在Python中分割文本文件的方法有很多种,包括按行、按字符、按特定分隔符、使用正则表达式等。 其中,按行分割是最常见的方法,因为大多数情况下,我们需要逐行处理文本文件。为了深入了解这些方法,下面将详细介绍几种常见的文本文件分割方法,并提供示例代码。
一、按行分割文本文件
按行分割是最常见的文本文件分割方法,可以使用Python的内置函数 readlines()
或者迭代文件对象。
使用 readlines()
readlines()
函数将文件中的所有行读取到一个列表中,每一行作为列表中的一个元素。
def split_file_by_lines(filepath):
with open(filepath, 'r', encoding='utf-8') as file:
lines = file.readlines()
return lines
示例调用
filepath = 'example.txt'
lines = split_file_by_lines(filepath)
for line in lines:
print(line.strip())
使用文件对象的迭代
直接迭代文件对象也可以逐行读取文件,这种方法更为高效,适合处理大型文件。
def split_file_by_lines_iter(filepath):
with open(filepath, 'r', encoding='utf-8') as file:
for line in file:
print(line.strip())
示例调用
filepath = 'example.txt'
split_file_by_lines_iter(filepath)
二、按字符数分割文本文件
有时我们需要根据字符数来分割文件,这可以通过读取固定大小的块来实现。
def split_file_by_chars(filepath, chunk_size):
with open(filepath, 'r', encoding='utf-8') as file:
while True:
chunk = file.read(chunk_size)
if not chunk:
break
print(chunk)
示例调用
filepath = 'example.txt'
chunk_size = 100 # 每100字符一个块
split_file_by_chars(filepath, chunk_size)
三、按特定分隔符分割文本文件
如果文本文件使用特定的字符作为分隔符,例如逗号、空格等,可以使用 split()
函数分割文件内容。
def split_file_by_delimiter(filepath, delimiter):
with open(filepath, 'r', encoding='utf-8') as file:
content = file.read()
parts = content.split(delimiter)
return parts
示例调用
filepath = 'example.txt'
delimiter = ',' # 以逗号作为分隔符
parts = split_file_by_delimiter(filepath, delimiter)
for part in parts:
print(part.strip())
四、使用正则表达式分割文本文件
正则表达式提供了更强大的分割功能,适合复杂的分隔模式。
import re
def split_file_by_regex(filepath, pattern):
with open(filepath, 'r', encoding='utf-8') as file:
content = file.read()
parts = re.split(pattern, content)
return parts
示例调用
filepath = 'example.txt'
pattern = r'\s+' # 以一个或多个空白字符作为分隔符
parts = split_file_by_regex(filepath, pattern)
for part in parts:
print(part.strip())
五、按固定大小分割文本文件并保存到多个文件
有时候我们需要将一个大文件按固定大小分割成多个小文件,这可以通过逐步读取并写入多个文件来实现。
def split_file_to_multiple_files(filepath, chunk_size, output_dir):
with open(filepath, 'r', encoding='utf-8') as file:
i = 0
while True:
chunk = file.read(chunk_size)
if not chunk:
break
output_filepath = f"{output_dir}/part_{i}.txt"
with open(output_filepath, 'w', encoding='utf-8') as output_file:
output_file.write(chunk)
i += 1
示例调用
filepath = 'example.txt'
chunk_size = 1024 # 每个文件1KB
output_dir = 'output'
split_file_to_multiple_files(filepath, chunk_size, output_dir)
六、按逻辑分割文本文件
有些时候,我们需要根据逻辑条件分割文件,例如按段落分割,按某些关键字分割等。这需要结合具体需求和条件来进行分割。
def split_file_by_paragraphs(filepath):
with open(filepath, 'r', encoding='utf-8') as file:
content = file.read()
paragraphs = content.split('\n\n') # 按双换行符分割段落
return paragraphs
示例调用
filepath = 'example.txt'
paragraphs = split_file_by_paragraphs(filepath)
for paragraph in paragraphs:
print(paragraph.strip())
总结
Python提供了多种方法来分割文本文件,无论是按行、按字符、按特定分隔符、使用正则表达式,还是按逻辑条件分割,都可以通过合适的函数和模块来实现。选择合适的方法取决于具体的需求和文件的格式。 通过熟练掌握这些方法,可以高效地处理各种文本文件分割任务。
相关问答FAQs:
如何在Python中读取大文本文件?
在处理大型文本文件时,可以使用Python的内置函数open()
来逐行读取文件,这样可以有效管理内存使用。结合使用for
循环,可以逐行处理文件内容,避免一次性加载整个文件。例如:
with open('large_file.txt', 'r') as file:
for line in file:
# 处理每一行
print(line.strip())
Python支持哪些文本分割方法?
Python提供了多种文本分割方法,包括str.split()
、str.splitlines()
和re.split()
等。使用str.split()
可以根据指定的分隔符分割字符串,而str.splitlines()
可以按行分割文本。使用正则表达式的re.split()
方法则可以根据复杂的模式进行分割,这对处理格式不规则的文本非常有用。
如何将分割后的文本保存到新文件中?
在分割文本后,可以将结果保存到新的文件中。可以使用open()
函数以写入模式打开新文件,并使用write()
方法将分割后的内容写入。例如:
with open('output_file.txt', 'w') as output_file:
for part in text_parts:
output_file.write(part + '\n')
以上代码示例展示了如何将分割后的每一部分写入新的文本文件中。
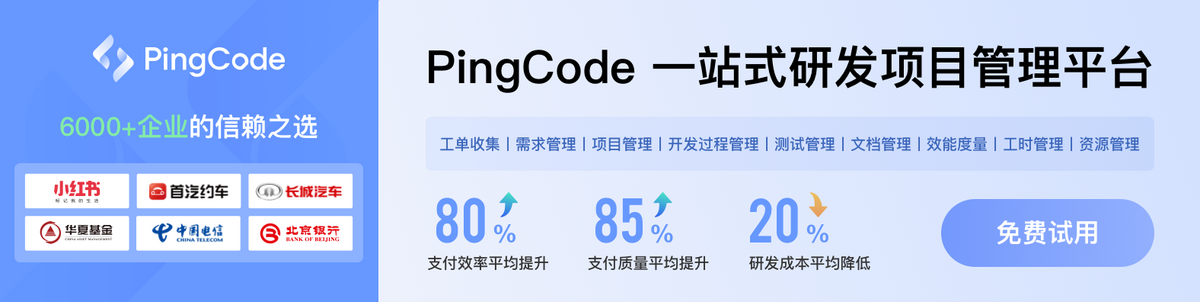