通过使用反缩进、使用空行、结束循环或条件、使用函数或类定义 可以结束Python中的缩进代码块。反缩进 是最常见的方法,通过减少缩进级别来结束代码块。反缩进 意味着减少代码前面的空格数量,这会告诉Python解释器当前代码块已经结束。
在Python中,缩进是用来表示代码块的边界,而不是使用花括号或其他符号。这使得代码的可读性更强,但也需要特别注意缩进的正确性。要结束一个缩进代码块,只需减少缩进的级别。例如,如果一个代码块缩进了四个空格,要结束它,只需回到前一个缩进级别即可。
一、反缩进
反缩进是最直接的方法,通过减少缩进级别来结束代码块。例如,如果一个代码块缩进了四个空格,要结束它,只需回到前一个缩进级别即可。
if condition:
# Start of code block
print("This is inside the block")
End of code block
print("This is outside the block")
在上面的例子中,print("This is inside the block")
是一个缩进代码块的部分。当我们减少缩进,回到前一个级别时,表示代码块结束。print("This is outside the block")
不再属于缩进代码块。
二、使用空行
在某些情况下,使用空行可以帮助读者更容易地理解代码块的结束位置,尽管这在技术上并不会影响代码的运行。
def my_function():
print("This is inside the function")
# More code here
Empty line to visually separate the function block
print("This is outside the function")
在上面的例子中,使用空行将函数内部的代码与外部代码视觉上分离开来。尽管空行并不会真正结束代码块,但它有助于提高代码的可读性。
三、结束循环或条件
在循环或条件语句中,通过结束循环或条件来结束代码块。例如,使用 break
语句来结束循环,或者通过条件判断来结束 if
语句。
for i in range(5):
if i == 3:
break # End of code block
print(i)
print("This is outside the loop")
在上面的例子中,当 i
等于 3 时,break
语句结束了循环,代码块随之结束。print("This is outside the loop")
不再属于循环代码块。
四、使用函数或类定义
通过定义函数或类,可以将代码块封装在函数或类的内部。当函数或类定义结束时,代码块也随之结束。
def my_function():
print("This is inside the function")
# More code here
End of function definition
print("This is outside the function")
class MyClass:
def __init__(self):
print("This is inside the class")
# More code here
End of class definition
print("This is outside the class")
在上面的例子中,函数 my_function
和类 MyClass
定义了各自的代码块。当函数或类定义结束时,代码块也随之结束。print("This is outside the function")
和 print("This is outside the class")
不再属于函数或类的代码块。
五、使用上下文管理器
上下文管理器(Context Manager)是一种用于管理资源的编程结构,通过 with
语句来使用。上下文管理器会在代码块结束时自动释放资源,例如文件、网络连接等。
with open('file.txt', 'r') as file:
content = file.read()
print("This is inside the context manager")
End of context manager
print("This is outside the context manager")
在上面的例子中,with open('file.txt', 'r') as file
定义了一个上下文管理器。上下文管理器内部的代码块在 with
语句缩进级别内,当缩进结束时,代码块也随之结束。print("This is outside the context manager")
不再属于上下文管理器的代码块。
六、使用异常处理
异常处理代码块使用 try
、except
和 finally
关键字。通过结束异常处理代码块,可以结束相应的缩进代码块。
try:
result = 1 / 0
except ZeroDivisionError:
print("This is inside the exception handling block")
finally:
print("This is inside the finally block")
End of exception handling block
print("This is outside the exception handling block")
在上面的例子中,try
、except
和 finally
定义了异常处理代码块。当 try
、except
和 finally
代码块结束时,代码块也随之结束。print("This is outside the exception handling block")
不再属于异常处理代码块。
七、使用迭代器和生成器
通过使用迭代器和生成器,可以定义并结束一个代码块。迭代器和生成器通常用于处理大数据集或流式数据。
def my_generator():
for i in range(5):
yield i
print("This is inside the generator")
End of generator definition
for value in my_generator():
print(value)
print("This is outside the generator")
在上面的例子中,my_generator
函数定义了一个生成器。当生成器函数结束时,代码块也随之结束。print("This is outside the generator")
不再属于生成器代码块。
八、使用内联代码
在某些情况下,可以通过将代码放在同一行内来结束缩进代码块。例如,使用 if
、else
和 for
语句。
if condition: print("This is inside the block")
End of inline block
print("This is outside the block")
在上面的例子中,if condition: print("This is inside the block")
是一个内联代码块。当内联代码块结束时,代码块也随之结束。print("This is outside the block")
不再属于内联代码块。
总结来说,结束Python中的缩进代码块有多种方法,包括反缩进、使用空行、结束循环或条件、使用函数或类定义、使用上下文管理器、使用异常处理、使用迭代器和生成器以及使用内联代码。正确地结束代码块可以提高代码的可读性和可维护性。
相关问答FAQs:
如何在Python中正确使用缩进?
在Python中,缩进是语法的一部分,用于定义代码块的开始和结束。通常,使用四个空格或一个制表符(Tab)来进行缩进。确保在一个代码块内使用一致的缩进方式,可以有效避免IndentationError错误。
如果我在Python代码中混合使用空格和制表符会发生什么?
混合使用空格和制表符可能导致代码可读性降低,并可能引发IndentationError。建议在编辑器中统一设置缩进方式,以保持代码的一致性和可读性。
如何检查和修复Python代码中的缩进错误?
可以通过阅读错误提示信息来查找缩进问题。许多现代代码编辑器和IDE提供了自动缩进功能,能够帮助您调整代码结构。此外,运行代码时会提示缩进错误,您可以根据提示逐行检查并修正。
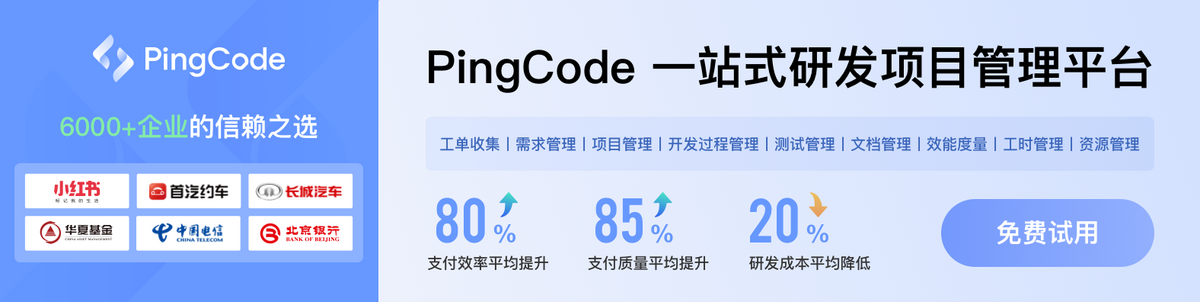