用Python做关键词词频可以通过多种方法实现,例如使用字符串操作、正则表达式、或者借助第三方库如NLTK、spaCy等。具体实现步骤包括:读取文本、预处理(如去除停用词、标点符号)、计算词频、可视化数据。 其中,预处理是关键的一步,通过去除停用词和标点符号可以显著提高词频统计的准确性。
以下将详细描述如何用Python来计算关键词词频,并提供代码示例和具体步骤。
一、读取文本
读取文本是词频统计的第一步,Python可以通过多种方式来读取文本文件,例如使用内置的open
函数或pandas库。
# 使用open函数读取文本文件
with open('textfile.txt', 'r', encoding='utf-8') as file:
text = file.read()
二、文本预处理
预处理步骤包括将文本转换为小写、去除标点符号、去除停用词等。这里我们将使用Python的字符串操作和正则表达式库re
来完成这一步。
import re
将文本转换为小写
text = text.lower()
去除标点符号
text = re.sub(r'[^\w\s]', '', text)
为了去除停用词,我们可以使用NLTK库,它提供了一组常见的英语停用词列表。
import nltk
from nltk.corpus import stopwords
nltk.download('stopwords')
stop_words = set(stopwords.words('english'))
分词并去除停用词
words = text.split()
filtered_words = [word for word in words if word not in stop_words]
三、计算词频
计算词频可以使用Python的collections.Counter
类来实现,这是一个高效的计数器。
from collections import Counter
计算词频
word_counts = Counter(filtered_words)
四、可视化数据
我们可以使用matplotlib库来可视化词频数据,使得结果更加直观。
import matplotlib.pyplot as plt
获取前10个最常见的词及其频率
common_words = word_counts.most_common(10)
words, counts = zip(*common_words)
绘制柱状图
plt.bar(words, counts)
plt.xlabel('Words')
plt.ylabel('Frequency')
plt.title('Top 10 Words Frequency')
plt.show()
五、完整代码示例
将上述步骤整合在一起,形成一个完整的Python脚本。
import re
import nltk
from collections import Counter
import matplotlib.pyplot as plt
from nltk.corpus import stopwords
def read_text_file(file_path):
with open(file_path, 'r', encoding='utf-8') as file:
return file.read()
def preprocess_text(text):
text = text.lower()
text = re.sub(r'[^\w\s]', '', text)
return text
def remove_stopwords(words):
nltk.download('stopwords')
stop_words = set(stopwords.words('english'))
return [word for word in words if word not in stop_words]
def calculate_word_frequency(words):
return Counter(words)
def visualize_word_frequency(word_counts, top_n=10):
common_words = word_counts.most_common(top_n)
words, counts = zip(*common_words)
plt.bar(words, counts)
plt.xlabel('Words')
plt.ylabel('Frequency')
plt.title('Top {} Words Frequency'.format(top_n))
plt.show()
主函数
def main(file_path):
text = read_text_file(file_path)
preprocessed_text = preprocess_text(text)
words = preprocessed_text.split()
filtered_words = remove_stopwords(words)
word_counts = calculate_word_frequency(filtered_words)
visualize_word_frequency(word_counts)
调用主函数,传入文本文件路径
main('textfile.txt')
六、扩展与优化
1. 使用更强大的NLP库
除了NLTK,spaCy也是一个强大的NLP库,可以提供更高效的文本处理和更丰富的功能。
import spacy
nlp = spacy.load('en_core_web_sm')
def preprocess_text_spacy(text):
doc = nlp(text)
tokens = [token.lemma_ for token in doc if not token.is_stop and not token.is_punct]
return tokens
替换原来的文本预处理函数
preprocessed_text = preprocess_text_spacy(text)
2. 处理大规模文本
对于大规模文本,可以考虑使用流式处理来逐行读取文件,减少内存占用。
def read_large_text_file(file_path):
with open(file_path, 'r', encoding='utf-8') as file:
for line in file:
yield line
修改主函数,逐行读取文本并处理
def main_large_file(file_path):
word_counts = Counter()
for line in read_large_text_file(file_path):
preprocessed_line = preprocess_text(line)
words = preprocessed_line.split()
filtered_words = remove_stopwords(words)
word_counts.update(filtered_words)
visualize_word_frequency(word_counts)
调用主函数
main_large_file('large_textfile.txt')
通过以上步骤,你可以使用Python高效地进行关键词词频统计,并根据需求进行扩展和优化。无论是处理小规模文本还是大规模文本,Python都能提供灵活高效的解决方案。
相关问答FAQs:
如何使用Python提取文本中的关键词?
在Python中,可以使用一些自然语言处理库,如NLTK或spaCy,来提取文本中的关键词。首先,需要对文本进行分词、去除停用词和标点符号,然后可以使用TF-IDF(词频-逆文档频率)或其他算法来识别关键词。具体步骤包括加载文本、清洗数据、计算词频以及最终提取关键词。
使用Python进行关键词分析需要哪些库?
进行关键词分析通常需要安装一些Python库,如NLTK、spaCy、Pandas和Scikit-learn。NLTK和spaCy用于文本预处理和自然语言处理,而Pandas可用于数据处理,Scikit-learn则提供了机器学习工具来进行更复杂的分析。通过这些库,可以有效地处理和分析文本数据。
如何判断一个关键词的重要性?
判断关键词的重要性通常可以通过计算其词频和逆文档频率(TF-IDF)来实现。词频表示关键词在文本中出现的频率,而逆文档频率则衡量关键词在整个语料库中的稀有程度。结合这两个指标,可以找到在特定文本中相对重要的关键词。此外,还可以使用数据可视化工具,帮助识别和理解关键词的重要性。
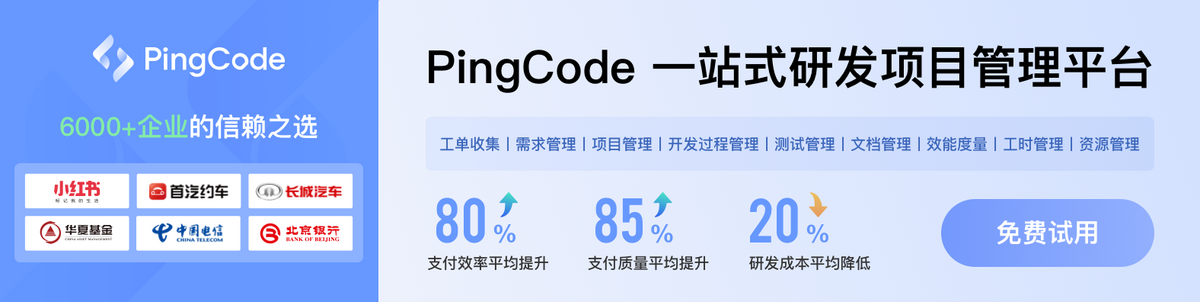