Python如何在输出中换行符,使用\n、使用多行字符串、使用print函数中的end参数
在Python中,处理输出中的换行符有多种方式。使用\n、使用多行字符串、使用print函数中的end参数是最常见的方法。这些方法都各自有其适用的场景和优点。下面,我们将详细介绍这些方法,并探讨它们在不同情境下的应用。
一、使用\n
使用\n是最简单、最直接的方式来在输出中添加换行符。它是一个转义字符,表示换行。在字符串中插入\n,Python会在输出时将其转换为换行。
print("Hello\nWorld")
上述代码会输出:
Hello
World
二、使用多行字符串
多行字符串是另一种方便的方法,特别适用于输出较长的文本。多行字符串使用三重引号(''' 或 """)包围,字符串中的换行会被保留。
print("""Hello
World""")
上述代码会输出:
Hello
World
这种方法在处理多段文本时尤为有用,因为它不需要手动插入\n。
三、使用print函数中的end参数
print函数默认在每次调用后都会添加一个换行符,可以通过修改end参数来控制这一行为。如果希望在一行中输出多个print的内容,可以设置end参数为空字符串或其他字符。
print("Hello", end='')
print(" World")
上述代码会输出:
Hello World
通过设置end参数为空字符串,两个print函数的输出会连在一起,形成一行输出。
四、结合使用多种方法
在实际编程中,有时需要结合使用多种方法来实现复杂的输出需求。例如,可以在长文本中使用多行字符串,同时在特定位置插入\n来控制换行。
print("""Hello
this is a long text with a manual newline\nright here.""")
五、在循环中使用\n
在循环中使用\n可以方便地控制每次迭代的输出格式。例如,当需要输出一个矩阵或表格时,可以在每行的末尾添加\n。
matrix = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
for row in matrix:
for element in row:
print(element, end=' ')
print('\n')
上述代码会输出:
1 2 3
4 5 6
7 8 9
在每行输出后,使用print('\n')来换行。
六、在字符串格式化中使用\n
Python提供了多种字符串格式化方式,如f字符串、str.format()和%格式化。在这些方法中,也可以使用\n来添加换行。
name = "Alice"
age = 30
print(f"Name: {name}\nAge: {age}")
上述代码会输出:
Name: Alice
Age: 30
七、在文件输出中使用\n
在将内容写入文件时,\n同样可以用来控制换行。无论是使用open函数还是其他文件操作库,\n都能确保文本格式正确。
with open('output.txt', 'w') as file:
file.write("Hello\nWorld")
上述代码会在output.txt文件中写入:
Hello
World
八、在函数返回值中使用\n
有时需要在函数返回的字符串中包含换行符,以确保在调用函数时能得到正确格式的输出。
def generate_greeting(name):
return f"Hello, {name}\nWelcome to the community!"
print(generate_greeting("Alice"))
上述代码会输出:
Hello, Alice
Welcome to the community!
九、在日志记录中使用\n
在记录日志时,使用\n可以使日志信息更具可读性。Python的logging模块支持在日志消息中使用换行符。
import logging
logging.basicConfig(level=logging.INFO)
logging.info("Starting the process...\nProcess started successfully.")
上述代码会在日志中记录:
INFO:root:Starting the process...
Process started successfully.
十、在正则表达式中使用\n
在正则表达式中,\n可以用来匹配换行符。例如,当需要匹配多行字符串中的特定模式时,\n是一个有力的工具。
import re
text = "Hello\nWorld"
pattern = r"Hello\nWorld"
match = re.match(pattern, text)
if match:
print("Match found!")
上述代码会输出:
Match found!
