为了提高精确度,可以结合多个数据源,例如使用GPS和Wi-Fi信息来交叉验证位置。
在Python中获取位置坐标需要哪些库?
获取电脑位置坐标常用的库包括geopy
、geocoder
和requests
等。geopy
用于地理编码和反向地理编码,而geocoder
则可以通过多种服务获取位置。requests
库可以帮助你通过API调用获取位置数据。
如何处理获取位置坐标时可能出现的错误?
在获取位置坐标的过程中,可能会遇到网络连接问题、API调用限制或权限不足等错误。处理这些错误的方法包括使用异常处理机制、检查网络连接状态以及确保程序具有必要的访问权限。此外,定期更新使用的库和API可以减少错误发生的频率。
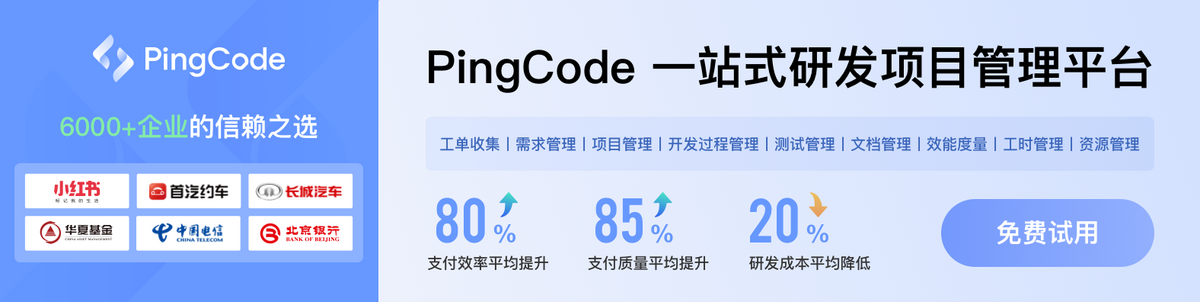