使用Python制作折线图的方法包括:导入必要的库、准备数据、使用matplotlib
库绘制折线图、添加标题和标签、显示图形。 其中,matplotlib
是一个非常强大的库,常用于数据可视化。下面将详细介绍如何使用matplotlib
库来制作折线图。
一、安装和导入必要的库
在开始之前,确保你已经安装了matplotlib
库。你可以通过以下命令安装:
pip install matplotlib
安装完成后,在你的Python脚本中导入该库:
import matplotlib.pyplot as plt
二、准备数据
在绘制折线图之前,首先需要准备好要展示的数据。假设我们有两个列表,分别表示时间和对应的温度数据:
time = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
temperature = [30, 32, 33, 31, 29, 35, 36, 34, 38, 37]
三、使用matplotlib
库绘制折线图
使用matplotlib
库的plot()
函数可以很方便地绘制折线图:
plt.plot(time, temperature)
四、添加标题和标签
为了让图表更具可读性,可以为图表添加标题和坐标轴标签:
plt.title('Temperature Over Time')
plt.xlabel('Time (hours)')
plt.ylabel('Temperature (°C)')
五、显示图形
最后,使用show()
函数来显示图表:
plt.show()
综合示例
将上述步骤整合在一起,完整的代码如下:
import matplotlib.pyplot as plt
准备数据
time = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
temperature = [30, 32, 33, 31, 29, 35, 36, 34, 38, 37]
绘制折线图
plt.plot(time, temperature)
添加标题和坐标轴标签
plt.title('Temperature Over Time')
plt.xlabel('Time (hours)')
plt.ylabel('Temperature (°C)')
显示图形
plt.show()
六、深入定制折线图
除了基础的折线图绘制,matplotlib
还提供了丰富的定制选项,可以满足不同的需求。
1、设置线条样式
你可以通过linestyle
参数来设置线条的样式,例如虚线、点线等:
plt.plot(time, temperature, linestyle='--') # 虚线
plt.plot(time, temperature, linestyle=':') # 点线
2、设置线条颜色和宽度
你可以通过color
和linewidth
参数来设置线条的颜色和宽度:
plt.plot(time, temperature, color='red', linewidth=2.0)
3、添加网格
为了更方便地读取数据,可以在图表中添加网格:
plt.grid(True)
4、添加数据点标记
你可以通过marker
参数来添加数据点标记,例如圆圈、方块等:
plt.plot(time, temperature, marker='o') # 圆圈标记
plt.plot(time, temperature, marker='s') # 方块标记
5、设置图例
在绘制多条折线时,可以添加图例来区分不同的数据集:
plt.plot(time, temperature, label='Temperature')
plt.legend()
综合示例(高级定制)
综合上述定制选项,完整的高级定制代码如下:
import matplotlib.pyplot as plt
准备数据
time = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
temperature = [30, 32, 33, 31, 29, 35, 36, 34, 38, 37]
绘制折线图,并设置样式
plt.plot(time, temperature, color='red', linestyle='--', linewidth=2.0, marker='o', label='Temperature')
添加标题和坐标轴标签
plt.title('Temperature Over Time')
plt.xlabel('Time (hours)')
plt.ylabel('Temperature (°C)')
添加网格
plt.grid(True)
添加图例
plt.legend()
显示图形
plt.show()
七、保存图形
除了显示图形外,你还可以将图形保存为文件。matplotlib
支持多种文件格式,例如PNG、PDF等。使用savefig()
函数可以将图形保存为文件:
plt.savefig('temperature_over_time.png') # 保存为PNG文件
plt.savefig('temperature_over_time.pdf') # 保存为PDF文件
八、总结
通过以上步骤和示例代码,我们详细介绍了如何使用Python制作折线图。首先,确保安装并导入matplotlib
库;其次,准备数据并使用plot()
函数绘制折线图;然后,添加标题、标签、网格、图例等,使图表更加美观和易于理解;最后,可以选择显示或保存图形。 通过这些方法,你可以轻松地使用Python制作各种样式的折线图,以满足不同的数据可视化需求。
相关问答FAQs:
如何使用Python生成折线图?
要在Python中生成折线图,可以使用Matplotlib库。首先,确保安装了Matplotlib,可以通过命令pip install matplotlib
进行安装。接下来,使用如下代码创建折线图:
import matplotlib.pyplot as plt
x = [1, 2, 3, 4, 5]
y = [2, 3, 5, 7, 11]
plt.plot(x, y)
plt.title('折线图示例')
plt.xlabel('X轴')
plt.ylabel('Y轴')
plt.show()
通过这段代码,您可以轻松绘制出折线图。
在Python中如何自定义折线图的样式?
使用Matplotlib时,可以自定义折线图的颜色、线型和标记。可以在plot
函数中使用参数来实现。例如:
plt.plot(x, y, color='red', linestyle='--', marker='o')
这将会生成红色、虚线的折线图,并在每个数据点上加上圆圈标记。通过这种方式,可以使折线图更具个性和易读性。
如何将生成的折线图保存为图片文件?
在使用Matplotlib生成折线图后,可以通过savefig
函数将图像保存为文件。可以使用以下代码进行保存:
plt.savefig('折线图.png')
可以指定文件名和格式(如PNG或JPEG),这样便于后续使用或分享。
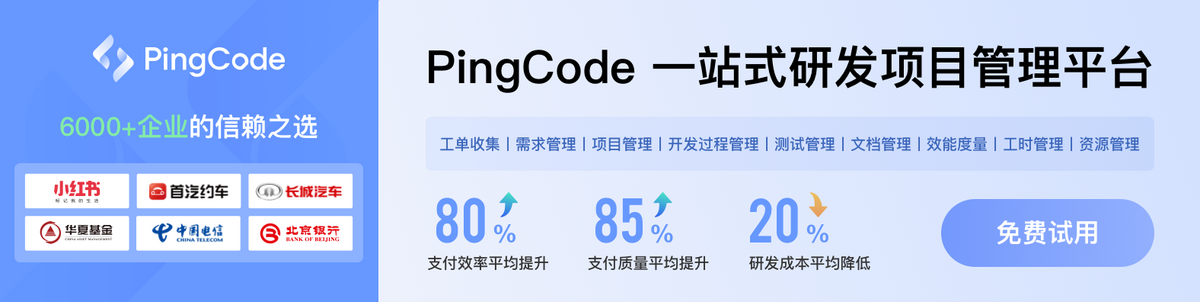