十一、在GUI应用中使用\n
在开发GUI应用时,\n可以用来控制文本控件中的内容格式。例如,在Tkinter中可以使用\n来添加多行文本。
import tkinter as tk
root = tk.Tk()
label = tk.Label(root, text="Hello\nWorld")
label.pack()
root.mainloop()
上述代码会在Tkinter窗口中显示:
Hello
World
十二、在网络通信中使用\n
在网络编程中,\n常用于分隔消息或命令。例如,在基于TCP的聊天应用中,\n可以用来区分不同的消息。
import socket
with socket.socket(socket.AF_INET, socket.SOCK_STREAM) as s:
s.connect(('localhost', 12345))
s.sendall(b'Hello\nWorld')
在接收端,可以使用\n来解析消息。
十三、在数据处理和分析中使用\n
在数据处理和分析时,\n可以用来格式化输出结果,使其更易于阅读。例如,在处理大型数据集时,可以使用\n来分隔不同的部分。
data = [1, 2, 3, 4, 5]
result = "Data:\n"
for item in data:
result += str(item) + '\n'
print(result)
上述代码会输出:
Data:
1
2
3
4
5
十四、在模板引擎中使用\n
在使用模板引擎(如Jinja2)生成HTML或其他文本内容时,\n可以用来确保生成的内容格式正确。
from jinja2 import Template
template = Template("Hello\n{{ name }}")
print(template.render(name="World"))
上述代码会输出:
Hello
World
十五、在API响应中使用\n
在开发API时,\n可以用来构建多行的响应消息,特别是在返回复杂的文本信息时。
from flask import Flask, jsonify
app = Flask(__name__)
@app.route('/greeting')
def greeting():
return jsonify(message="Hello\nWorld")
if __name__ == '__main__':
app.run()
上述代码会在访问/greeting路由时返回包含换行符的JSON响应:
{
"message": "Hello\nWorld"
}
十六、在测试中使用\n
在编写单元测试或集成测试时,\n可以用来验证多行输出的正确性。
import unittest
class TestOutput(unittest.TestCase):
def test_multiline_output(self):
output = "Hello\nWorld"
self.assertEqual(output, "Hello\nWorld")
if __name__ == '__main__':
unittest.main()
上述代码会验证输出中包含正确的换行符。
十七、在国际化和本地化中使用\n
在处理多语言文本时,\n可以确保不同语言版本的文本格式一致,特别是在包含多行文本时。
translations = {
'en': "Hello\nWorld",
'es': "Hola\nMundo",
}
language = 'es'
print(translations[language])
上述代码会根据选择的语言输出:
Hola
Mundo
十八、在命令行工具中使用\n
在开发命令行工具时,\n可以用来格式化输出,使其更具可读性和用户友好性。
import argparse
parser = argparse.ArgumentParser(description="Example script")
parser.add_argument('--name', type=str, help="Your name")
args = parser.parse_args()
print(f"Hello, {args.name}\nWelcome to the command line tool!")
运行上述脚本并传入参数:
python script.py --name Alice
会输出:
Hello, Alice
Welcome to the command line tool!
十九、在处理配置文件中使用\n
在读取和解析配置文件时,\n可以用来分隔不同的配置项,确保配置文件格式正确。
config = """
[settings]
name = Alice
age = 30
"""
print(config)
上述代码会输出:
[settings]
name = Alice
age = 30
二十、在数据序列化和反序列化中使用\n
在序列化和反序列化数据(如JSON或XML)时,\n可以用来控制输出格式,使数据更具可读性。
import json
data = {
'name': 'Alice',
'age': 30,
}
json_data = json.dumps(data, indent=4)
print(json_data)
上述代码会输出:
{
"name": "Alice",
"age": 30
}
通过indent参数,输出的JSON数据格式良好,易于阅读。
总结
通过上述多种方法,Python开发者可以灵活地在输出中添加换行符,满足不同场景的需求。使用\n、使用多行字符串、使用print函数中的end参数是最常见的方法,但在实际应用中,还可以结合其他技术和库,确保输出的文本格式正确、易读。在不同的编程任务中,选择合适的方法来处理换行符,可以大大提高代码的可读性和维护性。
相关问答FAQs:
如何在Python中使用换行符?
在Python中,换行符通常使用\n
来表示。当你想要在输出中插入换行时,只需在字符串中添加\n
即可。例如,使用print("Hello\nWorld")
将分别在两行中输出“Hello”和“World”。
可以在文件中使用换行符吗?
当然可以!在写入文件时,可以使用\n
来控制文本的换行。例如,使用with open('file.txt', 'w') as f:
配合f.write("Hello\nWorld")
可以在文件中创建换行。打开文件后,你会看到“Hello”和“World”分别位于不同的行上。
如何在多行字符串中使用换行符?
在Python中,可以使用三重引号('''
或 """
)来定义多行字符串,这样可以在字符串中自然换行,而无需手动插入\n
。例如,使用multiline_string = """Hello\nWorld"""
或multiline_string = '''Hello\nWorld'''
,输出时也会自动换行。
是否可以自定义换行符?
Python允许你使用不同的换行符,除了标准的\n
,还可以使用\r\n
(适用于Windows系统)或其他字符。通过在字符串中指定不同的换行符,你可以根据需要调整输出格式。
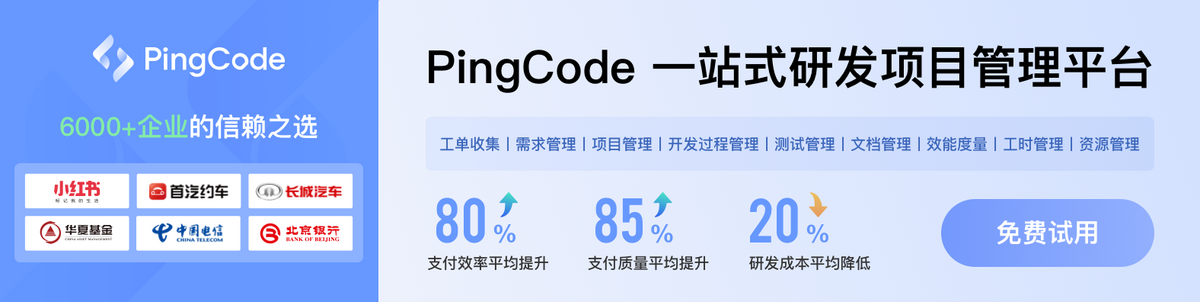