在Python中,使用for循环计算求和可以通过以下步骤实现:定义一个累加变量、使用for循环遍历数列、在循环中累加和。其中,定义一个累加变量是至关重要的步骤,因为它作为存储和更新求和结果的容器。下面将详细解释这一点。
一、定义累加变量
在使用for循环计算求和之前,首先需要定义一个累加变量来存储求和的结果。通常这个变量会被初始化为0。例如:
total = 0
二、使用for循环遍历数列
接下来,需要使用for循环遍历数列中的每一个元素。在每次循环中,我们都会将当前元素的值加到累加变量中。这是实现求和的核心步骤。例如:
for number in range(1, 11): # 遍历从1到10的数列
total += number
三、在循环中累加和
在for循环内部,每次循环都会将当前数列元素的值加到累加变量中。这样循环结束后,累加变量中就存储了所有元素的和。例如:
total = 0
for number in range(1, 11):
total += number
print("The sum is:", total)
以上代码将输出:The sum is: 55
。
一、基本的for循环求和
1.1、求和范围内的整数
最基本的for循环求和是对一个整数范围内的数进行求和。可以使用Python的range函数生成数列,然后遍历这些数进行求和。
total = 0
for number in range(1, 101): # 求和从1到100的整数
total += number
print("The sum of integers from 1 to 100 is:", total)
上述代码中,range(1, 101)生成从1到100的数列,for循环遍历这些数,并将每一个数加到total中。最后输出的结果是5050。
1.2、求和范围内的偶数
如果需要求和范围内的偶数,可以在for循环中加入条件判断,或直接使用range函数生成偶数列。
total = 0
for number in range(2, 101, 2): # 求和从2到100的偶数
total += number
print("The sum of even numbers from 1 to 100 is:", total)
这里,range(2, 101, 2)生成了从2到100的偶数数列,for循环遍历这些偶数并求和。最后输出的结果是2550。
二、列表和元组的求和
2.1、列表求和
除了整数范围,还可以对列表中的数进行求和。
numbers = [1, 2, 3, 4, 5]
total = 0
for number in numbers:
total += number
print("The sum of the list is:", total)
上述代码中,for循环遍历列表中的每一个数,并将其加到total中。最后输出的结果是15。
2.2、元组求和
同样,也可以对元组中的数进行求和。元组与列表类似,只是元组是不可变的。
numbers = (1, 2, 3, 4, 5)
total = 0
for number in numbers:
total += number
print("The sum of the tuple is:", total)
上述代码的运行结果也是15。
三、嵌套for循环求和
在某些情况下,可能需要使用嵌套for循环进行求和。例如,对一个二维数组中的所有元素求和。
matrix = [
[1, 2, 3],
[4, 5, 6],
[7, 8, 9]
]
total = 0
for row in matrix:
for number in row:
total += number
print("The sum of the matrix is:", total)
上述代码中,首先遍历矩阵的每一行,然后在每一行中遍历每一个元素,并将其加到total中。最后输出的结果是45。
四、使用条件的for循环求和
在求和过程中,可以使用条件判断来筛选需要求和的元素。例如,只对列表中的正数进行求和。
numbers = [1, -2, 3, -4, 5]
total = 0
for number in numbers:
if number > 0: # 只对正数求和
total += number
print("The sum of positive numbers is:", total)
上述代码中,for循环遍历列表中的每一个数,并在条件判断中筛选出正数进行求和。最后输出的结果是9。
五、使用enumerate函数的for循环求和
有时候,在遍历列表或其他可迭代对象时,需要同时获取元素的索引和值。这时可以使用enumerate函数。
numbers = [1, 2, 3, 4, 5]
total = 0
for index, number in enumerate(numbers):
total += number
print(f"Index: {index}, Number: {number}, Running Total: {total}")
print("The total sum is:", total)
上述代码中,for循环使用enumerate函数同时获取元素的索引和值,并在循环中进行求和。同时,还输出了每次循环时的索引、当前数和当前累加和。最后输出的结果是15。
六、使用内置函数sum进行求和
