Python游戏开始页面通常包括游戏的标题、开始游戏按钮、帮助按钮、退出按钮等。使用Pygame库、显示游戏标题、添加按钮、响应用户交互。下面将详细讲解如何创建一个简单的Python游戏开始页面。
一、安装并导入Pygame库
首先,我们需要安装Pygame库。可以使用以下命令进行安装:
pip install pygame
安装完成后,在Python脚本中导入Pygame库:
import pygame
import sys
from pygame.locals import *
二、初始化Pygame
在使用Pygame之前,我们需要初始化Pygame库,并设置游戏窗口的标题和尺寸:
pygame.init()
设置游戏窗口尺寸
screen_width = 800
screen_height = 600
screen = pygame.display.set_mode((screen_width, screen_height))
设置游戏窗口标题
pygame.display.set_caption('My Python Game')
三、定义颜色和字体
为了美化游戏开始页面,我们需要定义一些颜色和字体:
# 定义颜色
WHITE = (255, 255, 255)
BLACK = (0, 0, 0)
RED = (255, 0, 0)
GREEN = (0, 255, 0)
BLUE = (0, 0, 255)
定义字体
font = pygame.font.SysFont('Arial', 48)
button_font = pygame.font.SysFont('Arial', 36)
四、创建按钮类
为了方便管理和绘制按钮,我们可以创建一个Button类:
class Button:
def __init__(self, text, x, y, width, height, color, hover_color, font):
self.text = text
self.x = x
self.y = y
self.width = width
self.height = height
self.color = color
self.hover_color = hover_color
self.font = font
self.rect = pygame.Rect(x, y, width, height)
def draw(self, screen):
mouse_pos = pygame.mouse.get_pos()
if self.rect.collidepoint(mouse_pos):
pygame.draw.rect(screen, self.hover_color, self.rect)
else:
pygame.draw.rect(screen, self.color, self.rect)
text_surface = self.font.render(self.text, True, WHITE)
text_rect = text_surface.get_rect(center=self.rect.center)
screen.blit(text_surface, text_rect)
def is_clicked(self, mouse_pos):
return self.rect.collidepoint(mouse_pos)
五、创建游戏开始页面
接下来,我们将创建游戏开始页面,包括标题和按钮:
def game_start_page():
start_button = Button('Start Game', 300, 250, 200, 50, BLUE, GREEN, button_font)
help_button = Button('Help', 300, 350, 200, 50, BLUE, GREEN, button_font)
quit_button = Button('Quit', 300, 450, 200, 50, BLUE, GREEN, button_font)
while True:
screen.fill(WHITE)
# 绘制标题
title_surface = font.render('My Python Game', True, BLACK)
title_rect = title_surface.get_rect(center=(screen_width // 2, 150))
screen.blit(title_surface, title_rect)
# 绘制按钮
start_button.draw(screen)
help_button.draw(screen)
quit_button.draw(screen)
for event in pygame.event.get():
if event.type == QUIT:
pygame.quit()
sys.exit()
if event.type == MOUSEBUTTONDOWN:
if start_button.is_clicked(event.pos):
# 开始游戏逻辑
print("Start Game")
if help_button.is_clicked(event.pos):
# 显示帮助信息
print("Help")
if quit_button.is_clicked(event.pos):
pygame.quit()
sys.exit()
pygame.display.update()
六、运行游戏开始页面
最后,运行游戏开始页面:
if __name__ == '__main__':
game_start_page()
七、总结
通过以上步骤,我们创建了一个简单的Python游戏开始页面。使用Pygame库、显示游戏标题、添加按钮、响应用户交互。可以根据需求进一步美化页面,添加更多功能。
相关问答FAQs:
如何在Python中创建一个游戏开始页面?
在Python中创建游戏开始页面通常涉及使用图形用户界面(GUI)库,如Pygame或Tkinter。你可以设置一个窗口,添加游戏标题、开始按钮和背景图像。使用Pygame时,可以通过绘制文本和图像来实现这个页面,而Tkinter则可以使用内置的按钮和标签来设计界面。
我需要学习哪些基础知识才能制作游戏开始页面?
制作游戏开始页面需要掌握一些基础知识,包括Python编程语言、图形库的使用(如Pygame或Tkinter)、基本的游戏循环概念以及如何处理用户输入。熟悉这些知识可以帮助你更流畅地实现游戏界面和交互功能。
有没有示例代码可以参考?
当然,许多在线资源和教程提供了示例代码。一个简单的Pygame开始页面示例包括初始化Pygame,设置窗口,加载背景图像,渲染文本,并使用事件循环来检测用户点击开始按钮。查阅Pygame官方网站或GitHub上的开源项目可以找到许多有用的示例。
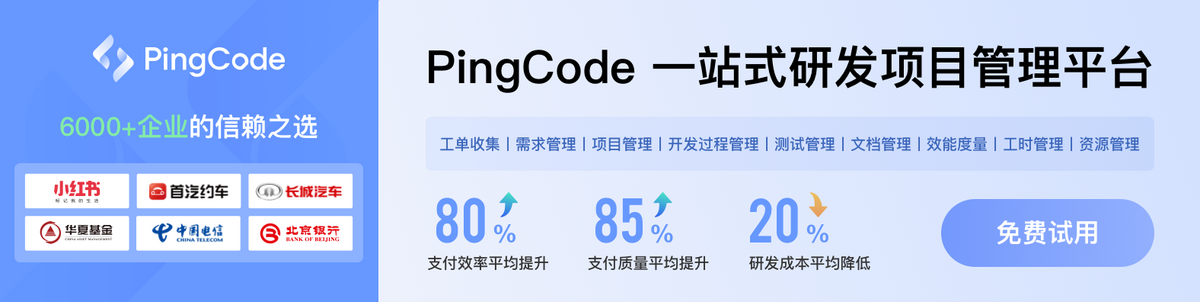