如何用python统计四级词汇
要使用Python统计四级词汇,可以通过以下方法:使用文本预处理、分词技术、正则表达式、计数器等工具。首先,准备一个包含四级词汇的词库,然后读取需要统计的文本,对文本进行预处理和分词,最后统计词汇的频率。读取词库、预处理文本、分词、统计频率是实现这一目标的关键步骤。下面将重点介绍如何进行文本预处理。
一、读取词库
首先,我们需要一个四级词汇的词库,这可以是一个包含所有四级词汇的文本文件或数据库。我们可以读取这个文件,并将词汇存储在一个数据结构中,例如列表或集合,以便后续使用。
def load_word_list(file_path):
with open(file_path, 'r', encoding='utf-8') as file:
word_list = [line.strip() for line in file.readlines()]
return word_list
cet4_words = load_word_list('cet4_word_list.txt')
二、读取文本和预处理
读取需要统计的文本,进行预处理。这包括去除标点符号、转换为小写等操作,以确保统计的准确性。
import re
def preprocess_text(text):
# 将文本转换为小写
text = text.lower()
# 去除标点符号
text = re.sub(r'[^\w\s]', '', text)
return text
with open('sample_text.txt', 'r', encoding='utf-8') as file:
text = file.read()
preprocessed_text = preprocess_text(text)
三、分词
分词是将文本拆分成单个词汇的过程。在英文处理中,可以使用简单的空格分词方法。
def tokenize(text):
return text.split()
tokens = tokenize(preprocessed_text)
四、统计词频
使用计数器统计词汇的频率,并与四级词汇表进行比较,以统计出四级词汇在文本中的出现频率。
from collections import Counter
def count_cet4_words(tokens, cet4_words):
token_counter = Counter(tokens)
cet4_word_count = {word: token_counter[word] for word in cet4_words if word in token_counter}
return cet4_word_count
cet4_word_count = count_cet4_words(tokens, cet4_words)
五、输出结果
输出四级词汇及其出现频率。
for word, count in cet4_word_count.items():
print(f'{word}: {count}')
六、进一步优化和扩展
1、使用自然语言处理库
在实际应用中,可能需要更强大的分词和处理能力。可以使用如nltk、spaCy等自然语言处理库来增强文本处理能力。
import spacy
nlp = spacy.load('en_core_web_sm')
def advanced_tokenize(text):
doc = nlp(text)
return [token.text for token in doc]
tokens = advanced_tokenize(preprocessed_text)
2、处理不同形式的词汇
考虑到四级词汇的不同形式,如复数、过去式等,可以使用词干提取和词形还原技术。
from nltk.stem import PorterStemmer
stemmer = PorterStemmer()
def stem_tokens(tokens):
return [stemmer.stem(token) for token in tokens]
stemmed_tokens = stem_tokens(tokens)
3、处理大规模数据
对于大规模文本数据,可以使用更高效的数据处理方法,如生成器和多线程处理。
def read_large_file(file_path):
with open(file_path, 'r', encoding='utf-8') as file:
for line in file:
yield line
def process_large_file(file_path, cet4_words):
cet4_word_count = Counter()
for line in read_large_file(file_path):
preprocessed_line = preprocess_text(line)
tokens = tokenize(preprocessed_line)
cet4_word_count.update(count_cet4_words(tokens, cet4_words))
return cet4_word_count
cet4_word_count = process_large_file('large_text_file.txt', cet4_words)
七、结果分析与可视化
将统计结果进行可视化,可以更直观地展示四级词汇的分布情况。
import matplotlib.pyplot as plt
def plot_word_frequency(word_count):
words = list(word_count.keys())
counts = list(word_count.values())
plt.figure(figsize=(10, 5))
plt.bar(words, counts, color='skyblue')
plt.xlabel('Words')
plt.ylabel('Frequency')
plt.title('CET-4 Word Frequency')
plt.xticks(rotation=90)
plt.show()
plot_word_frequency(cet4_word_count)
八、总结
通过以上步骤,我们可以使用Python高效地统计四级词汇在文本中的出现频率。关键步骤包括读取词库、预处理文本、分词、统计词频等。进一步的优化和扩展可以提高处理大规模数据的效率,并增强文本处理能力。通过结果分析与可视化,可以更直观地展示统计结果。希望本文对您在使用Python进行四级词汇统计时有所帮助。
相关问答FAQs:
如何用Python来识别和统计四级词汇的频率?
使用Python统计四级词汇的频率,可以通过读取包含这些词汇的文件,使用字典或Counter类来记录每个词的出现次数。借助正则表达式,您可以有效地提取单词,并使用简单的循环来计算它们的频率。这种方法使得分析过程高效且易于实现。
有哪些Python库可以帮助我处理四级词汇的统计?
在进行四级词汇统计时,可以使用一些强大的Python库,例如Pandas用于数据处理、NumPy用于数值计算、以及NLTK或spaCy等自然语言处理库。这些工具可以帮助您更方便地清洗数据、分析词汇,并进行可视化展示。
如果我想将四级词汇统计结果可视化,有哪些推荐的图表库?
为了将四级词汇统计结果进行可视化,可以使用Matplotlib和Seaborn等图表库。这些库提供了丰富的图表类型,能够帮助您直观地展示词汇频率分布、词云等信息,使得数据分析更加生动易懂。通过图形化表现,您可以更清晰地识别出高频词汇和低频词汇。
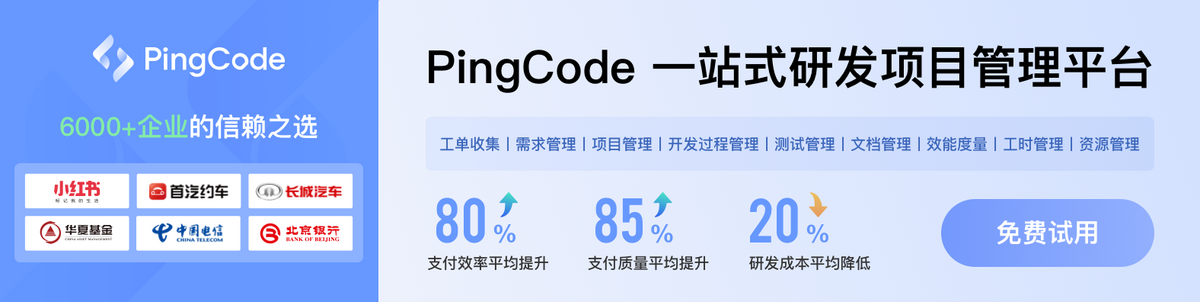