使用Python统计文本中的单词数,可以通过读取文件内容、拆分文本、统计单词频率等方式实现。 其中一种常见的方法是使用Python的内置字符串方法和集合。首先,将文本内容读取到程序中,然后使用字符串的split方法将文本拆分为单词,最后统计每个单词的出现次数。此外,还可以使用Python的collections库中的Counter类来简化统计过程。
一、读取文本内容
在统计单词数之前,首先需要读取文本内容。我们可以使用Python的内置函数open()来读取文件,并将文件内容存储在变量中。以下是一个示例代码:
def read_file(file_path):
with open(file_path, 'r', encoding='utf-8') as file:
text = file.read()
return text
这段代码定义了一个名为read_file的函数,该函数接受一个文件路径作为参数,并返回文件的内容。使用with语句打开文件,可以确保文件在读取完成后自动关闭,以节省资源。
二、拆分文本
读取文本内容后,需要将文本拆分为单词。我们可以使用Python的内置字符串方法split()来完成这一任务。以下是一个示例代码:
def split_text(text):
words = text.split()
return words
这段代码定义了一个名为split_text的函数,该函数接受一个文本字符串作为参数,并返回一个包含单词的列表。split()方法默认会根据空白字符(如空格、制表符和换行符)拆分文本。
三、统计单词频率
拆分文本后,我们可以使用collections库中的Counter类来统计每个单词的出现次数。以下是一个示例代码:
from collections import Counter
def count_words(words):
word_counts = Counter(words)
return word_counts
这段代码定义了一个名为count_words的函数,该函数接受一个包含单词的列表作为参数,并返回一个字典,其中键是单词,值是单词的出现次数。Counter类是一个特殊的字典,用于统计可哈希对象的频率。
四、主函数
最后,我们可以将上述步骤组合在一起,编写一个主函数来统计文本中的单词数。以下是一个完整的示例代码:
from collections import Counter
def read_file(file_path):
with open(file_path, 'r', encoding='utf-8') as file:
text = file.read()
return text
def split_text(text):
words = text.split()
return words
def count_words(words):
word_counts = Counter(words)
return word_counts
def main(file_path):
text = read_file(file_path)
words = split_text(text)
word_counts = count_words(words)
return word_counts
if __name__ == '__main__':
file_path = 'example.txt'
word_counts = main(file_path)
for word, count in word_counts.items():
print(f'{word}: {count}')
在这个示例中,我们首先定义了三个函数read_file、split_text和count_words,然后定义了一个名为main的主函数,该函数将前面定义的函数组合在一起,实现了读取文件、拆分文本和统计单词频率的功能。最后,我们在if name == 'main'中调用主函数,并输出每个单词的出现次数。
五、处理标点符号和大小写
在实际应用中,文本通常包含标点符号和不同大小写的单词。为了提高统计的准确性,我们需要对文本进行预处理,去除标点符号并将所有单词转换为小写。以下是一个示例代码:
import string
from collections import Counter
def read_file(file_path):
with open(file_path, 'r', encoding='utf-8') as file:
text = file.read()
return text
def preprocess_text(text):
text = text.translate(str.maketrans('', '', string.punctuation))
text = text.lower()
return text
def split_text(text):
words = text.split()
return words
def count_words(words):
word_counts = Counter(words)
return word_counts
def main(file_path):
text = read_file(file_path)
text = preprocess_text(text)
words = split_text(text)
word_counts = count_words(words)
return word_counts
if __name__ == '__main__':
file_path = 'example.txt'
word_counts = main(file_path)
for word, count in word_counts.items():
print(f'{word}: {count}')
在这个示例中,我们定义了一个名为preprocess_text的函数,该函数使用string模块去除文本中的标点符号,并将所有单词转换为小写。然后,我们在主函数中调用这个预处理函数,以确保统计结果的准确性。
六、处理停用词
在文本处理中,停用词(如"the"、"is"、"and"等)通常会被过滤掉,因为它们对文本的主要信息贡献较少。我们可以使用NLTK库中的stopwords模块来过滤停用词。以下是一个示例代码:
import string
from collections import Counter
from nltk.corpus import stopwords
def read_file(file_path):
with open(file_path, 'r', encoding='utf-8') as file:
text = file.read()
return text
def preprocess_text(text):
text = text.translate(str.maketrans('', '', string.punctuation))
