在Python脚本中执行ping命令有几种方式:使用subprocess模块、os模块、以及ping3库。 其中subprocess模块最常用,因为它提供了更多的控制和灵活性。以下是对subprocess模块的详细描述和代码示例。
一、使用subprocess模块
subprocess模块允许你生成新的进程、连接到它们的输入/输出/错误管道,并获取它们的返回码。它是执行系统命令(如ping)的强大工具。subprocess.run、subprocess.Popen是其中比较常用的方法。下面是一个详细的示例:
import subprocess
def ping(host):
# 使用subprocess.run执行ping命令
result = subprocess.run(['ping', '-c', '4', host], stdout=subprocess.PIPE, stderr=subprocess.PIPE, text=True)
if result.returncode == 0:
print(f"Ping to {host} successful:")
print(result.stdout)
else:
print(f"Ping to {host} failed:")
print(result.stderr)
if __name__ == "__main__":
host = "google.com"
ping(host)
在上述代码中,我们使用subprocess.run来执行ping命令,-c选项指定发送4个ICMP请求包。stdout、stderr参数用于捕获命令的标准输出和错误输出,text=True将输出作为字符串处理。
二、使用os模块
os模块提供了一个简单的方法来执行系统命令,但它不如subprocess模块灵活。以下是一个示例:
import os
def ping(host):
response = os.system(f"ping -c 4 {host}")
if response == 0:
print(f"Ping to {host} successful")
else:
print(f"Ping to {host} failed")
if __name__ == "__main__":
host = "google.com"
ping(host)
虽然os.system简单易用,但它无法捕获命令的输出,并且存在安全性问题(例如命令注入)。
三、使用ping3库
ping3是一个纯Python实现的ping库,它不依赖于系统的ping命令,跨平台支持很好。下面是一个示例:
from ping3 import ping, verbose_ping
def ping_host(host):
delay = ping(host)
if delay is None:
print(f"Ping to {host} failed")
else:
print(f"Ping to {host} successful, delay: {delay} seconds")
if __name__ == "__main__":
host = "google.com"
ping_host(host)
ping3库提供了简单的接口来执行ping命令,并返回延迟时间。如果ping失败,它返回None。
详细描述subprocess模块
subprocess.run方法是执行系统命令的一个高级接口。它简化了执行命令并捕获输出的过程。以下是一些常用参数:
- args: 要执行的命令和参数列表。
- stdout: 指定标准输出的处理方式。
- stderr: 指定错误输出的处理方式。
- text: 如果为True,输出将作为字符串处理。
- check: 如果为True,当命令返回非零状态码时会引发CalledProcessError异常。
例如:
import subprocess
result = subprocess.run(['ping', '-c', '4', 'google.com'], stdout=subprocess.PIPE, stderr=subprocess.PIPE, text=True, check=True)
print(result.stdout)
在这个示例中,check=True会在命令失败时抛出异常,帮助我们更好地处理错误。
处理不同操作系统
不同操作系统的ping命令选项有所不同。例如,Windows使用-n选项来指定发送的请求数,而Linux/Unix使用-c选项。我们可以通过检测操作系统来选择合适的选项:
import subprocess
import platform
def ping(host):
param = '-n' if platform.system().lower() == 'windows' else '-c'
command = ['ping', param, '4', host]
result = subprocess.run(command, stdout=subprocess.PIPE, stderr=subprocess.PIPE, text=True)
if result.returncode == 0:
print(f"Ping to {host} successful:")
print(result.stdout)
else:
print(f"Ping to {host} failed:")
print(result.stderr)
if __name__ == "__main__":
host = "google.com"
ping(host)
通过platform模块,我们可以检测当前操作系统,并相应地调整ping命令的参数。
捕获并解析ping输出
在某些情况下,我们可能需要解析ping命令的输出以提取特定的信息(如平均延迟时间)。我们可以使用正则表达式(re模块)来完成这项任务:
import subprocess
import re
def ping(host):
result = subprocess.run(['ping', '-c', '4', host], stdout=subprocess.PIPE, stderr=subprocess.PIPE, text=True)
if result.returncode == 0:
print(f"Ping to {host} successful:")
