如何用Python爬取微信公众号
要用Python爬取微信公众号,可以使用Selenium、Requests、BeautifulSoup、微信公众号第三方API。其中,Selenium 是一种用于自动化测试的工具,它可以模拟用户操作浏览器,适用于需要登录和处理JavaScript的场景;Requests 可以发送HTTP请求,获取网页内容;BeautifulSoup 可以解析HTML文档,提取所需的数据;微信公众号第三方API 则可以直接获取公众号的一些数据。本文将详细介绍如何利用Selenium来爬取微信公众号的文章。
一、准备工作
在开始爬取微信公众号之前,需要进行一些准备工作:
- 安装Python及其相关库。
- 注册微信公众号。
- 获取目标微信公众号的文章链接。
1. 安装Python及其相关库
首先,需要确保系统中已经安装了Python。如果没有,可以从Python官网(https://www.python.org/)下载并安装最新版本的Python。安装完成后,可以使用以下命令来安装所需的库:
pip install selenium requests beautifulsoup4
2. 注册微信公众号
在进行爬取之前,需要注册一个微信公众号,并获取相关的登录信息。这个步骤较为简单,只需按照微信公众号的注册流程进行操作即可。
3. 获取目标微信公众号的文章链接
在开始爬取之前,需要获取目标微信公众号的文章链接。这可以通过微信公众号的后台管理界面获取。
二、使用Selenium模拟登录
由于微信公众号的文章内容需要登录才能访问,因此需要使用Selenium模拟登录操作。以下是一个示例代码,用于模拟登录微信公众号:
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.common.keys import Keys
import time
设置浏览器驱动路径
driver_path = 'path/to/chromedriver'
创建浏览器对象
driver = webdriver.Chrome(driver_path)
打开微信公众号登录页面
driver.get('https://mp.weixin.qq.com/')
输入用户名和密码
username = driver.find_element(By.NAME, 'account')
username.send_keys('your_username')
password = driver.find_element(By.NAME, 'password')
password.send_keys('your_password')
点击登录按钮
login_button = driver.find_element(By.CLASS_NAME, 'btn_login')
login_button.click()
等待登录完成
time.sleep(5)
关闭浏览器
driver.quit()
以上代码使用Selenium模拟了微信公众号的登录操作。在实际使用中,需要替换your_username
和your_password
为实际的用户名和密码。同时,还需要将path/to/chromedriver
替换为实际的Chromedriver路径。
三、爬取文章内容
在成功登录之后,可以开始爬取微信公众号的文章内容。以下是一个示例代码,用于获取文章列表并提取文章内容:
from selenium import webdriver
from bs4 import BeautifulSoup
import time
设置浏览器驱动路径
driver_path = 'path/to/chromedriver'
创建浏览器对象
driver = webdriver.Chrome(driver_path)
打开微信公众号文章页面
driver.get('https://mp.weixin.qq.com/')
模拟登录操作(同上)
...
