Python统计词汇出现频次的方法包括:使用字典、使用collections.Counter、使用pandas。其中,使用collections.Counter是最简便和高效的方法。下面将详细介绍这几种方法。
一、使用字典
在Python中,字典是一种非常高效的数据结构,可以用于存储键值对。我们可以利用字典来统计词汇的出现频次。具体步骤如下:
- 读取文本数据:可以从文件、字符串等多种来源读取数据。
- 分词:将文本数据分割成单独的词汇。
- 统计频次:使用字典统计每个词汇的出现次数。
示例代码:
def count_word_frequency(text):
# 1. 读取文本数据
words = text.split()
# 2. 初始化一个空字典
frequency = {}
# 3. 统计词汇出现频次
for word in words:
if word in frequency:
frequency[word] += 1
else:
frequency[word] = 1
return frequency
示例文本
text = "this is a test text with some words this is a test"
frequency = count_word_frequency(text)
print(frequency)
二、使用collections.Counter
collections.Counter
是Python标准库中的一个非常有用的类,它用于计数可哈希对象。使用Counter
可以非常方便地统计词汇的出现频次。
示例代码:
from collections import Counter
def count_word_frequency(text):
# 1. 读取文本数据
words = text.split()
# 2. 使用Counter统计词汇出现频次
frequency = Counter(words)
return frequency
示例文本
text = "this is a test text with some words this is a test"
frequency = count_word_frequency(text)
print(frequency)
三、使用pandas
pandas
是一个强大的数据处理和分析库,虽然它主要用于处理结构化数据,但也可以用于统计词汇的出现频次。具体步骤如下:
- 读取文本数据。
- 创建DataFrame:将词汇放入DataFrame中。
- 使用value_counts()方法:统计词汇的出现频次。
示例代码:
import pandas as pd
def count_word_frequency(text):
# 1. 读取文本数据
words = text.split()
# 2. 创建DataFrame
df = pd.DataFrame(words, columns=['word'])
# 3. 使用value_counts()方法统计词汇出现频次
frequency = df['word'].value_counts()
return frequency
示例文本
text = "this is a test text with some words this is a test"
frequency = count_word_frequency(text)
print(frequency)
四、数据预处理
在统计词汇出现频次之前,我们通常需要对数据进行一些预处理,例如去除标点符号、转换为小写字母等。这有助于提高统计的准确性。
示例代码:
import re
from collections import Counter
def preprocess_text(text):
# 1. 去除标点符号
text = re.sub(r'[^\w\s]', '', text)
# 2. 转换为小写字母
text = text.lower()
return text
def count_word_frequency(text):
# 1. 预处理文本数据
text = preprocess_text(text)
# 2. 读取文本数据
words = text.split()
# 3. 使用Counter统计词汇出现频次
frequency = Counter(words)
return frequency
示例文本
text = "This is a test, text with some words! This is a test."
frequency = count_word_frequency(text)
print(frequency)
五、处理大文本数据
当需要处理大文本数据时,可以使用生成器(generator)来逐行读取文件,以节省内存。
示例代码:
import re
from collections import Counter
def preprocess_text(text):
# 1. 去除标点符号
text = re.sub(r'[^\w\s]', '', text)
# 2. 转换为小写字母
text = text.lower()
return text
def count_word_frequency(file_path):
frequency = Counter()
# 1. 逐行读取文件
with open(file_path, 'r', encoding='utf-8') as file:
for line in file:
# 2. 预处理文本数据
line = preprocess_text(line)
# 3. 分词
words = line.split()
# 4. 更新词汇频次
frequency.update(words)
return frequency
示例文件路径
file_path = 'large_text_file.txt'
frequency = count_word_frequency(file_path)
print(frequency)
通过以上几种方法,我们可以高效地统计词汇的出现频次。不同方法有各自的优缺点,选择适合自己的方法可以更好地完成任务。对于小文本数据,使用字典或collections.Counter
都非常方便;而对于大文本数据,使用生成器逐行读取文件可以节省内存,提高效率。
相关问答FAQs:
如何使用Python统计文本中各个词汇的频次?
在Python中,可以使用collections.Counter
类来统计词汇的出现频次。首先,读取文本数据,然后使用split()
方法将文本分割成单词,接着用Counter
来计算每个词的频次。以下是一个简单的示例代码:
from collections import Counter
text = "这是一个示例文本,文本中包含一些重复的词汇。"
words = text.split() # 分割文本
word_counts = Counter(words) # 统计词汇频次
print(word_counts)
在统计词汇频次时,如何处理标点符号和大小写问题?
处理标点符号和大小写是确保统计结果准确的重要步骤。在分割文本之前,可以使用正则表达式删除标点符号,并将所有单词转换为小写。这样可以避免同一词汇因大小写不同而被统计为不同词汇。例如:
import re
from collections import Counter
text = "这是一个示例文本,文本中包含一些重复的词汇。"
text = re.sub(r'[^\w\s]', '', text) # 去除标点符号
words = text.lower().split() # 转为小写并分割
word_counts = Counter(words)
print(word_counts)
有什么Python库可以更方便地进行词频统计?
除了使用collections.Counter
,还有许多第三方库可以简化词频统计的过程。例如,nltk
(自然语言工具包)和pandas
库都提供了强大的文本处理功能。使用nltk
时,可以轻松地进行分词、去停用词等处理,进而进行词频统计。以下是一个使用nltk
的示例:
import nltk
from nltk.tokenize import word_tokenize
from collections import Counter
nltk.download('punkt') # 下载punkt模块
text = "这是一个示例文本,文本中包含一些重复的词汇。"
words = word_tokenize(text) # 使用nltk进行分词
word_counts = Counter(words)
print(word_counts)
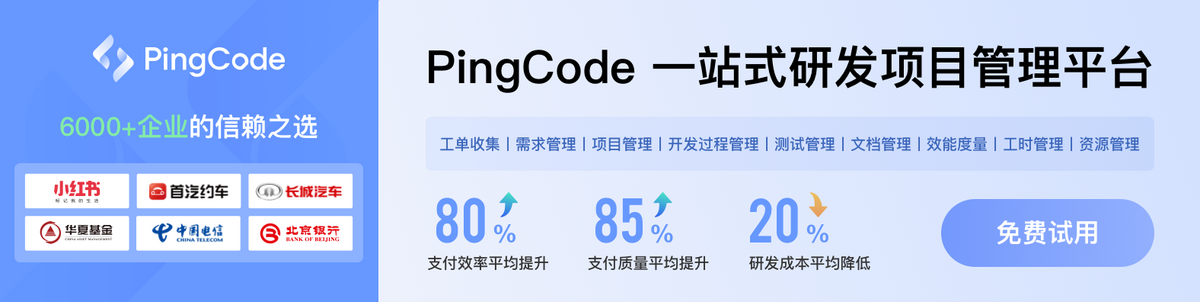