如何用Python实现图像的目标检测
用Python实现图像的目标检测主要通过以下几种方法:使用预训练模型、使用深度学习框架、使用开源库、数据增强。 使用预训练模型可以快速进行目标检测,使用深度学习框架如TensorFlow和PyTorch可以进行自定义的目标检测模型,使用开源库如OpenCV和Detectron2可以简化实现步骤,数据增强可以提高模型的泛化能力。下面将详细介绍其中一种方法,即使用预训练模型进行目标检测。
一、预训练模型
预训练模型是指在大量数据上已经训练好的模型,这些模型可以用来快速进行目标检测。常见的预训练模型包括YOLO(You Only Look Once)、SSD(Single Shot MultiBox Detector)和Faster R-CNN。使用这些模型可以省去训练的时间和资源。
1.1 YOLO(You Only Look Once)
YOLO是一种实时目标检测系统,它能在单次前向传递中同时预测多个边界框和类别概率。它的速度和精度使其在实时应用中非常受欢迎。
import cv2
import numpy as np
加载YOLO模型
net = cv2.dnn.readNet("yolov3.weights", "yolov3.cfg")
layer_names = net.getLayerNames()
output_layers = [layer_names[i[0] - 1] for i in net.getUnconnectedOutLayers()]
加载图像
img = cv2.imread("image.jpg")
height, width, channels = img.shape
预处理图像
blob = cv2.dnn.blobFromImage(img, 0.00392, (416, 416), (0, 0, 0), True, crop=False)
net.setInput(blob)
outs = net.forward(output_layers)
解析输出
class_ids = []
confidences = []
boxes = []
for out in outs:
for detection in out:
scores = detection[5:]
class_id = np.argmax(scores)
confidence = scores[class_id]
if confidence > 0.5:
center_x = int(detection[0] * width)
center_y = int(detection[1] * height)
w = int(detection[2] * width)
h = int(detection[3] * height)
x = int(center_x - w / 2)
y = int(center_y - h / 2)
boxes.append([x, y, w, h])
confidences.append(float(confidence))
class_ids.append(class_id)
非极大值抑制
indexes = cv2.dnn.NMSBoxes(boxes, confidences, 0.5, 0.4)
绘制边界框
for i in range(len(boxes)):
if i in indexes:
x, y, w, h = boxes[i]
label = str(classes[class_ids[i]])
cv2.rectangle(img, (x, y), (x + w, y + h), (0, 255, 0), 2)
cv2.putText(img, label, (x, y + 30), cv2.FONT_HERSHEY_PLAIN, 3, (0, 255, 0), 3)
cv2.imshow("Image", img)
cv2.waitKey(0)
cv2.destroyAllWindows()
二、深度学习框架
使用深度学习框架如TensorFlow和PyTorch可以进行自定义的目标检测模型。以下是使用TensorFlow的一个简单示例。
2.1 使用TensorFlow进行目标检测
TensorFlow提供了一个对象检测API,里面包含了许多预训练模型和工具,可以方便地进行目标检测。
import tensorflow as tf
import numpy as np
import cv2
加载预训练模型
model = tf.saved_model.load("ssd_mobilenet_v2_fpnlite_320x320/saved_model")
加载图像
img = cv2.imread("image.jpg")
input_tensor = tf.convert_to_tensor(img)
input_tensor = input_tensor[tf.newaxis, ...]