等待页面加载完成
time.sleep(5)
获取文章列表
articles = driver.find_elements(By.CSS_SELECTOR, 'div.article_list')
for article in articles:
# 获取文章链接
link = article.find_element(By.TAG_NAME, 'a').get_attribute('href')
# 打开文章链接
driver.get(link)
# 获取文章内容
content = driver.page_source
# 解析文章内容
soup = BeautifulSoup(content, 'html.parser')
title = soup.find('h1', {'class': 'rich_media_title'}).text.strip()
body = soup.find('div', {'class': 'rich_media_content'}).text.strip()
# 打印文章标题和内容
print(f'Title: {title}')
print(f'Content: {body}')
# 返回文章列表页面
driver.back()
# 等待页面加载完成
time.sleep(5)
关闭浏览器
driver.quit()
以上代码使用Selenium获取了微信公众号的文章列表,并使用BeautifulSoup解析了文章内容。在实际使用中,可以根据需要进行修改和扩展。
四、处理验证码和反爬虫机制
在实际操作中,可能会遇到验证码和反爬虫机制。以下是一些处理验证码和反爬虫机制的建议:
1. 处理验证码
可以使用第三方验证码识别服务,如打码兔、超级鹰等,来自动识别验证码。以下是一个示例代码:
from selenium import webdriver
from selenium.webdriver.common.by import By
import requests
import time
设置浏览器驱动路径
driver_path = 'path/to/chromedriver'
创建浏览器对象
driver = webdriver.Chrome(driver_path)
打开微信公众号登录页面
driver.get('https://mp.weixin.qq.com/')
输入用户名和密码
username = driver.find_element(By.NAME, 'account')
username.send_keys('your_username')
password = driver.find_element(By.NAME, 'password')
password.send_keys('your_password')
获取验证码图片
captcha_img = driver.find_element(By.ID, 'captcha_img')
captcha_src = captcha_img.get_attribute('src')
下载验证码图片
response = requests.get(captcha_src)
with open('captcha.jpg', 'wb') as f:
f.write(response.content)
使用第三方验证码识别服务
captcha_code = recognize_captcha('captcha.jpg')
输入验证码
captcha_input = driver.find_element(By.NAME, 'captcha')
captcha_input.send_keys(captcha_code)
点击登录按钮
login_button = driver.find_element(By.CLASS_NAME, 'btn_login')
login_button.click()
等待登录完成
time.sleep(5)
关闭浏览器
driver.quit()
2. 处理反爬虫机制
可以使用代理、请求头伪装、延时等方法来应对反爬虫机制。以下是一些示例代码:
import requests
from fake_useragent import UserAgent
使用代理
proxies = {
'http': 'http://your_proxy',
'https': 'https://your_proxy',
}
伪装请求头
ua = UserAgent()
headers = {
'User-Agent': ua.random,
}
发送请求
response = requests.get('https://mp.weixin.qq.com/', proxies=proxies, headers=headers)
解析响应内容
content = response.text
五、保存爬取的数据
在爬取到微信公众号的文章内容后,可以将其保存到本地文件或数据库中。以下是一些示例代码:
1. 保存到本地文件
# 保存到本地文件
with open('articles.txt', 'a', encoding='utf-8') as f:
f.write(f'Title: {title}\n')
f.write(f'Content: {body}\n')
f.write('\n')
2. 保存到数据库
import sqlite3
连接数据库
conn = sqlite3.connect('articles.db')
cursor = conn.cursor()
创建表
cursor.execute('''
CREATE TABLE IF NOT EXISTS articles (
id INTEGER PRIMARY KEY AUTOINCREMENT,
title TEXT,
content TEXT
)
''')
插入数据
cursor.execute('''
INSERT INTO articles (title, content)
VALUES (?, ?)
