Python删除回车符号的方法有很多种,主要包括使用字符串的replace方法、strip方法和正则表达式等。你可以根据具体情况选择合适的方法。
详细描述:使用字符串的replace方法是最简单直接的方式。通过将字符串中的回车符号(\n或\r)替换为空字符串,可以轻松去掉回车符号。此外,strip方法可以移除字符串开头和结尾的回车符号,而正则表达式则提供了更强大的匹配和替换功能。
一、使用replace方法
使用字符串的replace方法是最常见和简单的方法。可以通过replace方法将回车符号替换为空字符串,从而达到去掉回车符号的目的。
text = "Hello\nWorld\nThis is a test\n"
cleaned_text = text.replace("\n", "")
print(cleaned_text)
在上述示例中,replace方法将所有的换行符(\n)替换为空字符串,从而删除了字符串中的所有换行符。
二、使用strip方法
strip方法用于移除字符串开头和结尾的空白字符,包括换行符、空格和制表符等。可以使用strip方法来去掉字符串开头和结尾的回车符号。
text = "\n\nHello World\n\n"
cleaned_text = text.strip()
print(cleaned_text)
在上述示例中,strip方法移除了字符串开头和结尾的所有换行符。
三、使用正则表达式
正则表达式提供了更强大的匹配和替换功能,可以用于删除字符串中的回车符号。可以使用Python的re模块来实现。
import re
text = "Hello\nWorld\nThis is a test\n"
cleaned_text = re.sub(r"[\r\n]+", "", text)
print(cleaned_text)
在上述示例中,re.sub函数使用正则表达式[\r\n]+匹配一个或多个回车符号,并将其替换为空字符串,从而删除了字符串中的所有回车符号。
四、使用split和join方法
可以使用split方法将字符串按回车符号分割成多个子字符串,然后使用join方法将这些子字符串重新连接起来,从而去掉回车符号。
text = "Hello\nWorld\nThis is a test\n"
parts = text.split("\n")
cleaned_text = "".join(parts)
print(cleaned_text)
在上述示例中,split方法将字符串按换行符分割成多个子字符串,然后join方法将这些子字符串重新连接起来,从而删除了字符串中的所有换行符。
五、处理多行字符串
对于多行字符串,可以使用上述方法中的任意一种来删除回车符号。下面是一个示例,演示了如何处理多行字符串。
text = """Hello
World
This is a test
"""
cleaned_text = text.replace("\n", "")
print(cleaned_text)
在上述示例中,replace方法将多行字符串中的所有换行符替换为空字符串,从而删除了所有换行符。
六、处理文件中的回车符号
有时候需要处理文件中的回车符号,可以读取文件内容并使用上述方法来删除回车符号。下面是一个示例,演示了如何处理文件中的回车符号。
with open("input.txt", "r") as file:
text = file.read()
cleaned_text = text.replace("\n", "")
with open("output.txt", "w") as file:
file.write(cleaned_text)
在上述示例中,读取了文件input.txt的内容,使用replace方法删除了回车符号,然后将处理后的内容写入到文件output.txt中。
七、处理不同平台的回车符号
不同操作系统使用不同的回车符号,例如Windows使用\r\n,Unix和Linux使用\n,Mac使用\r。可以使用replace方法分别处理这些回车符号。
text = "Hello\r\nWorld\rThis is a test\n"
text = text.replace("\r\n", "")
text = text.replace("\r", "")
text = text.replace("\n", "")
print(cleaned_text)
在上述示例中,分别使用replace方法处理了不同平台的回车符号。
八、处理字符串中的回车符号和其他空白字符
有时候不仅需要删除回车符号,还需要删除其他空白字符,例如空格和制表符。可以使用strip方法来删除字符串开头和结尾的所有空白字符。
text = " \tHello World\t \n"
cleaned_text = text.strip()
print(cleaned_text)
在上述示例中,strip方法移除了字符串开头和结尾的所有空白字符。
九、处理HTML中的回车符号
在处理HTML内容时,回车符号可能存在于HTML标签之间,可以使用BeautifulSoup库来解析和处理HTML内容。
from bs4 import BeautifulSoup
html = "<html><body><p>Hello\nWorld</p></body></html>"
soup = BeautifulSoup(html, "html.parser")
cleaned_html = soup.prettify(formatter="minimal")
print(cleaned_html)