print(result.stdout)
# 使用正则表达式提取平均延迟时间
match = re.search(r'avg = ([\d.]+)', result.stdout)
if match:
print(f"Average delay: {match.group(1)} ms")
else:
print(f"Ping to {host} failed:")
print(result.stderr)
if __name__ == "__main__":
host = "google.com"
ping(host)
在这个示例中,我们使用正则表达式来匹配并提取平均延迟时间。这对于需要进一步处理ping结果的应用程序非常有用。
错误处理与异常捕获
在执行系统命令时,处理错误和异常是非常重要的。subprocess模块提供了多种处理错误的方法。例如,我们可以使用try-except块来捕获异常,并根据错误类型采取不同的处理措施:
import subprocess
def ping(host):
try:
result = subprocess.run(['ping', '-c', '4', host], stdout=subprocess.PIPE, stderr=subprocess.PIPE, text=True, check=True)
print(f"Ping to {host} successful:")
print(result.stdout)
except subprocess.CalledProcessError as e:
print(f"Ping to {host} failed with error: {e}")
except Exception as e:
print(f"An unexpected error occurred: {e}")
if __name__ == "__main__":
host = "google.com"
ping(host)
通过捕获不同类型的异常,我们可以对不同的错误情况做出适当的响应,提高程序的健壮性。
使用多线程/多进程
在某些情况下,我们可能需要同时ping多个主机。我们可以使用多线程或多进程来提高效率。以下是一个使用线程池的示例:
import subprocess
from concurrent.futures import ThreadPoolExecutor
def ping(host):
result = subprocess.run(['ping', '-c', '4', host], stdout=subprocess.PIPE, stderr=subprocess.PIPE, text=True)
if result.returncode == 0:
print(f"Ping to {host} successful:")
print(result.stdout)
else:
print(f"Ping to {host} failed:")
print(result.stderr)
if __name__ == "__main__":
hosts = ["google.com", "yahoo.com", "bing.com"]
with ThreadPoolExecutor(max_workers=4) as executor:
executor.map(ping, hosts)
在这个示例中,我们使用concurrent.futures.ThreadPoolExecutor来创建一个线程池,并使用map方法将ping函数应用于多个主机。
结论
在Python脚本中执行ping命令有多种方式,每种方式都有其优缺点。subprocess模块提供了更多的控制和灵活性,是最常用的方法。os模块虽然简单易用,但不如subprocess灵活,并且存在安全性问题。ping3库是一个纯Python实现的ping库,跨平台支持很好,适用于不依赖系统命令的场景。
通过上述方法,我们可以在Python脚本中高效地执行ping命令,并根据不同需求选择合适的实现方式。希望这篇文章对你有所帮助。
相关问答FAQs:
如何在Python脚本中使用ping命令来测试网络连通性?
在Python中,可以使用subprocess
模块来执行系统命令,包括ping命令。通过调用subprocess.run()
或subprocess.Popen()
,你可以发送ping请求并获取结果。例如,使用以下代码可以发送ping请求并打印响应:
import subprocess
def ping(host):
result = subprocess.run(["ping", "-c", "4", host], capture_output=True, text=True)
print(result.stdout)
ping("google.com")
在Python中执行ping命令时有哪些常见的错误?
执行ping命令时,常见的问题包括权限不足、目标主机不可达或网络配置错误。确保你的Python脚本有足够的权限执行系统命令,并检查目标主机的地址是否正确。如果使用Windows系统,ping命令的参数可能需要调整,比如将-c
改为-n
来指定发送的请求数量。
如何在Python中处理ping命令的输出结果?
处理ping命令的输出可以使用字符串方法或正则表达式来提取有用信息。通过分析返回的stdout
,你可以提取丢包率、延迟等数据。以下是一个简单的示例,展示如何提取平均延迟:
import subprocess
import re
def get_ping_average(host):
result = subprocess.run(["ping", "-c", "4", host], capture_output=True, text=True)
average = re.search(r'avg = (\d+\.\d+) ms', result.stdout)
if average:
print(f"Average ping time to {host}: {average.group(1)} ms")
else:
print("Could not find average ping time.")
get_ping_average("google.com")
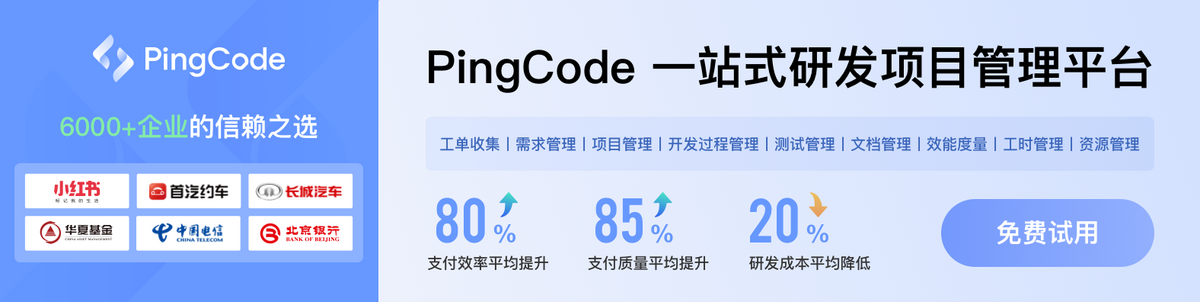