使用Python从文件中删除指定列表的内容
在Python中,删除文件中的指定列表内容是一项常见的任务。我们可以通过读取文件内容、删除指定内容、再将修改后的内容写回文件。其中的关键步骤包括:读取文件、处理内容、写回文件。下面将详细描述如何实现这一过程。
一、读取文件内容
首先,我们需要读取文件内容。我们可以使用Python的内置open
函数来完成这一任务。
def read_file(file_path):
with open(file_path, 'r') as file:
lines = file.readlines()
return lines
二、删除指定列表内容
接下来,我们需要删除文件中的指定列表内容。我们可以通过遍历文件内容,检查每一行是否包含指定列表中的元素,并删除这些元素。
def remove_specified_content(lines, content_to_remove):
updated_lines = []
for line in lines:
if not any(item in line for item in content_to_remove):
updated_lines.append(line)
return updated_lines
三、写回文件
最后,我们需要将处理后的内容写回文件。我们可以使用open
函数以写模式打开文件,并将修改后的内容写入文件。
def write_file(file_path, lines):
with open(file_path, 'w') as file:
file.writelines(lines)
四、综合示例
下面是一个完整的示例代码,演示如何从文件中删除指定列表内容。
def read_file(file_path):
with open(file_path, 'r') as file:
lines = file.readlines()
return lines
def remove_specified_content(lines, content_to_remove):
updated_lines = []
for line in lines:
if not any(item in line for item in content_to_remove):
updated_lines.append(line)
return updated_lines
def write_file(file_path, lines):
with open(file_path, 'w') as file:
file.writelines(lines)
def remove_content_from_file(file_path, content_to_remove):
lines = read_file(file_path)
updated_lines = remove_specified_content(lines, content_to_remove)
write_file(file_path, updated_lines)
示例用法
file_path = 'example.txt'
content_to_remove = ['delete this line', 'remove this content']
remove_content_from_file(file_path, content_to_remove)
五、优化处理大文件
对于大文件,我们需要优化读取和写入的效率,以避免内存占用过高。我们可以逐行处理文件内容,而不是一次性读取所有内容。
def remove_content_from_large_file(file_path, content_to_remove):
temp_file_path = file_path + '.tmp'
with open(file_path, 'r') as read_file, open(temp_file_path, 'w') as write_file:
for line in read_file:
if not any(item in line for item in content_to_remove):
write_file.write(line)
os.replace(temp_file_path, file_path)
在上面的代码中,我们使用了一个临时文件来存储修改后的内容,最后将临时文件替换原文件。
六、处理特殊情况
在实际应用中,我们还需要处理一些特殊情况。例如,文件不存在或文件权限不足的错误。
import os
def safe_remove_content_from_file(file_path, content_to_remove):
if not os.path.exists(file_path):
print(f"Error: File {file_path} does not exist.")
return
try:
remove_content_from_large_file(file_path, content_to_remove)
print(f"Content removed successfully from {file_path}.")
except Exception as e:
print(f"Error: {e}")
示例用法
file_path = 'example.txt'
content_to_remove = ['delete this line', 'remove this content']
safe_remove_content_from_file(file_path, content_to_remove)
七、总结
从文件中删除指定列表内容是一个常见的任务,可以通过读取文件、处理内容、再写回文件来实现。对于大文件,我们需要优化处理效率,并处理可能的错误情况。通过以上步骤和示例代码,我们可以轻松实现这一任务。
希望这篇文章能帮助你更好地理解如何使用Python从文件中删除指定列表内容。如果你有任何问题或建议,欢迎在评论区留言交流。
相关问答FAQs:
如何在Python中读取文件并删除指定内容?
在Python中,可以使用内置的文件操作功能来读取文件内容,并通过列表过滤掉需要删除的特定内容。可以使用open()
函数读取文件,使用列表推导式或循环遍历内容并根据条件进行筛选。最后,将筛选后的内容写回文件中。
可以使用哪些方法来删除文件中的特定行?
除了使用列表推导式,还有其他方法可以删除文件中的特定行。例如,可以将文件的所有行读取到一个列表中,使用remove()
方法删除指定的行,或者通过filter()
函数进行过滤。处理完之后,需将更新后的内容写回原文件或新文件。
如何确保删除操作不会意外丢失数据?
在进行文件删除操作之前,备份原文件是一个好的做法。可以在删除之前将文件复制到另一个位置,确保在出现意外时能够恢复。此外,使用异常处理(如try...except
)可以捕获潜在错误,防止数据丢失。
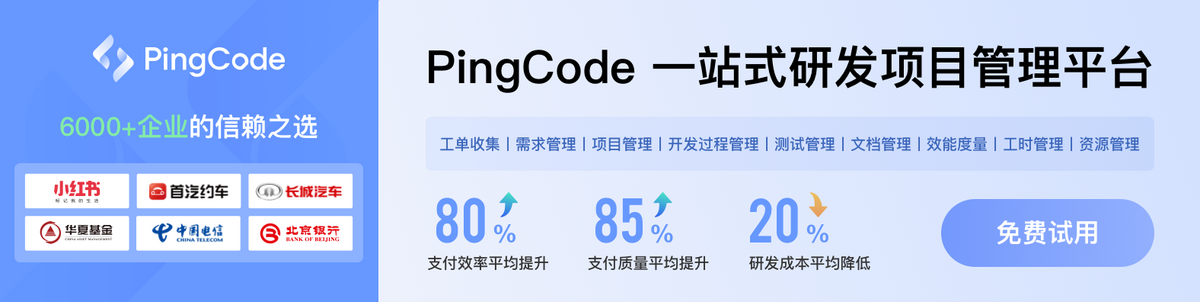