在上述示例中,使用BeautifulSoup库解析HTML内容,并通过prettify方法格式化HTML内容,删除了HTML标签之间的回车符号。
十、处理CSV文件中的回车符号
在处理CSV文件时,回车符号可能存在于CSV记录中,可以使用csv模块来处理CSV文件,并删除记录中的回车符号。
import csv
with open("input.csv", "r") as infile, open("output.csv", "w", newline="") as outfile:
reader = csv.reader(infile)
writer = csv.writer(outfile)
for row in reader:
cleaned_row = [field.replace("\n", "").replace("\r", "") for field in row]
writer.writerow(cleaned_row)
在上述示例中,使用csv模块读取CSV文件中的记录,并通过replace方法删除记录中的回车符号,然后将处理后的记录写入到新的CSV文件中。
十一、处理JSON字符串中的回车符号
在处理JSON字符串时,可以使用json模块来解析和处理JSON内容,并删除其中的回车符号。
import json
json_str = '{"message": "Hello\nWorld"}'
data = json.loads(json_str)
cleaned_data = {key: value.replace("\n", "").replace("\r", "") if isinstance(value, str) else value for key, value in data.items()}
cleaned_json_str = json.dumps(cleaned_data)
print(cleaned_json_str)
在上述示例中,使用json模块解析JSON字符串,并通过replace方法删除JSON内容中的回车符号,然后将处理后的数据重新转换为JSON字符串。
十二、处理日志文件中的回车符号
在处理日志文件时,回车符号可能存在于日志记录中,可以通过读取文件内容并使用上述方法删除回车符号。
with open("logfile.log", "r") as file:
log_content = file.read()
cleaned_log_content = log_content.replace("\n", "").replace("\r", "")
with open("cleaned_logfile.log", "w") as file:
file.write(cleaned_log_content)
在上述示例中,读取了日志文件logfile.log的内容,并通过replace方法删除了日志记录中的回车符号,然后将处理后的内容写入到新的日志文件中。
十三、处理XML字符串中的回车符号
在处理XML字符串时,回车符号可能存在于XML标签之间,可以使用ElementTree库来解析和处理XML内容。
import xml.etree.ElementTree as ET
xml_str = "<root><message>Hello\nWorld</message></root>"
root = ET.fromstring(xml_str)
for elem in root.iter():
if elem.text:
elem.text = elem.text.replace("\n", "").replace("\r", "")
cleaned_xml_str = ET.tostring(root, encoding="unicode")
print(cleaned_xml_str)
在上述示例中,使用ElementTree库解析XML字符串,并通过replace方法删除XML标签之间的回车符号,然后将处理后的XML内容重新转换为字符串。
十四、处理列表中的回车符号
在处理列表中的字符串元素时,可以使用列表推导式和replace方法来删除每个元素中的回车符号。
data = ["Hello\nWorld", "This\nis\na\ntest"]
cleaned_data = [item.replace("\n", "").replace("\r", "") for item in data]
print(cleaned_data)
在上述示例中,使用列表推导式遍历列表中的每个字符串元素,并通过replace方法删除其中的回车符号。
十五、处理字典中的回车符号
在处理字典中的字符串值时,可以使用字典推导式和replace方法来删除每个值中的回车符号。
data = {"message": "Hello\nWorld", "info": "This\nis\na\ntest"}
cleaned_data = {key: value.replace("\n", "").replace("\r", "") if isinstance(value, str) else value for key, value in data.items()}
print(cleaned_data)
在上述示例中,使用字典推导式遍历字典中的每个键值对,并通过replace方法删除值中的回车符号。
十六、处理元组中的回车符号
在处理元组中的字符串元素时,可以使用列表推导式和replace方法来删除每个元素中的回车符号,然后将处理后的元素重新转换为元组。
