Python库json如何读数据库:Python中的json库无法直接读取数据库,需要结合数据库连接库(如sqlite3、pymysql、psycopg2)进行操作、首先连接数据库、然后将数据转换为json格式存储。使用数据库连接库进行连接、读取数据并转换为json格式、使用json库进行数据序列化。接下来我们详细介绍如何通过实例实现这些步骤。
使用数据库连接库进行连接:
在使用json库之前,我们需要选择合适的数据库连接库,并通过它来连接到数据库。Python提供了多种数据库连接库,如sqlite3、pymysql、psycopg2等,下面我们将分别介绍它们的使用方法。
一、使用sqlite3连接SQLite数据库
SQLite是一个轻量级的数据库,适合存储少量数据。Python的标准库中包含了sqlite3模块,无需额外安装。
import sqlite3
连接到SQLite数据库
conn = sqlite3.connect('example.db')
创建一个游标对象
cursor = conn.cursor()
执行SQL查询
cursor.execute("SELECT * FROM users")
获取所有结果
rows = cursor.fetchall()
关闭游标和连接
cursor.close()
conn.close()
读取数据并转换为json格式:
一旦我们从数据库中获取了数据,下一步就是将其转换为json格式。为了实现这一点,我们可以使用json库中的dumps
函数。
import json
定义一个函数,将数据库结果转换为json
def convert_to_json(data):
columns = ["id", "name", "email"] # 假设表中的列名
result = []
for row in data:
result.append(dict(zip(columns, row)))
return json.dumps(result, indent=4)
示例数据
data = [(1, "John Doe", "john@example.com"), (2, "Jane Doe", "jane@example.com")]
转换为json
json_data = convert_to_json(data)
print(json_data)
二、使用pymysql连接MySQL数据库
MySQL是一种流行的关系型数据库管理系统。我们可以使用pymysql库来连接MySQL数据库。需要先安装pymysql库:
pip install pymysql
import pymysql
连接到MySQL数据库
conn = pymysql.connect(
host='localhost',
user='yourusername',
password='yourpassword',
database='yourdatabase'
)
创建一个游标对象
cursor = conn.cursor()
执行SQL查询
cursor.execute("SELECT * FROM users")
获取所有结果
rows = cursor.fetchall()
关闭游标和连接
cursor.close()
conn.close()
转换为json
json_data = convert_to_json(rows)
print(json_data)
三、使用psycopg2连接PostgreSQL数据库
PostgreSQL是一种功能强大的开源关系型数据库管理系统。我们可以使用psycopg2库来连接PostgreSQL数据库。需要先安装psycopg2库:
pip install psycopg2
import psycopg2
连接到PostgreSQL数据库
conn = psycopg2.connect(
host='localhost',
user='yourusername',
password='yourpassword',
dbname='yourdatabase'
)
创建一个游标对象
cursor = conn.cursor()
执行SQL查询
cursor.execute("SELECT * FROM users")
获取所有结果
rows = cursor.fetchall()
关闭游标和连接
cursor.close()
conn.close()
转换为json
json_data = convert_to_json(rows)
print(json_data)
四、处理复杂数据结构
在实际应用中,数据库中的数据结构可能会比较复杂,包括嵌套关系、多表关联等。我们需要根据具体情况,对数据进行预处理,然后再转换为json格式。
多表关联查询:
在关系型数据库中,多表关联查询是常见的操作。下面示例展示了如何进行多表关联查询,并将结果转换为json格式。
import sqlite3
import json
连接到SQLite数据库
conn = sqlite3.connect('example.db')
cursor = conn.cursor()
执行多表关联查询
cursor.execute("""
SELECT users.id, users.name, orders.order_id, orders.amount
FROM users
JOIN orders ON users.id = orders.user_id
""")
获取所有结果
rows = cursor.fetchall()
关闭游标和连接
cursor.close()
conn.close()
转换为json
columns = ["id", "name", "order_id", "amount"]
result = []
for row in rows:
result.append(dict(zip(columns, row)))
json_data = json.dumps(result, indent=4)
print(json_data)
处理嵌套关系:
有时我们需要将嵌套关系的数据转换为json格式。下面的示例展示了如何处理嵌套关系的数据。
import sqlite3
import json
连接到SQLite数据库
conn = sqlite3.connect('example.db')
cursor = conn.cursor()
执行查询获取用户信息
cursor.execute("SELECT id, name FROM users")
