在Python中,要在多线程环境中获取线程的返回值,可以使用多个方法,例如使用Thread
对象的子类化、使用concurrent.futures
模块中的ThreadPoolExecutor
、使用queue.Queue
等方法。在本文中,我们将详细探讨这些方法,并提供示例代码来说明它们的实现方式。
一、使用Thread对象的子类化
在Python中,可以通过子类化threading.Thread
对象并重写其run
方法来获取返回值。通过这种方式,可以在子类中定义一个实例变量来存储线程的返回值,并在主线程中访问该实例变量。
import threading
class MyThread(threading.Thread):
def __init__(self, *args, kwargs):
super(MyThread, self).__init__(*args, kwargs)
self._return = None
def run(self):
if self._target is not None:
self._return = self._target(*self._args, self._kwargs)
def join(self, *args, kwargs):
super(MyThread, self).join(*args, kwargs)
return self._return
def example_function(data):
return f"Processed {data}"
创建线程对象
thread = MyThread(target=example_function, args=("data",))
thread.start()
result = thread.join()
print(result) # 输出: Processed data
通过子类化threading.Thread
,我们可以在子类中定义一个实例变量来存储线程的返回值,并在主线程中通过调用join
方法来获取返回值。
二、使用concurrent.futures模块中的ThreadPoolExecutor
concurrent.futures
模块提供了一个更高级别的接口来管理线程和进程。使用ThreadPoolExecutor
,可以轻松地在多线程环境中获取返回值。
from concurrent.futures import ThreadPoolExecutor
def example_function(data):
return f"Processed {data}"
创建线程池
with ThreadPoolExecutor(max_workers=2) as executor:
# 提交任务
future = executor.submit(example_function, "data")
# 获取返回值
result = future.result()
print(result) # 输出: Processed data
使用ThreadPoolExecutor
,我们可以通过submit
方法提交任务,并通过Future
对象的result
方法获取返回值。这种方法非常简洁且易于使用。
三、使用queue.Queue
另一种获取线程返回值的方法是使用queue.Queue
。我们可以创建一个队列,将线程的返回值放入队列中,并在主线程中从队列中获取返回值。
import threading
import queue
def example_function(data, q):
result = f"Processed {data}"
q.put(result)
q = queue.Queue()
thread = threading.Thread(target=example_function, args=("data", q))
thread.start()
thread.join()
result = q.get()
print(result) # 输出: Processed data
通过使用queue.Queue
,我们可以在线程中将返回值放入队列中,并在主线程中通过get
方法从队列中获取返回值。这种方法也非常直观且易于实现。
四、使用回调函数
在某些情况下,我们可以使用回调函数来获取线程的返回值。我们可以将回调函数作为参数传递给线程函数,并在线程函数执行完毕后调用回调函数。
import threading
def example_function(data, callback):
result = f"Processed {data}"
callback(result)
def callback_function(result):
print(result) # 输出: Processed data
thread = threading.Thread(target=example_function, args=("data", callback_function))
thread.start()
thread.join()
通过使用回调函数,我们可以在线程执行完毕后立即处理返回值。这种方法特别适用于需要在返回值生成后立即进行处理的情况。
五、使用全局变量或共享变量
在多线程编程中,也可以使用全局变量或共享变量来存储和获取线程的返回值。然而,需要注意的是,在这种情况下,可能会出现线程安全问题,因此通常需要使用锁机制来确保线程安全。
import threading
result = None
lock = threading.Lock()
def example_function(data):
global result
res = f"Processed {data}"
with lock:
result = res
thread = threading.Thread(target=example_function, args=("data",))
thread.start()
thread.join()
print(result) # 输出: Processed data
通过使用全局变量和锁机制,可以确保在多线程环境中安全地存储和访问线程的返回值。然而,由于全局变量的使用可能会导致代码的可维护性降低,因此建议尽量避免使用这种方法。
六、总结
在Python中,有多种方法可以在多线程环境中获取线程的返回值。每种方法都有其优缺点,适用于不同的场景。通过子类化threading.Thread
对象、使用concurrent.futures
模块中的ThreadPoolExecutor
、使用queue.Queue
、使用回调函数以及使用全局变量或共享变量等方法,我们可以灵活地在多线程编程中处理返回值。根据具体的需求选择合适的方法,可以有效地提高代码的可读性和可维护性。
相关问答FAQs:
如何在Python多线程中获取返回值?
在Python多线程中,线程通常不直接返回值,因为它们是异步执行的。但是,可以通过使用concurrent.futures.ThreadPoolExecutor
或将结果存储在共享的数据结构中来实现获取返回值的目的。使用ThreadPoolExecutor
时,可以提交任务并使用Future
对象来获取结果,这样就能轻松地获取每个线程的返回值。
使用队列(Queue)来获取线程返回值是否可行?
是的,使用queue.Queue
是获取线程返回值的一个有效方法。每个线程可以将结果放入队列中,主线程则可以从队列中获取这些结果。这种方法可以确保线程安全,并且能够处理多个线程返回的数据。
多线程返回值的处理是否会影响程序性能?
多线程的返回值处理可能会对性能有一定影响,特别是在需要频繁访问共享数据的情况下。使用Queue
时,可能会引入一些额外的开销。然而,合理设计线程的工作负载和数据处理方式可以最大限度地减少这种影响,确保程序在并发执行时仍然高效。
在多线程中,如果任务执行失败,如何处理返回值?
在多线程中,如果任务执行失败,可以通过捕获异常并将其放入共享数据结构(如队列)中来处理。使用concurrent.futures
模块时,Future
对象会保存异常信息,可以通过result()
方法获取到异常,从而方便地进行错误处理。这种方式能够确保即使部分线程失败,程序仍然可以继续运行。
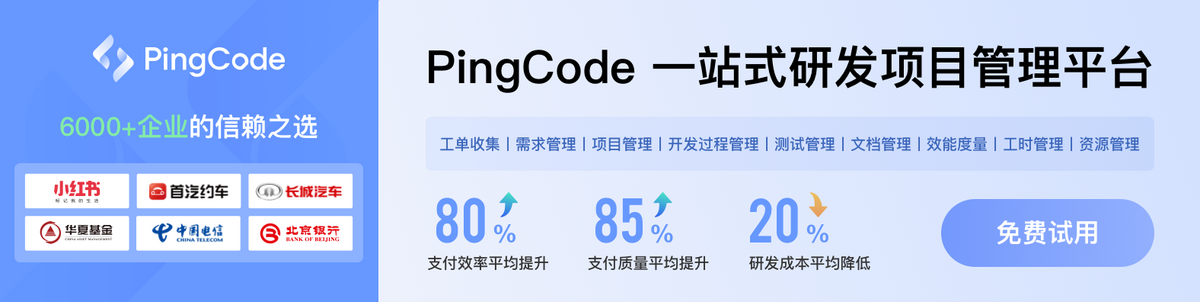