data = ("Hello\nWorld", "This\nis\na\ntest")
cleaned_data = tuple(item.replace("\n", "").replace("\r", "") for item in data)
print(cleaned_data)
在上述示例中,使用列表推导式遍历元组中的每个字符串元素,并通过replace方法删除其中的回车符号,然后将处理后的元素重新转换为元组。
十七、处理集合中的回车符号
在处理集合中的字符串元素时,可以使用集合推导式和replace方法来删除每个元素中的回车符号。
data = {"Hello\nWorld", "This\nis\na\ntest"}
cleaned_data = {item.replace("\n", "").replace("\r", "") for item in data}
print(cleaned_data)
在上述示例中,使用集合推导式遍历集合中的每个字符串元素,并通过replace方法删除其中的回车符号。
十八、处理嵌套数据结构中的回车符号
在处理嵌套数据结构(例如列表、字典和元组的嵌套)中的回车符号时,可以使用递归函数来遍历和处理嵌套的每个元素。
def clean_data(data):
if isinstance(data, str):
return data.replace("\n", "").replace("\r", "")
elif isinstance(data, list):
return [clean_data(item) for item in data]
elif isinstance(data, tuple):
return tuple(clean_data(item) for item in data)
elif isinstance(data, dict):
return {key: clean_data(value) for key, value in data.items()}
elif isinstance(data, set):
return {clean_data(item) for item in data}
else:
return data
data = {"message": "Hello\nWorld", "info": ["This\nis\na\ntest", "Another\nmessage"]}
cleaned_data = clean_data(data)
print(cleaned_data)
在上述示例中,定义了递归函数clean_data来遍历和处理嵌套数据结构中的每个元素,并通过replace方法删除其中的回车符号。
十九、处理Pandas数据框中的回车符号
在处理Pandas数据框中的字符串元素时,可以使用applymap方法和lambda函数来删除每个元素中的回车符号。
import pandas as pd
df = pd.DataFrame({"message": ["Hello\nWorld", "This\nis\na\ntest"]})
cleaned_df = df.applymap(lambda x: x.replace("\n", "").replace("\r", "") if isinstance(x, str) else x)
print(cleaned_df)
在上述示例中,使用applymap方法遍历数据框中的每个元素,并通过lambda函数和replace方法删除字符串元素中的回车符号。
二十、处理NumPy数组中的回车符号
在处理NumPy数组中的字符串元素时,可以使用vectorize函数来创建一个向量化的函数,然后应用于数组中的每个元素。
import numpy as np
arr = np.array(["Hello\nWorld", "This\nis\na\ntest"])
vectorized_replace = np.vectorize(lambda x: x.replace("\n", "").replace("\r", ""))
cleaned_arr = vectorized_replace(arr)
print(cleaned_arr)
在上述示例中,使用vectorize函数创建了一个向量化的replace函数,并将其应用于NumPy数组中的每个元素,从而删除了字符串元素中的回车符号。
通过上述多种方法,可以根据具体情况选择合适的方法来删除Python中的回车符号。无论是处理单个字符串、文件内容、数据结构还是数据框,都可以找到合适的解决方案。
相关问答FAQs:
如何在Python中识别回车符号?
在Python中,可以使用字符串的find()
方法或正则表达式来识别回车符号。回车符号通常表示为\n
(换行符)或\r
(回车符),通过这些方式可以轻松找到并处理它们。
删除回车符号的最佳实践是什么?
使用字符串的replace()
方法是删除回车符号的常见做法。例如,可以使用string.replace('\n', '')
来移除换行符,同时也可以结合strip()
方法去掉字符串开头和结尾的空白字符。
在处理文件时如何去掉回车符号?
在读取文件时,可以在读取内容后立即使用replace()
方法去除回车符号。例如,打开文件后,可以执行content = file.read().replace('\n', '')
来去掉所有的换行符。这种方法特别适合于需要清洗数据的场景。
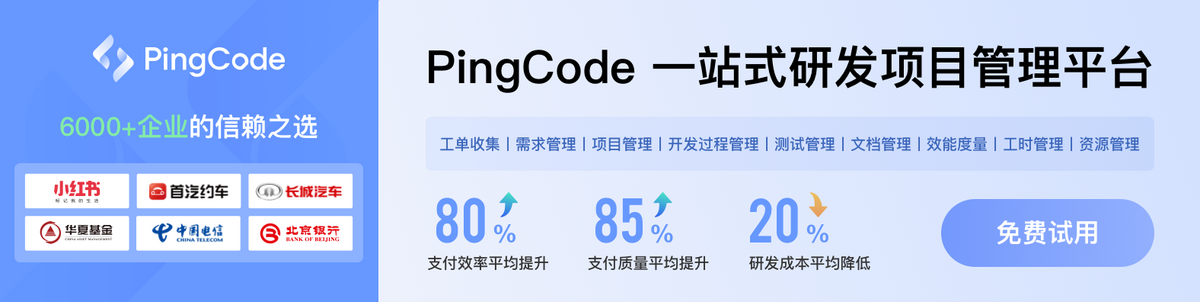