检测
detections = model(input_tensor)
解析输出
num_detections = int(detections.pop('num_detections'))
detections = {key: value[0, :num_detections].numpy() for key, value in detections.items()}
detections['num_detections'] = num_detections
绘制边界框
for i in range(num_detections):
box = detections['detection_boxes'][i]
score = detections['detection_scores'][i]
class_id = int(detections['detection_classes'][i])
if score > 0.5:
y1, x1, y2, x2 = box
x1, y1, x2, y2 = int(x1 * img.shape[1]), int(y1 * img.shape[0]), int(x2 * img.shape[1]), int(y2 * img.shape[0])
cv2.rectangle(img, (x1, y1), (x2, y2), (0, 255, 0), 2)
cv2.putText(img, str(class_id), (x1, y1 - 10), cv2.FONT_HERSHEY_SIMPLEX, 0.9, (36,255,12), 2)
cv2.imshow("Image", img)
cv2.waitKey(0)
cv2.destroyAllWindows()
三、开源库
除了预训练模型和深度学习框架,还有许多开源库可以用来进行目标检测。这些库通常封装了复杂的算法,使得目标检测变得更加简单。
3.1 OpenCV
OpenCV是一个开源的计算机视觉库,它包含了许多图像处理和计算机视觉算法。以下是使用OpenCV进行目标检测的示例。
import cv2
加载预训练模型
net = cv2.dnn.readNet("frozen_inference_graph.pb", "ssd_mobilenet_v2_coco.pbtxt")
加载图像
img = cv2.imread("image.jpg")
height, width, channels = img.shape
预处理图像
blob = cv2.dnn.blobFromImage(img, size=(300, 300), swapRB=True, crop=False)
net.setInput(blob)
检测
output = net.forward()
解析输出
for detection in output[0, 0, :, :]:
score = float(detection[2])
if score > 0.5:
left = detection[3] * width
top = detection[4] * height
right = detection[5] * width
bottom = detection[6] * height
cv2.rectangle(img, (int(left), int(top)), (int(right), int(bottom)), (0, 255, 0), thickness=2)
cv2.imshow("Image", img)
cv2.waitKey(0)
cv2.destroyAllWindows()
四、数据增强
数据增强是提高模型泛化能力的重要方法。通过对训练数据进行各种变换,如旋转、缩放、平移等,可以有效地增加数据量,提高模型的鲁棒性。
4.1 图像旋转
旋转是数据增强的一种常见方法,可以通过旋转图像来生成新的训练数据。
import cv2
import numpy as np
加载图像
img = cv2.imread("image.jpg")
旋转
(h, w) = img.shape[:2]
center = (w // 2, h // 2)
M = cv2.getRotationMatrix2D(center, 45, 1.0)
rotated = cv2.warpAffine(img, M, (w, h))
cv2.imshow("Rotated Image", rotated)
cv2.waitKey(0)
cv2.destroyAllWindows()
4.2 图像缩放
缩放是另一种常见的数据增强方法,可以通过缩放图像来生成新的训练数据。
# 加载图像
img = cv2.imread("image.jpg")
缩放
scaled = cv2.resize(img, None, fx=1.5, fy=1.5, interpolation=cv2.INTER_LINEAR)
cv2.imshow("Scaled Image", scaled)
cv2.waitKey(0)
cv2.destroyAllWindows()
通过以上几种方法,可以用Python实现图像的目标检测。无论是使用预训练模型、深度学习框架,还是开源库,都能有效地完成目标检测任务。同时,数据增强技术可以提高模型的泛化能力,使其在实际应用中表现更好。
相关问答FAQs:
如何选择合适的目标检测算法?
在使用Python进行目标检测时,选择合适的算法至关重要。常见的目标检测算法包括YOLO(You Only Look Once)、SSD(Single Shot Multibox Detector)和Faster R-CNN等。选择时可以考虑项目的需求,例如实时性、检测精度以及计算资源的限制。YOLO适合需要快速响应的应用,而Faster R-CNN则适合对精度要求较高的场景。
我需要哪些Python库来实现目标检测?
实现目标检测通常需要一些强大的Python库。OpenCV是一个广泛使用的计算机视觉库,提供了基本的图像处理功能。TensorFlow和PyTorch则是深度学习框架,可以用于构建和训练目标检测模型。此外,Matplotlib可以用于可视化检测结果,方便调试和分析。
如何评估目标检测模型的性能?
评估目标检测模型的性能通常涉及多个指标,如平均精度均值(mAP)、召回率(Recall)和精确率(Precision)。在Python中,可以使用COCO评估工具包来计算这些指标。通过将模型预测的边界框与真实标签进行比较,可以获得更全面的性能评估,帮助优化模型的准确性和鲁棒性。
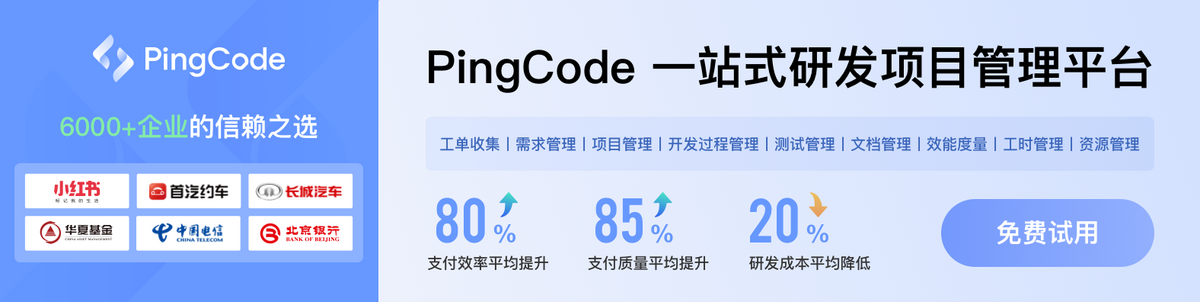