text = text.lower()
return text
def split_text(text):
words = text.split()
return words
def remove_stopwords(words):
stop_words = set(stopwords.words('english'))
filtered_words = [word for word in words if word not in stop_words]
return filtered_words
def count_words(words):
word_counts = Counter(words)
return word_counts
def main(file_path):
text = read_file(file_path)
text = preprocess_text(text)
words = split_text(text)
words = remove_stopwords(words)
word_counts = count_words(words)
return word_counts
if __name__ == '__main__':
file_path = 'example.txt'
word_counts = main(file_path)
for word, count in word_counts.items():
print(f'{word}: {count}')
在这个示例中,我们定义了一个名为remove_stopwords的函数,该函数使用NLTK库中的stopwords模块过滤停用词。然后,我们在主函数中调用这个函数,以确保统计结果中不包含停用词。
七、处理不同语言的文本
如果需要处理不同语言的文本,我们可以使用NLTK库中的stopwords模块来获取对应语言的停用词列表。以下是一个示例代码:
import string
from collections import Counter
from nltk.corpus import stopwords
def read_file(file_path):
with open(file_path, 'r', encoding='utf-8') as file:
text = file.read()
return text
def preprocess_text(text):
text = text.translate(str.maketrans('', '', string.punctuation))
text = text.lower()
return text
def split_text(text):
words = text.split()
return words
def remove_stopwords(words, language='english'):
stop_words = set(stopwords.words(language))
filtered_words = [word for word in words if word not in stop_words]
return filtered_words
def count_words(words):
word_counts = Counter(words)
return word_counts
def main(file_path, language='english'):
text = read_file(file_path)
text = preprocess_text(text)
words = split_text(text)
words = remove_stopwords(words, language)
word_counts = count_words(words)
return word_counts
if __name__ == '__main__':
file_path = 'example.txt'
language = 'english'
word_counts = main(file_path, language)
for word, count in word_counts.items():
print(f'{word}: {count}')
在这个示例中,我们在remove_stopwords函数和main函数中添加了一个language参数,该参数用于指定要处理的语言。我们可以通过传递不同的语言参数来处理不同语言的文本。
通过上述步骤,我们可以使用Python统计文本中的单词数。这个过程包括读取文件、拆分文本、预处理文本、过滤停用词和统计单词频率。通过适当的修改和扩展,我们可以处理不同语言的文本,并根据具体需求进行进一步分析和处理。
相关问答FAQs:
如何使用Python读取文本文件并统计单词数?
可以使用Python的内置函数来读取文本文件。首先,打开文件并读取内容,然后使用字符串的split()
方法将文本分割成单词,最后使用len()
函数计算单词的数量。示例代码如下:
with open('file.txt', 'r') as file:
text = file.read()
word_count = len(text.split())
print("单词总数:", word_count)
在统计单词数时,如何处理标点符号和大小写?
为确保统计的准确性,可以在分割单词之前使用re
模块来去除标点符号,并将文本转换为小写。这样可以避免同一单词因大小写或标点符号不同而被错误计数。例如:
import re
with open('file.txt', 'r') as file:
text = file.read()
text = re.sub(r'[^\w\s]', '', text).lower()
word_count = len(text.split())
print("单词总数:", word_count)
有没有现成的Python库可以简化单词计数的过程?
是的,collections
模块中的Counter
类可以帮助你快速统计文本中每个单词的出现次数。使用此方法不仅可以得到单词总数,还能查看每个单词的频率。示例代码如下:
from collections import Counter
import re
with open('file.txt', 'r') as file:
text = file.read()
text = re.sub(r'[^\w\s]', '', text).lower()
word_list = text.split()
word_count = Counter(word_list)
print("单词出现频率:", word_count)
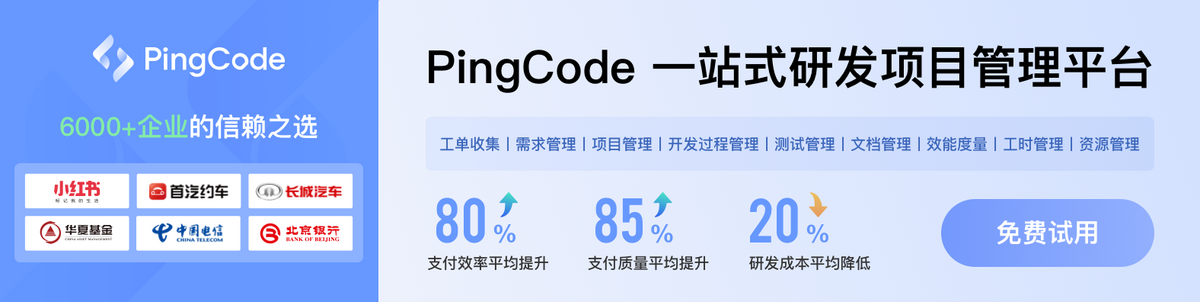