使用Python绘制旋转正方形,可以通过多个方法来实现。主要方法包括:使用Matplotlib库、使用Pygame库、使用Turtle库。 这篇文章将详细介绍如何使用这些方法来绘制和旋转正方形。特别是,Matplotlib库提供了强大的绘图功能,使其成为实现这一目标的理想选择。接下来,我将详细介绍使用Matplotlib库实现旋转正方形的步骤。
一、MATPLOTLIB库
Matplotlib是Python中最流行的数据可视化库之一。它提供了丰富的绘图功能,可以轻松绘制各种图形,包括旋转正方形。
1、安装Matplotlib库
首先,你需要确保安装了Matplotlib库。如果没有安装,可以使用以下命令进行安装:
pip install matplotlib
2、绘制基本正方形
接下来,我们将使用Matplotlib库来绘制一个基本的正方形。
import matplotlib.pyplot as plt
import numpy as np
定义正方形的顶点
square = np.array([
[1, 1],
[-1, 1],
[-1, -1],
[1, -1],
[1, 1]
])
绘制正方形
plt.plot(square[:, 0], square[:, 1])
plt.axis('equal')
plt.show()
在这段代码中,我们定义了一个正方形的顶点,并使用plt.plot
函数绘制正方形。plt.axis('equal')
确保x轴和y轴的比例相同。
3、实现旋转功能
为了旋转正方形,我们需要定义一个旋转矩阵,并将其应用于正方形的顶点。旋转矩阵的定义如下:
[ R(\theta) = \begin{bmatrix}
\cos(\theta) & -\sin(\theta) \
\sin(\theta) & \cos(\theta)
\end{bmatrix} ]
其中,(\theta)是旋转角度。
import matplotlib.pyplot as plt
import numpy as np
定义旋转矩阵
def rotation_matrix(theta):
return np.array([
[np.cos(theta), -np.sin(theta)],
[np.sin(theta), np.cos(theta)]
])
定义正方形的顶点
square = np.array([
[1, 1],
[-1, 1],
[-1, -1],
[1, -1],
[1, 1]
])
旋转正方形
theta = np.radians(45) # 旋转角度(例如45度)
rotated_square = square.dot(rotation_matrix(theta))
绘制旋转后的正方形
plt.plot(rotated_square[:, 0], rotated_square[:, 1])
plt.axis('equal')
plt.show()
在这段代码中,我们定义了一个旋转矩阵函数,并使用该函数旋转正方形的顶点。
4、创建动态旋转动画
我们还可以使用Matplotlib的动画功能来创建一个正方形旋转的动画。
import matplotlib.pyplot as plt
import numpy as np
import matplotlib.animation as animation
定义旋转矩阵
def rotation_matrix(theta):
return np.array([
[np.cos(theta), -np.sin(theta)],
[np.sin(theta), np.cos(theta)]
])
定义正方形的顶点
square = np.array([
[1, 1],
[-1, 1],
[-1, -1],
[1, -1],
[1, 1]
])
创建图形和轴
fig, ax = plt.subplots()
line, = ax.plot([], [], lw=2)
ax.axis('equal')
ax.set_xlim(-2, 2)
ax.set_ylim(-2, 2)
初始化函数
def init():
line.set_data([], [])
return line,
动画更新函数
def update(frame):
theta = np.radians(frame)
rotated_square = square.dot(rotation_matrix(theta))
line.set_data(rotated_square[:, 0], rotated_square[:, 1])
return line,
创建动画
ani = animation.FuncAnimation(fig, update, frames=np.linspace(0, 360, 360), init_func=init, blit=True)
plt.show()
这段代码使用animation.FuncAnimation
函数创建一个正方形旋转的动画。update
函数用于在每一帧中更新正方形的旋转角度。
二、PYGAME库
Pygame是一个跨平台的Python模块,用于编写视频游戏。它包括计算机图形和声音库。Pygame也可以用来绘制和旋转正方形。
1、安装Pygame库
首先,确保安装了Pygame库。如果没有安装,可以使用以下命令进行安装:
pip install pygame
2、绘制基本正方形
接下来,使用Pygame库绘制一个基本的正方形。
import pygame
import sys
初始化Pygame
pygame.init()
定义颜色
WHITE = (255, 255, 255)
BLACK = (0, 0, 0)
设置屏幕大小
size = (400, 400)
screen = pygame.display.set_mode(size)
pygame.display.set_caption("Rotating Square")
定义正方形顶点
square = [(100, 100), (200, 100), (200, 200), (100, 200)]
主循环
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
# 填充屏幕背景
screen.fill(WHITE)
# 绘制正方形
pygame.draw.polygon(screen, BLACK, square)
# 刷新屏幕
pygame.display.flip()
退出Pygame
pygame.quit()
sys.exit()
这段代码使用Pygame绘制一个基本的正方形,并显示在屏幕上。
3、实现旋转功能
为了旋转正方形,我们需要定义一个旋转函数,并将其应用于正方形的顶点。
import pygame
import sys
import math
初始化Pygame
pygame.init()
定义颜色
WHITE = (255, 255, 255)
BLACK = (0, 0, 0)
设置屏幕大小
size = (400, 400)
screen = pygame.display.set_mode(size)
