一、概述
Python向服务器发送数据的方式有多种,如使用HTTP请求、WebSocket协议、FTP协议等。其中,使用HTTP请求是最常见的方法,因为它简单且广泛支持。具体来说,可以使用requests
库来发送HTTP请求。接下来,我将详细介绍如何使用requests
库向服务器发送数据。
二、使用HTTP请求发送数据
- 安装和导入requests库
首先,需要安装并导入requests
库。requests
库是Python中非常流行的用于发送HTTP请求的库。通过以下命令安装:
pip install requests
然后在代码中导入:
import requests
- 发送GET请求
GET请求通常用于从服务器获取数据,但有时也需要在查询字符串中附带数据。以下是一个示例:
url = "http://example.com/api"
params = {"key1": "value1", "key2": "value2"}
response = requests.get(url, params=params)
print(response.text)
在上述代码中,params
参数是一个字典,其中包含要发送的数据。requests.get
函数会将这些数据添加到URL的查询字符串中,并发送GET请求。
- 发送POST请求
POST请求通常用于向服务器发送数据,例如表单数据或JSON数据。以下是一个示例:
url = "http://example.com/api"
data = {"key1": "value1", "key2": "value2"}
response = requests.post(url, data=data)
print(response.text)
在上述代码中,data
参数是一个字典,其中包含要发送的数据。requests.post
函数会将这些数据作为表单数据发送给服务器。
如果需要发送JSON数据,可以使用以下示例:
import json
url = "http://example.com/api"
data = {"key1": "value1", "key2": "value2"}
headers = {'Content-Type': 'application/json'}
response = requests.post(url, data=json.dumps(data), headers=headers)
print(response.text)
在上述代码中,json.dumps(data)
将字典转换为JSON字符串,并通过headers
参数指定内容类型为application/json
。
- 处理响应
发送请求后,可以通过response
对象获取服务器的响应。以下是一些常用的属性和方法:
response.status_code
:获取HTTP状态码。response.text
:获取响应内容(字符串)。response.json()
:将响应内容解析为JSON对象(如果内容是JSON格式)。
示例如下:
url = "http://example.com/api"
data = {"key1": "value1", "key2": "value2"}
response = requests.post(url, data=data)
if response.status_code == 200:
print("Request was successful")
print(response.json())
else:
print("Request failed with status code", response.status_code)
三、使用WebSocket协议发送数据
- 安装和导入websocket-client库
WebSocket是一种在单个TCP连接上进行全双工通信的协议。通过以下命令安装websocket-client
库:
pip install websocket-client
然后在代码中导入:
import websocket
- 发送数据
以下是一个使用WebSocket协议发送数据的示例:
def on_message(ws, message):
print("Received message:", message)
def on_error(ws, error):
print("Error occurred:", error)
def on_close(ws):
print("Connection closed")
def on_open(ws):
data = {"key1": "value1", "key2": "value2"}
ws.send(json.dumps(data))
url = "ws://example.com/websocket"
ws = websocket.WebSocketApp(url,
on_message=on_message,
on_error=on_error,
on_close=on_close)
ws.on_open = on_open
ws.run_forever()
在上述代码中,定义了四个回调函数on_message
、on_error
、on_close
和on_open
,用于处理WebSocket连接的不同事件。在on_open
函数中,通过ws.send
方法发送JSON数据。
四、使用FTP协议发送数据
- 安装和导入ftplib库
FTP(文件传输协议)是一种用于在网络上进行文件传输的协议。Python标准库中包含ftplib
库,可以通过以下代码导入:
from ftplib import FTP
- 发送文件
以下是一个使用FTP协议发送文件的示例:
ftp = FTP("ftp.example.com")
ftp.login("username", "password")
filename = "example.txt"
with open(filename, "rb") as file:
ftp.storbinary(f"STOR {filename}", file)
ftp.quit()
在上述代码中,首先连接到FTP服务器并登录,然后通过ftp.storbinary
方法将文件上传到服务器。
五、总结
Python提供了多种向服务器发送数据的方式,包括使用HTTP请求、WebSocket协议和FTP协议。通过requests
库可以方便地发送GET和POST请求,并处理服务器的响应。通过websocket-client
库可以实现WebSocket通信,并在单个TCP连接上进行全双工通信。通过标准库中的ftplib
库可以使用FTP协议进行文件传输。根据具体需求选择合适的方式,可以高效地实现数据传输。
相关问答FAQs:
如何使用Python发送HTTP请求向服务器传输数据?
在Python中,可以使用requests
库来发送HTTP请求并向服务器传输数据。通过使用requests.post()
方法,可以将数据以字典形式传递给服务器。例如:
import requests
url = 'http://example.com/api'
data = {'key1': 'value1', 'key2': 'value2'}
response = requests.post(url, json=data)
print(response.status_code)
print(response.json())
这样,数据将以JSON格式发送到指定的URL,并且可以处理服务器的响应。
Python支持哪些数据格式来发送到服务器?
Python可以通过多种数据格式向服务器发送数据,包括JSON、表单数据(form data)、XML等。使用requests
库时,可以通过设置headers
来指定数据格式,例如:
- JSON格式:使用
json=data
参数 - 表单数据:使用
data=data
参数 - XML格式:将XML字符串发送到服务器并设置相应的
Content-Type
头部信息。
在发送数据时如何处理服务器的响应?
发送数据到服务器后,通常需要处理服务器的响应。在使用requests
库时,可以通过response
对象来获取状态码、响应内容和头部信息。例如:
if response.status_code == 200:
print("数据发送成功:", response.json())
else:
print("错误发生,状态码:", response.status_code)
这样可以根据返回的状态码判断请求是否成功,并处理相应的结果。
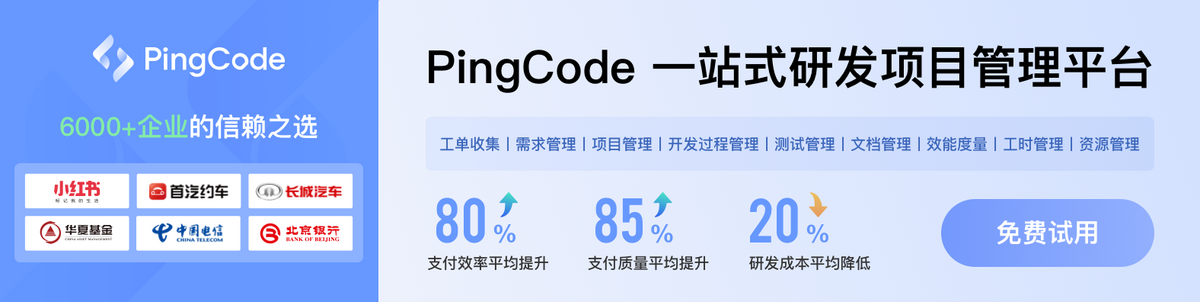