users = cursor.fetchall()
获取每个用户的订单信息
user_data = []
for user in users:
user_id, user_name = user
cursor.execute("SELECT order_id, amount FROM orders WHERE user_id = ?", (user_id,))
orders = cursor.fetchall()
user_data.append({
"id": user_id,
"name": user_name,
"orders": [{"order_id": order[0], "amount": order[1]} for order in orders]
})
关闭游标和连接
cursor.close()
conn.close()
转换为json
json_data = json.dumps(user_data, indent=4)
print(json_data)
五、数据验证与错误处理
在实际应用中,数据验证与错误处理非常重要。我们需要确保数据的完整性和正确性,同时处理可能出现的错误。
数据验证:
在将数据转换为json之前,我们可以对数据进行验证,确保其符合预期格式。
import re
def validate_data(data):
for user in data:
if not isinstance(user["id"], int) or user["id"] <= 0:
raise ValueError("Invalid user ID")
if not isinstance(user["name"], str) or not user["name"]:
raise ValueError("Invalid user name")
for order in user["orders"]:
if not isinstance(order["order_id"], int) or order["order_id"] <= 0:
raise ValueError("Invalid order ID")
if not isinstance(order["amount"], float) or order["amount"] <= 0:
raise ValueError("Invalid order amount")
示例数据
user_data = [
{"id": 1, "name": "John Doe", "orders": [{"order_id": 101, "amount": 99.99}]},
{"id": 2, "name": "Jane Doe", "orders": [{"order_id": 102, "amount": 49.99}]}
]
验证数据
validate_data(user_data)
转换为json
json_data = json.dumps(user_data, indent=4)
print(json_data)
错误处理:
在数据库操作和数据转换过程中,可能会出现各种错误。我们需要通过错误处理机制,确保程序的稳定性。
import sqlite3
import json
try:
# 连接到SQLite数据库
conn = sqlite3.connect('example.db')
cursor = conn.cursor()
# 执行查询
cursor.execute("SELECT id, name FROM users")
users = cursor.fetchall()
# 获取每个用户的订单信息
user_data = []
for user in users:
user_id, user_name = user
cursor.execute("SELECT order_id, amount FROM orders WHERE user_id = ?", (user_id,))
orders = cursor.fetchall()
user_data.append({
"id": user_id,
"name": user_name,
"orders": [{"order_id": order[0], "amount": order[1]} for order in orders]
})
# 关闭游标和连接
cursor.close()
conn.close()
# 转换为json
json_data = json.dumps(user_data, indent=4)
print(json_data)
except sqlite3.Error as e:
print(f"SQLite error: {e}")
except ValueError as e:
print(f"Data validation error: {e}")
except Exception as e:
print(f"Unexpected error: {e}")
通过以上步骤,我们能够使用Python的json库结合数据库连接库,成功地从数据库中读取数据,并将其转换为json格式。确保在实际应用中处理复杂数据结构、进行数据验证与错误处理,以提高程序的可靠性和健壮性。
相关问答FAQs:
如何使用Python库json读取数据库中的数据?
在使用Python库json读取数据库数据之前,需要先从数据库中提取数据。可以使用如sqlite3、pymysql等库连接数据库,并执行SQL查询。获取的数据通常是以字典或列表的形式返回,接着可以使用json库将其转换为JSON格式。例如,使用json.dumps()
方法将Python对象转换为JSON字符串。
在读取数据库数据时,如何处理空值或缺失值?
在数据库中,空值或缺失值是常见的情况。可以在提取数据后,使用Python的条件语句检查这些值,并根据需求进行处理。例如,可以将其转换为None
,或用特定的占位符替代。在转换为JSON格式之前,确保数据的完整性和一致性,以避免在后续处理中出现错误。
JSON格式的数据在数据库中存储有什么优势?
使用JSON格式存储数据在数据库中可以提高灵活性和可扩展性。由于JSON支持嵌套结构,可以方便地存储复杂的数据类型。同时,许多现代数据库(如PostgreSQL和MongoDB)原生支持JSON格式,这使得在查询和更新时更加高效。此外,JSON格式易于与Web应用程序交互,特别是在处理API请求和响应时非常方便。
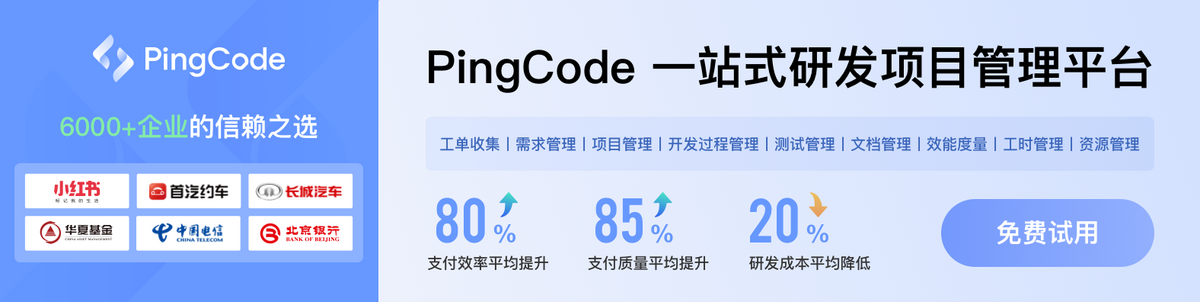