pygame.display.set_caption("Rotating Square")
定义旋转函数
def rotate_point(point, angle, center):
angle = math.radians(angle)
x, y = point
cx, cy = center
x -= cx
y -= cy
new_x = x * math.cos(angle) - y * math.sin(angle) + cx
new_y = x * math.sin(angle) + y * math.cos(angle) + cy
return new_x, new_y
定义正方形顶点
square = [(100, 100), (200, 100), (200, 200), (100, 200)]
center = (150, 150)
angle = 0
主循环
running = True
clock = pygame.time.Clock()
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
# 填充屏幕背景
screen.fill(WHITE)
# 旋转正方形
rotated_square = [rotate_point(point, angle, center) for point in square]
angle += 1
# 绘制旋转后的正方形
pygame.draw.polygon(screen, BLACK, rotated_square)
# 刷新屏幕
pygame.display.flip()
clock.tick(60)
退出Pygame
pygame.quit()
sys.exit()
这段代码定义了一个旋转函数,并在主循环中使用该函数旋转正方形的顶点。
三、TURTLE库
Turtle是Python中一个简单的图形库,适合初学者学习编程。它允许用户通过控制一个“海龟”在屏幕上绘制图形。
1、绘制基本正方形
首先,使用Turtle库绘制一个基本的正方形。
import turtle
初始化Turtle
t = turtle.Turtle()
绘制正方形
for _ in range(4):
t.forward(100)
t.right(90)
保持窗口打开
turtle.done()
这段代码使用Turtle库绘制一个基本的正方形,并显示在屏幕上。
2、实现旋转功能
为了旋转正方形,我们可以在每次绘制正方形前旋转Turtle的方向。
import turtle
初始化Turtle
t = turtle.Turtle()
定义旋转函数
def rotate_square(turtle, angle):
turtle.right(angle)
绘制旋转正方形
angle = 0
while True:
t.clear()
t.penup()
t.goto(-50, 50)
t.pendown()
rotate_square(t, angle)
for _ in range(4):
t.forward(100)
t.right(90)
angle += 1
保持窗口打开
turtle.done()
这段代码在每次绘制正方形前旋转Turtle的方向,从而实现正方形的旋转效果。
结论
本文详细介绍了如何使用Python绘制和旋转正方形,重点介绍了Matplotlib、Pygame和Turtle库的实现方法。每种方法都有其独特的优势和应用场景,可以根据具体需求选择合适的方法。希望这篇文章能帮助你更好地理解和应用这些技术。
相关问答FAQs:
如何使用Python绘制一个简单的正方形?
可以使用Matplotlib库来绘制正方形。首先,确保安装了Matplotlib。接着,你可以创建一个正方形的坐标,并用plt.plot()
函数绘制它。代码示例如下:
import matplotlib.pyplot as plt
# 定义正方形的坐标
square = [[0, 1, 1, 0, 0], [0, 0, 1, 1, 0]]
# 绘制正方形
plt.plot(square[0], square[1])
plt.axis('equal') # 保持比例
plt.show()
如何实现正方形的旋转效果?
要实现旋转效果,可以使用NumPy库来对正方形的坐标进行变换。通过定义旋转角度和计算旋转矩阵,可以生成旋转后的坐标。以下是一个基本的示例:
import numpy as np
import matplotlib.pyplot as plt
# 定义旋转角度(以弧度为单位)
angle = np.pi / 4 # 45度
cos_angle = np.cos(angle)
sin_angle = np.sin(angle)
# 定义正方形的坐标
square = np.array([[0, 1, 1, 0, 0], [0, 0, 1, 1, 0]])
# 旋转矩阵
rotation_matrix = np.array([[cos_angle, -sin_angle], [sin_angle, cos_angle]])
# 应用旋转矩阵
rotated_square = rotation_matrix @ square
# 绘制旋转后的正方形
plt.plot(rotated_square[0], rotated_square[1])
plt.axis('equal')
plt.show()
在绘制旋转正方形时,如何调整旋转中心?
默认情况下,旋转是围绕原点进行的。如果希望围绕正方形的中心进行旋转,需要先平移正方形,使其中心位于原点,进行旋转后再平移回去。代码示例如下:
import numpy as np
import matplotlib.pyplot as plt
# 定义旋转角度
angle = np.pi / 4
cos_angle = np.cos(angle)
sin_angle = np.sin(angle)
# 定义正方形的坐标
square = np.array([[0, 1, 1, 0, 0], [0, 0, 1, 1, 0]])
# 计算正方形的中心
center = np.mean(square, axis=1)
# 平移正方形到原点
translated_square = square - center[:, np.newaxis]
# 旋转矩阵
rotation_matrix = np.array([[cos_angle, -sin_angle], [sin_angle, cos_angle]])
# 应用旋转矩阵
rotated_square = rotation_matrix @ translated_square
# 将正方形平移回去
final_square = rotated_square + center[:, np.newaxis]
# 绘制最终的正方形
plt.plot(final_square[0], final_square[1])
plt.axis('equal')
plt.show()
通过这些方法,可以轻松绘制出旋转正方形,并根据需要调整其旋转中心。
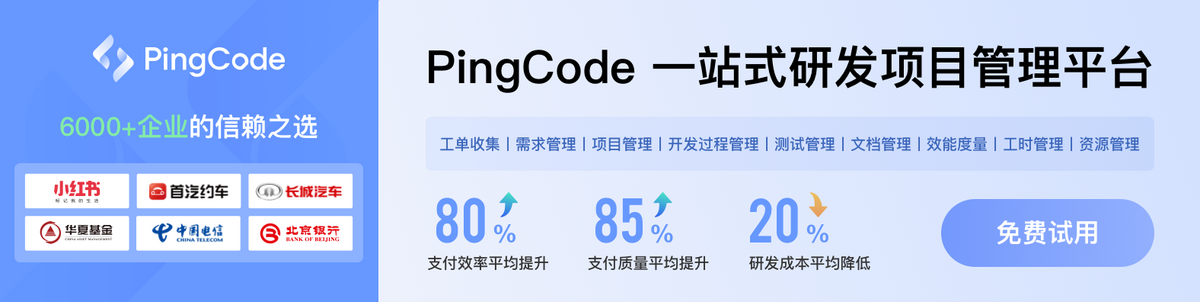