''', (title, body))
提交事务
conn.commit()
关闭连接
conn.close()
六、优化爬虫性能
在爬虫运行过程中,可能会遇到性能瓶颈。以下是一些优化爬虫性能的建议:
1. 使用多线程或多进程
可以使用多线程或多进程来提高爬虫的并发能力。以下是一个示例代码:
import threading
def crawl_article(link):
# 打开文章链接
driver.get(link)
# 获取文章内容
content = driver.page_source
# 解析文章内容
soup = BeautifulSoup(content, 'html.parser')
title = soup.find('h1', {'class': 'rich_media_title'}).text.strip()
body = soup.find('div', {'class': 'rich_media_content'}).text.strip()
# 打印文章标题和内容
print(f'Title: {title}')
print(f'Content: {body}')
获取文章列表
articles = driver.find_elements(By.CSS_SELECTOR, 'div.article_list')
创建线程池
threads = []
for article in articles:
link = article.find_element(By.TAG_NAME, 'a').get_attribute('href')
thread = threading.Thread(target=crawl_article, args=(link,))
threads.append(thread)
启动线程
for thread in threads:
thread.start()
等待线程完成
for thread in threads:
thread.join()
2. 使用异步IO
可以使用异步IO来提高爬虫的并发能力。以下是一个示例代码:
import aiohttp
import asyncio
from bs4 import BeautifulSoup
async def crawl_article(session, link):
async with session.get(link) as response:
content = await response.text()
soup = BeautifulSoup(content, 'html.parser')
title = soup.find('h1', {'class': 'rich_media_title'}).text.strip()
body = soup.find('div', {'class': 'rich_media_content'}).text.strip()
print(f'Title: {title}')
print(f'Content: {body}')
async def main():
async with aiohttp.ClientSession() as session:
tasks = []
for article in articles:
link = article.find_element(By.TAG_NAME, 'a').get_attribute('href')
task = asyncio.create_task(crawl_article(session, link))
tasks.append(task)
await asyncio.gather(*tasks)
获取文章列表
articles = driver.find_elements(By.CSS_SELECTOR, 'div.article_list')
运行异步任务
asyncio.run(main())
七、总结
本文详细介绍了如何用Python爬取微信公众号,包括准备工作、使用Selenium模拟登录、爬取文章内容、处理验证码和反爬虫机制、保存爬取的数据、优化爬虫性能等方面的内容。通过本文的介绍,可以帮助读者掌握如何使用Python爬取微信公众号,并应对实际操作中可能遇到的问题。希望本文对大家有所帮助。
相关问答FAQs:
如何选择合适的Python库来爬取微信公众号内容?
在爬取微信公众号内容时,选择合适的Python库非常重要。常用的库包括requests、BeautifulSoup和Scrapy等。requests库用于发送HTTP请求,获取网页内容;BeautifulSoup则用于解析HTML文档,提取所需的数据;Scrapy是一个功能强大的爬虫框架,适用于大规模数据抓取。根据你的需求,可以选择单一库或者组合使用。
爬取微信公众号时需要注意哪些法律法规?
在进行数据爬取时,需遵循相关法律法规,确保不侵犯他人知识产权和隐私权。根据《网络安全法》和《著作权法》,未经授权抓取他人的原创内容可能会导致法律风险。因此,建议在爬取之前检查公众号的使用条款,确保遵循相关规定,并考虑使用官方API获取数据。
如何处理微信公众号的反爬虫机制?
微信公众号通常会采取一些反爬虫措施,如验证登录、限制访问频率等。为应对这些机制,可以使用动态代理池,随机更换IP地址,避免被封禁;设置请求间隔时间,模拟人类浏览行为;此外,使用浏览器自动化工具如Selenium,可以模拟真实用户的操作,从而绕过一些基本的反爬虫措施。
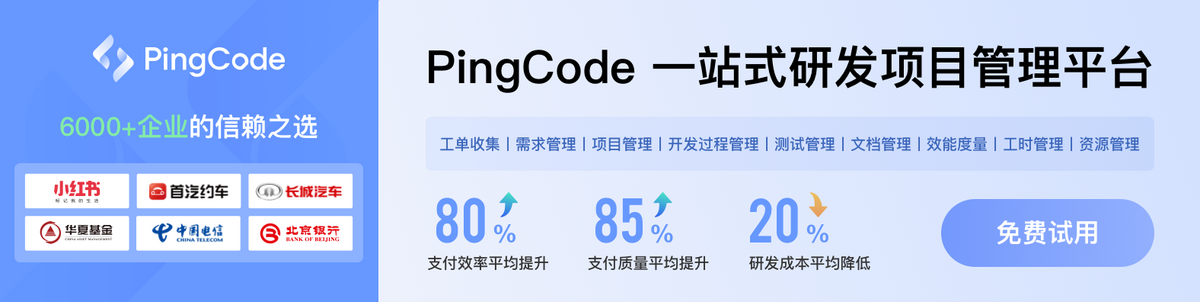