Python 显示上一条语录的方法有多种,常见的方法包括使用列表、文件存储、数据库存储等。通过列表存储、文件存储、数据库存储的方法,可以轻松实现显示上一条语录的功能。其中,使用列表是最简单的一种方式,适合处理少量数据。文件存储适合保存数据到本地,数据量较大时也能高效处理;数据库存储则适合处理大量数据和复杂查询需求。
一、使用列表
列表是一种非常适合存储多条语录的简单数据结构。在Python中,可以通过列表存储多个语录,并且可以方便地访问列表中的前一条语录。
1. 示例代码
以下是一个使用列表存储并显示上一条语录的示例代码:
quotes = [
"The greatest glory in living lies not in never falling, but in rising every time we fall. - Nelson Mandela",
"The way to get started is to quit talking and begin doing. - Walt Disney",
"Your time is limited, so don't waste it living someone else's life. - Steve Jobs",
"If life were predictable it would cease to be life, and be without flavor. - Eleanor Roosevelt",
"If you look at what you have in life, you'll always have more. - Oprah Winfrey"
]
def display_previous_quote(index):
if index <= 0:
print("No previous quote available.")
else:
print(quotes[index - 1])
current_index = 3 # Assuming we are currently at the 4th quote
display_previous_quote(current_index)
2. 代码详解
在这个示例中,quotes
列表存储了多个语录。函数 display_previous_quote
接受一个索引参数 index
,并检查是否有上一条语录。如果有,则打印上一条语录;否则,提示没有上一条语录。
二、使用文件存储
当需要在程序关闭后仍能保存语录时,可以将语录存储在文件中。这样可以实现持久化存储,并且可以在下次运行程序时继续使用。
1. 示例代码
以下是一个将语录存储在文件中,并显示上一条语录的示例代码:
# 保存语录到文件
def save_quotes_to_file(filename, quotes):
with open(filename, 'w') as file:
for quote in quotes:
file.write(quote + "\n")
读取文件中的语录
def load_quotes_from_file(filename):
quotes = []
with open(filename, 'r') as file:
for line in file:
quotes.append(line.strip())
return quotes
显示上一条语录
def display_previous_quote(quotes, index):
if index <= 0:
print("No previous quote available.")
else:
print(quotes[index - 1])
示例语录
quotes = [
"The greatest glory in living lies not in never falling, but in rising every time we fall. - Nelson Mandela",
"The way to get started is to quit talking and begin doing. - Walt Disney",
"Your time is limited, so don't waste it living someone else's life. - Steve Jobs",
"If life were predictable it would cease to be life, and be without flavor. - Eleanor Roosevelt",
"If you look at what you have in life, you'll always have more. - Oprah Winfrey"
]
filename = "quotes.txt"
save_quotes_to_file(filename, quotes)
loaded_quotes = load_quotes_from_file(filename)
current_index = 3 # Assuming we are currently at the 4th quote
display_previous_quote(loaded_quotes, current_index)
2. 代码详解
在这个示例中,函数 save_quotes_to_file
将语录保存到文件中,函数 load_quotes_from_file
从文件中读取语录。函数 display_previous_quote
接受语录列表和索引参数,功能与上一个示例类似。
三、使用数据库存储
当需要处理大量语录或进行复杂查询时,可以使用数据库存储语录。SQLite 是一个轻量级的嵌入式数据库,非常适合这种需求。
1. 示例代码
以下是一个使用 SQLite 存储语录,并显示上一条语录的示例代码:
import sqlite3
创建数据库连接
conn = sqlite3.connect('quotes.db')
cursor = conn.cursor()
创建表
cursor.execute('''CREATE TABLE IF NOT EXISTS quotes
(id INTEGER PRIMARY KEY AUTOINCREMENT,
quote TEXT NOT NULL)''')
插入语录
def insert_quote(quote):
cursor.execute("INSERT INTO quotes (quote) VALUES (?)", (quote,))
conn.commit()
获取所有语录
def get_all_quotes():
cursor.execute("SELECT * FROM quotes")
return cursor.fetchall()
显示上一条语录
def display_previous_quote(quotes, index):
if index <= 0:
print("No previous quote available.")