虽然for循环是实现求和的常用方法,但Python提供了内置函数sum,可以简化求和过程。
numbers = [1, 2, 3, 4, 5]
total = sum(numbers)
print("The sum of the list is:", total)
上述代码中,sum函数直接对列表中的数进行求和,并将结果赋值给total。最后输出的结果是15。
七、对字典值求和
字典是一种常用的数据结构,有时需要对字典中的值进行求和。
grades = {'math': 90, 'science': 85, 'english': 88}
total = 0
for subject, grade in grades.items():
total += grade
print("The sum of grades is:", total)
上述代码中,for循环遍历字典中的每一个键值对,并将值加到total中。最后输出的结果是263。
八、对文件中的数值求和
在某些应用场景中,可能需要对文件中的数值进行求和。例如,文件中每一行包含一个数值。
total = 0
with open('numbers.txt', 'r') as file:
for line in file:
total += int(line.strip())
print("The sum of numbers in the file is:", total)
上述代码中,使用with open打开文件,并逐行读取文件内容。for循环遍历每一行,并将其转换为整数后加到total中。最后输出的结果是文件中所有数值的和。
九、对生成器求和
生成器是一种特殊的迭代器,有时需要对生成器中的数进行求和。
def number_generator():
for number in range(1, 6):
yield number
total = 0
for number in number_generator():
total += number
print("The sum of the generated numbers is:", total)
上述代码中,定义了一个生成器函数number_generator,使用yield关键字生成数列。for循环遍历生成器中的数,并进行求和。最后输出的结果是15。
十、对复杂数据结构求和
在实际应用中,数据结构可能非常复杂。例如,一个包含嵌套列表和元组的列表。需要对其中的所有数进行求和,可以使用递归函数。
data = [1, [2, 3], (4, 5, [6, 7]), 8, [9, (10, 11)]]
def recursive_sum(data):
total = 0
for item in data:
if isinstance(item, (list, tuple)):
total += recursive_sum(item)
else:
total += item
return total
total = recursive_sum(data)
print("The sum of all numbers in the complex data structure is:", total)
上述代码中,定义了一个递归函数recursive_sum,用于处理嵌套列表和元组。如果当前元素是列表或元组,则递归调用自身进行求和;否则,将当前元素加到total中。最后输出的结果是66。
通过上述各种方法,可以使用Python for循环计算不同类型的数据求和。根据具体应用场景,选择合适的方法,能够提高代码的可读性和运行效率。希望这些示例能帮助你更好地理解和应用Python的for循环求和。
相关问答FAQs:
如何使用Python中的for循环来计算一系列数字的和?
可以通过创建一个for循环来遍历一个数字序列并累加它们的值。首先,定义一个变量用于存储总和,然后用for循环遍历数字序列,将每个数字加到总和中。以下是一个简单的示例:
total_sum = 0
for num in range(1, 101): # 计算1到100的和
total_sum += num
print(total_sum) # 输出结果5050
在Python中,如何使用for循环处理用户输入的数值以计算总和?
您可以结合input()
函数来获取用户输入的数值,并将其转换为整数或浮点数。使用for循环来遍历这些数值并计算和。以下是实现的示例:
numbers = input("请输入一系列数字,用空格分隔:").split()
total_sum = 0
for num in numbers:
total_sum += int(num) # 或使用float(num)处理浮点数
print("总和为:", total_sum)
在Python中,for循环的性能如何影响大规模数据求和的效率?
对于大规模数据集,for循环可能会导致性能瓶颈。优化策略包括使用内置函数如sum()
来提高效率。Python的sum()
函数比手动循环更高效,因为它是用C语言实现的,能够更快地处理大数据量。示例代码如下:
numbers = range(1, 1000001) # 计算1到1000000的和
total_sum = sum(numbers)
print(total_sum)
使用这种方法可以显著提高处理速度,尤其是在处理大量数据时。
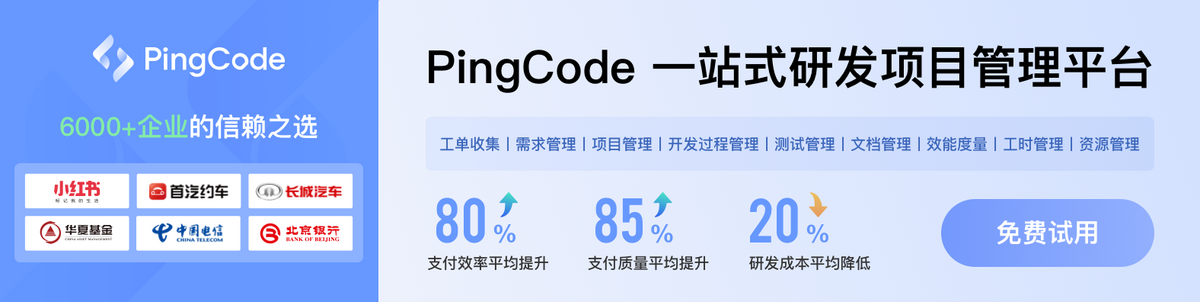