else:
print(quotes[index - 1][1])
示例语录
quotes = [
"The greatest glory in living lies not in never falling, but in rising every time we fall. - Nelson Mandela",
"The way to get started is to quit talking and begin doing. - Walt Disney",
"Your time is limited, so don't waste it living someone else's life. - Steve Jobs",
"If life were predictable it would cease to be life, and be without flavor. - Eleanor Roosevelt",
"If you look at what you have in life, you'll always have more. - Oprah Winfrey"
]
插入示例语录
for quote in quotes:
insert_quote(quote)
loaded_quotes = get_all_quotes()
current_index = 3 # Assuming we are currently at the 4th quote
display_previous_quote(loaded_quotes, current_index)
关闭数据库连接
conn.close()
2. 代码详解
在这个示例中,首先创建了一个 SQLite 数据库连接,并创建了一个 quotes
表。函数 insert_quote
用于插入语录,函数 get_all_quotes
用于获取所有语录。函数 display_previous_quote
接受语录列表和索引参数,功能与前两个示例类似。
四、总结
在Python中,可以使用列表、文件存储、数据库存储等多种方式显示上一条语录。 列表适合处理少量数据,文件存储适合保存数据到本地,数据库存储适合处理大量数据和复杂查询需求。根据实际需求选择合适的存储方式,可以高效地实现显示上一条语录的功能。
相关问答FAQs:
如何在Python中显示上一条语录?
在Python中,可以通过列表来存储多条语录,并使用索引来访问上一条语录。例如,您可以创建一个包含语录的列表,并通过减去一个索引值来获取上一条语录。以下是一个简单的示例:
quotes = ["第一条语录", "第二条语录", "第三条语录"]
current_index = 2 # 假设当前索引为2,表示第三条语录
previous_quote = quotes[current_index - 1] # 获取上一条语录
print(previous_quote)
这种方法非常直接,适合处理少量语录的情况。
我可以使用什么数据结构来管理多条语录?
除了列表,您还可以考虑使用字典或自定义类来管理语录。例如,字典可以通过键值对存储语录及其相关信息,方便检索和操作。如果需要更复杂的功能,自定义类将允许您添加方法来管理语录,增加灵活性和可读性。
如何在程序中实现随机显示语录并提供上一条的选项?
您可以使用random
模块随机选择一条语录,同时保留当前显示的索引,以便用户选择查看上一条。代码示例如下:
import random
quotes = ["第一条语录", "第二条语录", "第三条语录"]
current_index = random.randint(0, len(quotes) - 1)
current_quote = quotes[current_index]
print("当前语录:", current_quote)
# 提供查看上一条语录的选项
if current_index > 0:
previous_quote = quotes[current_index - 1]
print("上一条语录:", previous_quote)
else:
print("没有上一条语录。")
这种方式可以增加用户的互动性,提供更好的体验。
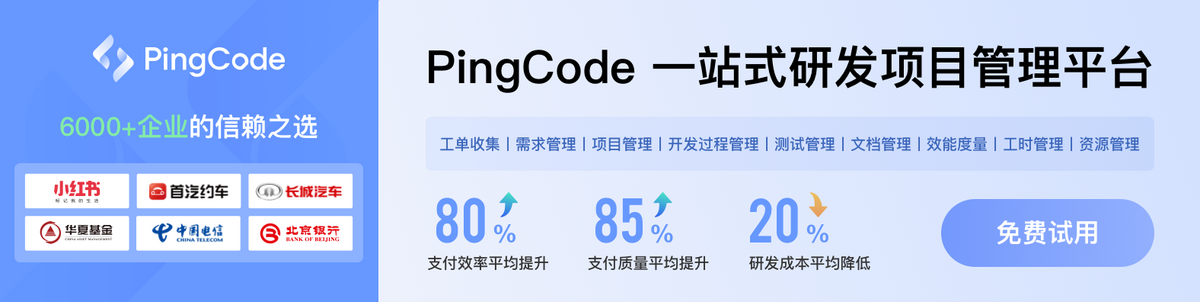